How to use Date to implement stopwatch in javascript
With the development of modern society, the role of timers is becoming more and more important. Whether it is timing in daily life, or timing in sports competitions and computer competitions, it needs to be implemented as a stopwatch. Today we will introduce how to use the Date object in JavaScript to implement a stopwatch.
First, let’s take a look at JavaScript’s Date object. The Date object is a very commonly used object in JavaScript, which is used to represent time and date. We can use the new Date() statement to create a new Date object. By default, using the new Date() statement returns the current time and date.
The following is an example showing how to use a Date object to get the current time:
let now = new Date(); console.log(now);
The output result is the current time and date, for example:
Thu Oct 07 2021 12:08:20 GMT+0800 (中国标准时间)
After getting the current time, we You can use it to implement a stopwatch. The stopwatch needs to use a timer, and the timing function is implemented by setting the setInterval() function. The following is an example showing how to use the setInterval() function to implement a simple timer:
let count = 0; let intervalId = setInterval(function() { count++; console.log(count); }, 1000);
In this example, we use the count variable to record the number of seconds that have passed. After each second, the Count is incremented by 1 and output to the console. We used the setInterval() function and assigned it to the intervalId variable. The setInterval() function will execute the passed function at regular intervals and return a unique identifier for subsequent operations.
Next, we can add start, stop and reset functions to the stopwatch. Below is a complete implementation that includes the 3 buttons Start, Stop and Reset and can be timed correctly.
<html> <head> <title>JavaScript秒表</title> </head> <body> <h1>JavaScript秒表</h1> <div id="clock">00:00:00</div> <button id="startBtn">开始</button> <button id="stopBtn">停止</button> <button id="resetBtn">重置</button> </body> <script> let intervalId = null; let count = 0; let clock = document.getElementById('clock'); let startBtn = document.getElementById('startBtn'); let stopBtn = document.getElementById('stopBtn'); let resetBtn = document.getElementById('resetBtn'); function startClock(){ intervalId = setInterval(function() { count++; let hours = Math.floor(count / 3600); let minutes = Math.floor((count - hours * 3600) / 60); let seconds = count - hours * 3600 - minutes * 60; clock.innerHTML = hours.toString().padStart(2, '0') + ':' + minutes.toString().padStart(2, '0') + ':' + seconds.toString().padStart(2, '0'); }, 1000); } function stopClock(){ clearInterval(intervalId); } function resetClock(){ clearInterval(intervalId); count = 0; clock.innerHTML = '00:00:00'; } startBtn.addEventListener('click', startClock); stopBtn.addEventListener('click', stopClock); resetBtn.addEventListener('click', resetClock); </script> </html>
In this example, we first created three buttons and added start, stop and reset functions to them. We use the getElementById() function to obtain the HTML elements on the page and assign them to the corresponding JavaScript variables.
In the startClock() function, we use the setInterval() function to time, and output the timing results to the page in the format of hh:mm:ss. We use the Math.floor() function to round, and the padStart() function to format the number into a two-digit string for easy output to the page. In the stopClock() and resetClock() functions, we use the clearInterval() function to stop the timer and reset the count and clock values respectively.
If you want to use JavaScript to implement a stopwatch, then this example should be able to provide you with some help. You can modify and extend the code as needed to achieve richer and more complex timing functions. Okay, that’s it for now. I hope this article will be helpful to you.
The above is the detailed content of How to use Date to implement stopwatch in javascript. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


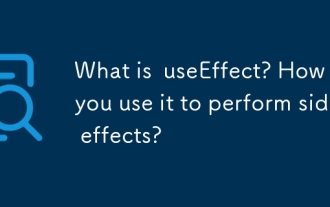
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.
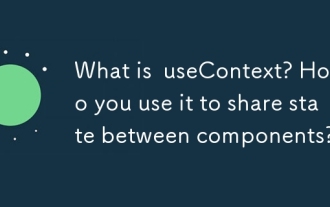
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
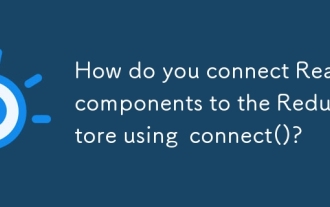
Article discusses connecting React components to Redux store using connect(), explaining mapStateToProps, mapDispatchToProps, and performance impacts.
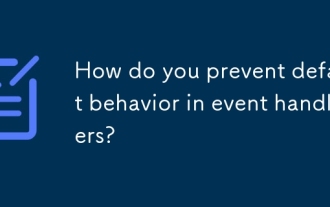
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
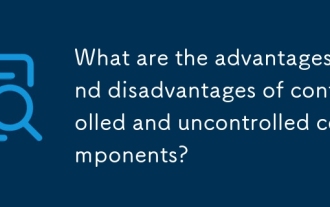
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.
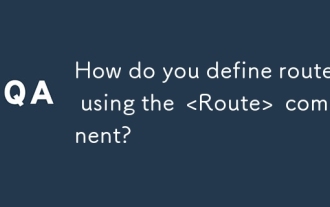
The article discusses defining routes in React Router using the <Route> component, covering props like path, component, render, children, exact, and nested routing.

React combines JSX and HTML to improve user experience. 1) JSX embeds HTML to make development more intuitive. 2) The virtual DOM mechanism optimizes performance and reduces DOM operations. 3) Component-based management UI to improve maintainability. 4) State management and event processing enhance interactivity.
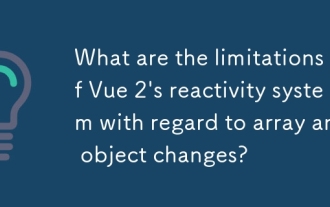
Vue 2's reactivity system struggles with direct array index setting, length modification, and object property addition/deletion. Developers can use Vue's mutation methods and Vue.set() to ensure reactivity.
