How to use uniapp to implement long press pop-up deletion operation
For more and more apps nowadays, long pressing an element to pop up the operation has become a very common operation method. Today, we will talk about how to implement long-press pop-up deletion in development using uniapp.
- Defining elements in HTML
First, we need to define the elements that need to be operated on in HTML. In this example, we can use v-for
to generate a list, and then each list item should contain the action we need, such as a delete button. The HTML code is as follows:
<template> <div class="list"> <div class="item" v-for="(item, index) in list" :key="index"> <div>{{ item.name }}</div> <button class="delete" @click="deleteItem(index)">删除</button> </div> </div> </template>
where list
is an array, representing the data we need to display, item
is a reference to each item in the array, index
is the index of the current item in the array. Each list item needs to contain a div
element that displays the name, and a button to delete the item.
- Bind events to elements
Next, we need to bind events to the elements we just defined. We need to bind a @longpress
event, which will be triggered when the user long presses the element. At the same time, we also need to record the index of the item that the user long-pressed, so that we can use it in the pop-up delete operation. The HTML code is as follows:
<template> <div class="list"> <div class="item" v-for="(item, index) in list" :key="index" @longpress.native="showMenu(index)"> <div>{{ item.name }}</div> <button class="delete" @click="deleteItem(index)">删除</button> </div> </div> </template>
<script> export default { data() { return { list: [{ name: "item 1" }, { name: "item 2" }, { name: "item 3" }], // 列表数据 longPressIndex: null // 长按的项的下标 }; }, methods: { showMenu(index) { this.longPressIndex = index; // TODO: 显示删除操作的菜单 }, deleteItem(index) { // TODO: 删除列表项 } } }; </script>
As shown above, we record the index of the currently long-pressed item in the showMenu
method, and then we can use it in the pop-up delete operation.
- Pop up the delete operation menu
The next thing we need to achieve is the highlight: pop up the delete operation menu. We can use the uni.showActionSheet
API provided by uniapp to achieve this. We can call it in the showMenu
method to pop up the menu. The code is as follows:
<script> export default { data() { return { list: [{ name: "item 1" }, { name: "item 2" }, { name: "item 3" }], // 列表数据 longPressIndex: null // 长按的项的下标 }; }, methods: { showMenu(index) { this.longPressIndex = index; uni.showActionSheet({ itemList: ["删除"], success: res => { if (res.tapIndex === 0) { this.deleteItem(this.longPressIndex); } } }); }, deleteItem(index) { this.list.splice(index, 1); } } }; </script>
Now, we have successfully implemented the function of long-press pop-up deletion operation. When the user long presses a list item, a menu will pop up. After the user selects delete, the item is deleted from the list.
Summary
Through the above method, we can easily implement the long press pop-up deletion operation in uniapp. However, it should be noted that the long press operation may be different on different platforms, so it needs to be handled for different platforms. Especially in mini programs, when implementing a long press operation, you need to call the mini program API instead of the API provided by uniapp. At the same time, it is also necessary to note that the API used by different versions of uniapp may also be different.
The above is the detailed content of How to use uniapp to implement long press pop-up deletion operation. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
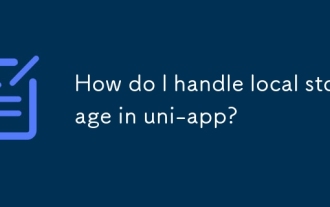
This article details uni-app's local storage APIs (uni.setStorageSync(), uni.getStorageSync(), and their async counterparts), emphasizing best practices like using descriptive keys, limiting data size, and handling JSON parsing. It stresses that lo
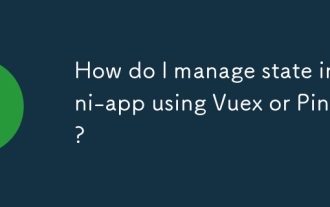
This article compares Vuex and Pinia for state management in uni-app. It details their features, implementation, and best practices, highlighting Pinia's simplicity versus Vuex's structure. The choice depends on project complexity, with Pinia suita

This article details making and securing API requests within uni-app using uni.request or Axios. It covers handling JSON responses, best security practices (HTTPS, authentication, input validation), troubleshooting failures (network issues, CORS, s
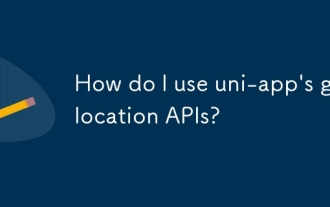
This article details uni-app's geolocation APIs, focusing on uni.getLocation(). It addresses common pitfalls like incorrect coordinate systems (gcj02 vs. wgs84) and permission issues. Improving location accuracy via averaging readings and handling
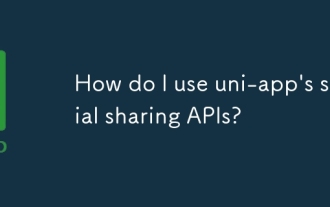
The article details how to integrate social sharing into uni-app projects using uni.share API, covering setup, configuration, and testing across platforms like WeChat and Weibo.

This article explains uni-app's easycom feature, automating component registration. It details configuration, including autoscan and custom component mapping, highlighting benefits like reduced boilerplate, improved speed, and enhanced readability.
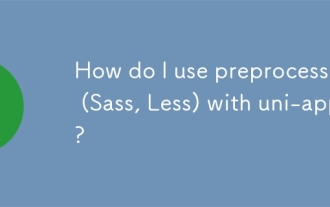
Article discusses using Sass and Less preprocessors in uni-app, detailing setup, benefits, and dual usage. Main focus is on configuration and advantages.[159 characters]
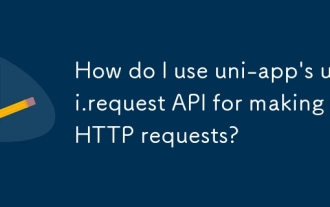
This article details uni.request API in uni-app for making HTTP requests. It covers basic usage, advanced options (methods, headers, data types), robust error handling techniques (fail callbacks, status code checks), and integration with authenticat
