What features does reflection in Java provide?
1. Description
Determine the class to which any object belongs at runtime
Construct an object of any class at runtime
In Determine the member variables and methods of any class at runtime
Get generic information at runtime
Call the member variables and methods of any object at runtime
Process annotations at runtime
Generate dynamic proxy
2, instance
@Test public void test1() throws Exception { Class<Person> clazz = Person.class; //1.通过反射,创建Person类对象 Constructor<Person> cons = clazz.getConstructor(String.class, int.class); Person person = cons.newInstance("Tom", 12); System.out.println(person);//Person{name='Tom', age=12} //2.通过反射,调用对象指定的属性、方法 //调用属性 Field age = clazz.getDeclaredField("age"); age.setAccessible(true); age.set(person, 10); System.out.println(person.toString());//Person{name='Tom', age=10} //调用方法 Method show = clazz.getDeclaredMethod("show"); show.invoke(person);//my name is Tom and age is 10 System.out.println("==================================="); //通过反射,可以调用Person类的私有结构的。比如:私有的构造器、方法、属性 //调用私有的构造器 Constructor<Person> cons1 = clazz.getDeclaredConstructor(String.class); cons1.setAccessible(true); Person p1 = cons1.newInstance("Bruce"); System.out.println(p1);//Person{name='Bruce', age=0} //调用私有的属性 Field name = clazz.getDeclaredField("name"); name.setAccessible(true); name.set(p1, "Jarry"); System.out.println(p1); //调用私有的方法 Method nation = clazz.getDeclaredMethod("nation", String.class); nation.setAccessible(true); Object nation1 = (String) nation.invoke(p1, "China");//相当于String nation = p1.showNation("China") System.out.println(nation1);//I come from China }
The above is the detailed content of What features does reflection in Java provide?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


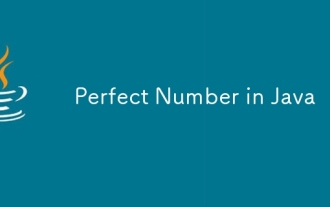
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
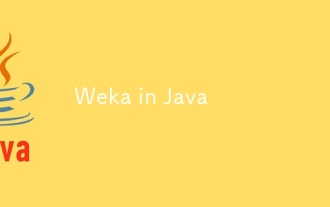
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
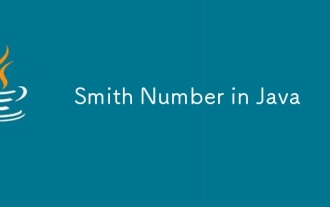
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
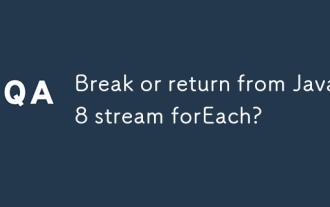
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
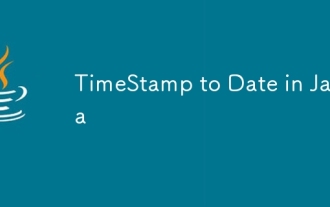
Guide to TimeStamp to Date in Java. Here we also discuss the introduction and how to convert timestamp to date in java along with examples.
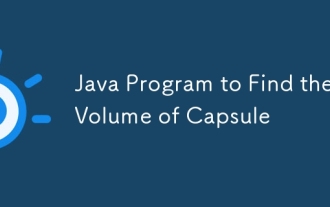
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
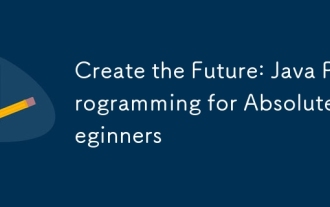
Java is a popular programming language that can be learned by both beginners and experienced developers. This tutorial starts with basic concepts and progresses through advanced topics. After installing the Java Development Kit, you can practice programming by creating a simple "Hello, World!" program. After you understand the code, use the command prompt to compile and run the program, and "Hello, World!" will be output on the console. Learning Java starts your programming journey, and as your mastery deepens, you can create more complex applications.
