How to get the height of an element in uniapp
In the process of developing projects using uniapp, we often need to obtain the height of elements in order to perform corresponding operations and layout, such as dynamically calculating the height of list items, setting the minimum height of components, etc. So, how to get the height of the element in uniapp?
Method 1: uni.createComponent()
In uniapp, use uni.createComponent() to dynamically create custom components. In a custom component, we can use the uni.createSelectorQuery() method in the life cycle function of the custom component to obtain the information of the element node, including the height, width, etc. of the element.
Take getting the height of a div element as an example:
In the created life cycle function of the custom component, you can use the uni.createSelectorQuery() method to get the element information. The specific code is as follows:
<template> <div class="component"> <div class="content" ref="content"> 我是一个自定义组件 </div> </div> </template> <script> export default { created () { // 获取元素的信息 uni.createSelectorQuery().in(this).select('.content').boundingClientRect((rect) => { console.log('元素高度为:' + rect.height) }).exec() } } </script> <style> .component { width: 100%; height: 100%; } .content { width: 100px; height: 100px; background-color: red; } </style>
In the above code, ref is used to obtain the reference of the div element, and then the uni.createSelectorQuery() method is used in the created life cycle function to query the element information. Among them, select('.content') means to query the element whose class is content, and the boundingClientRect() method means to query the size information of the element. The rect returned in the callback function is an object containing the position, size and other information of the element.
Method 2: uni.pageScrollTo()
In some cases, we need to get the height of an element on the page, which can be achieved using the uni.pageScrollTo() method. The specific code is as follows:
<template> <div class="component"> <div class="content" ref="content"> 我是一个自定义组件 </div> </div> </template> <script> export default { mounted () { // 获取页面中元素的高度 uni.pageScrollTo({ selector: '.content', success: (res) => { console.log('元素高度为:' + res[0].top) } }) } } </script> <style> .component { width: 100%; height: 100%; } .content { width: 100px; height: 100px; background-color: red; } </style>
In the above code, the mounted life cycle function is used, and the uni.pageScrollTo() method is used after the page rendering is completed. selector: '.content' means querying elements with class content. The res in the success callback function can obtain the element information, where res[0].top means the distance between the element and the top of the page.
Summary:
The above two methods, the former is suitable for getting the height of elements in custom components, and the latter is suitable for getting the height of an element on the page. Both have their own advantages and disadvantages, and you can choose according to specific scenarios. No matter which method is used, you need to pay attention to adding the callback function of the size information within the corresponding life cycle function or method in order to obtain the height and other information of the element.
The above is the detailed content of How to get the height of an element in uniapp. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
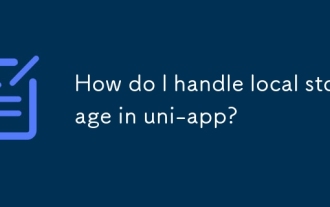
This article details uni-app's local storage APIs (uni.setStorageSync(), uni.getStorageSync(), and their async counterparts), emphasizing best practices like using descriptive keys, limiting data size, and handling JSON parsing. It stresses that lo
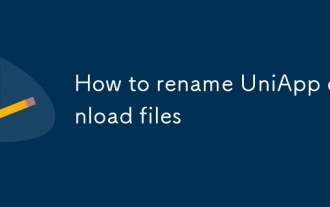
This article details workarounds for renaming downloaded files in UniApp, lacking direct API support. Android/iOS require native plugins for post-download renaming, while H5 solutions are limited to suggesting filenames. The process involves tempor
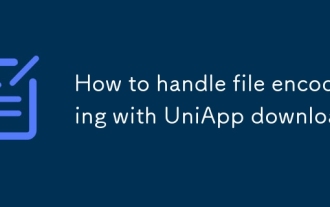
This article addresses file encoding issues in UniApp downloads. It emphasizes the importance of server-side Content-Type headers and using JavaScript's TextDecoder for client-side decoding based on these headers. Solutions for common encoding prob
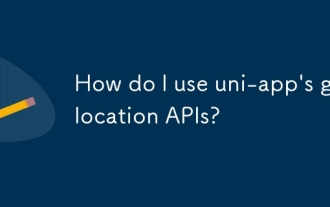
This article details uni-app's geolocation APIs, focusing on uni.getLocation(). It addresses common pitfalls like incorrect coordinate systems (gcj02 vs. wgs84) and permission issues. Improving location accuracy via averaging readings and handling
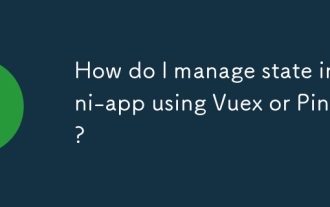
This article compares Vuex and Pinia for state management in uni-app. It details their features, implementation, and best practices, highlighting Pinia's simplicity versus Vuex's structure. The choice depends on project complexity, with Pinia suita

This article details making and securing API requests within uni-app using uni.request or Axios. It covers handling JSON responses, best security practices (HTTPS, authentication, input validation), troubleshooting failures (network issues, CORS, s
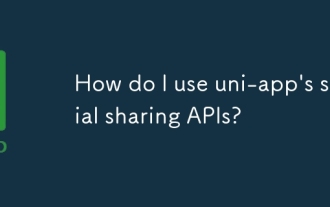
The article details how to integrate social sharing into uni-app projects using uni.share API, covering setup, configuration, and testing across platforms like WeChat and Weibo.

This article explains uni-app's easycom feature, automating component registration. It details configuration, including autoscan and custom component mapping, highlighting benefits like reduced boilerplate, improved speed, and enhanced readability.
