How to remove a key from an array in php
In PHP programs, arrays are one of the commonly used data types, and we often need to operate on arrays. Sometimes, we need to delete a key in an array. This article teaches you how to delete a key in an array in PHP.
To delete a key in an array, you need to use the PHP built-in function unset(). The function of unset() function is to destroy a variable, including a key in the array. Its syntax is as follows:
unset($array[$key]);
Among them, $array is the array of keys to be deleted, and $key is the key to be deleted.
Next, we will introduce in detail how to delete a key in an array in PHP through the following steps.
Step 1: Create an array
First, we need to create an array, which can be created using array literals or array functions.
// 使用数组字面量创建一个数组 $arr = ['a' => 1, 'b' => 2, 'c' => 3]; // 使用数组函数创建一个数组 $arr2 = array('a' => 1, 'b' => 2, 'c' => 3);
Here we use two methods to create two arrays, one using array literals and the other using array functions. Their results are the same.
Step 2: Use the unset() function to delete a key
After we have the array, we can use the unset() function to delete a key in the array.
// 删除数组$arr中的键a unset($arr['a']); // 删除数组$arr2中的键b unset($arr2['b']);
Here we use the unset() function to delete key a in array $arr and key b in array $arr2.
Step 3: Use the print_r() function to output the array
After completing the deletion operation, we can use the print_r() function to output the array to verify whether the operation just performed success.
// 输出数组$arr print_r($arr); // 输出数组$arr2 print_r($arr2);
The output results are as follows:
// 删除键a之后的数组$arr Array ( [b] => 2 [c] => 3 ) // 删除键b之后的数组$arr2 Array ( [a] => 1 [c] => 3 )
As can be seen from the output results, the key we specified has been successfully deleted from the array.
Summary
Using the unset() function can very conveniently delete a key in a PHP array. It should be noted that when we delete a key, PHP will reconstruct the array, and the index will start counting from 0 according to natural numbers.
Hope this article will be helpful to PHP programmers.
The above is the detailed content of How to remove a key from an array in php. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
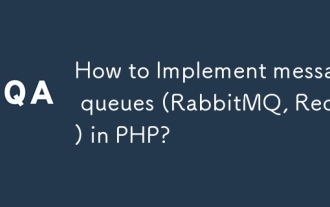
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
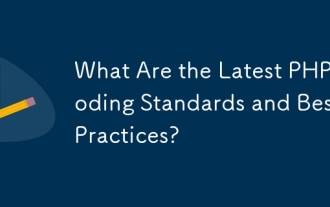
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
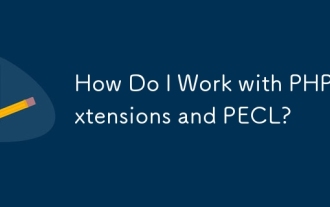
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
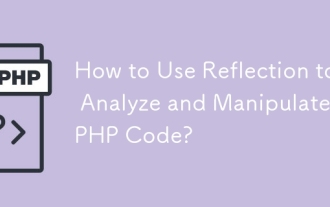
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
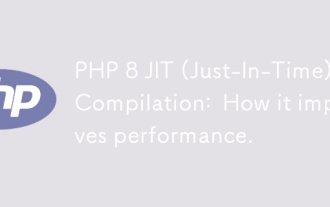
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
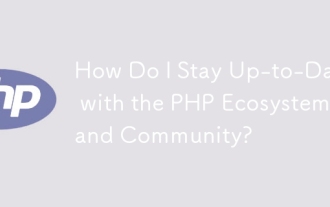
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
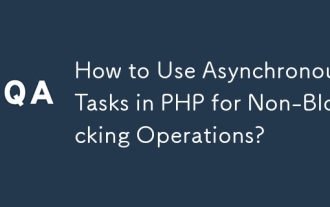
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
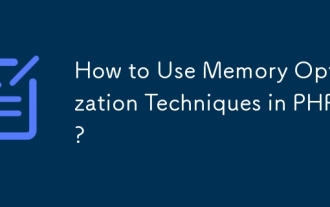
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v
