How to determine whether an object array is empty in php
PHP is a scripting language widely used in web programming, especially good at handling dynamic content and interactive applications. There are many variable types in PHP, of which objects and arrays are two very important types. During the development process, we may use object arrays, and judging whether an object array is empty is a basic operation.
The object array in PHP is an array composed of multiple objects, each object has its own properties and methods. Below we will discuss how to determine whether an object array is empty from two different perspectives.
1. Determine whether the object array has elements
In PHP, you can use the count() function to determine the number of elements in an array. This function works with arrays of all data types, including arrays of objects. Therefore, you can use the following method to determine whether an object array is empty:
<?php $object_array = array(); // 创建一个空的对象数组 if (count($object_array) == 0) { echo "这个对象数组没有元素"; } else { echo "这个对象数组有元素"; } ?>
In the above code, we created an empty object array named $object_array and used the count() function to determine it. . If there are no elements in the array, output "This object array has no elements", otherwise output "This object array has elements".
2. Determine whether the object in the object array is empty
The above method can only determine whether the object array has elements, but cannot determine whether the object's properties and methods are empty. If we want to check whether each object in the object array is empty, we can use the following method:
<?php class example { public $member; public function do_something() { // 做一些事情 } } $object_array = array(new example(), new example()); // 创建一个有两个对象的数组 $empty_flag = true; foreach ($object_array as $object) { if (!empty(get_object_vars($object)) || !empty(get_class_methods(get_class($object)))) { $empty_flag = false; break; } } if ($empty_flag) { echo "这个对象数组中所有的对象都是空的"; } else { echo "这个对象数组中至少有一个对象不是空的"; } ?>
The above code first defines a class named example and creates a class with two example objects. Object array $object_array. Next, we use a foreach loop to iterate through each object in the array and use the get_object_vars() and get_class_methods() functions to get the object properties and methods. If for any object, its properties and methods are empty, $empty_flag is true. Finally, we output the corresponding results based on the value of $empty_flag.
Summary
In PHP, determining whether an object array is empty is a basic operation. We can use PHP's built-in functions to check the number of elements in the object array, and we can also determine whether the object array is empty by checking the properties and methods of each object. In actual development, considering the readability and maintainability of the code, we should choose an appropriate way to determine whether the object array is empty.
The above is the detailed content of How to determine whether an object array is empty in php. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


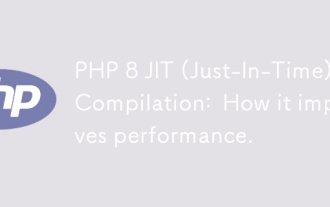
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
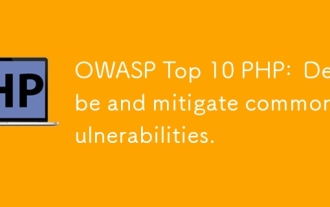
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
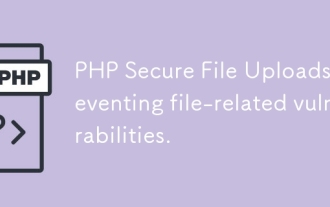
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
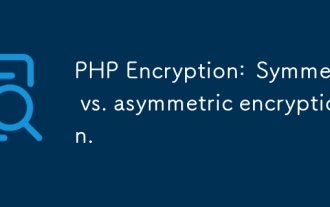
The article discusses symmetric and asymmetric encryption in PHP, comparing their suitability, performance, and security differences. Symmetric encryption is faster and suited for bulk data, while asymmetric is used for secure key exchange.

The article discusses implementing robust authentication and authorization in PHP to prevent unauthorized access, detailing best practices and recommending security-enhancing tools.
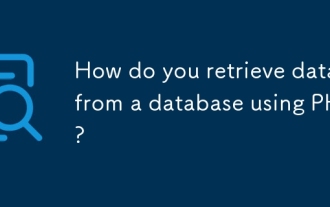
Article discusses retrieving data from databases using PHP, covering steps, security measures, optimization techniques, and common errors with solutions.Character count: 159
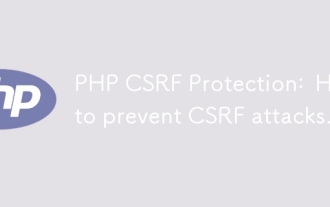
The article discusses strategies to prevent CSRF attacks in PHP, including using CSRF tokens, Same-Site cookies, and proper session management.

Prepared statements in PHP enhance database security and efficiency by preventing SQL injection and improving query performance through compilation and reuse.Character count: 159
