


Detailed explanation and practical guide of Python Socket programming
In today's Internet, the Socket protocol is one of the most important foundations. This article covers all areas of working with Socket programming in Python.
Why use Sockets
Sockets are the various communication protocols that make up today's networks. These protocols enable the transmission of information between two different programs or devices. become possible. For example, when we open a browser, we as clients create a connection to the server to transfer information.
Before delving into this communication principle, let us first clarify what Sockets are.
What are Sockets
Generally speaking, Sockets are internal application protocols built for sending and receiving data. A single network will have two Sockets, one for each communicating device or program, these Sockets are a combination of IP address and port. Depending on the port number used, a single device can have "n" number of Sockets, and different ports can be used for different types of protocols.
The following figure shows some common port numbers and related protocol information:
Protocol |
Port number |
##Python library |
Application |
HTTP | ##80|||
|
|
||
119 |
##nttplib |
News Transfer |
|
SMTP |
25 | ##smtplib | Send email|
|
|
|
| POP3
##110 |
##poplib |
Receive email |
|
70
|
gopherlib |
Document transfer |
Now that we have understood the concept of Sockets, let us take a look at Python's Socket module
How to implement Socket programming in Python
To implement Socket programming in Python, you need Import the socket module.
Some important methods of this module are as follows:
##Methods |
Description |
|
|
|
|
|
|
|
|
|
The above is the detailed content of Detailed explanation and practical guide of Python Socket programming. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
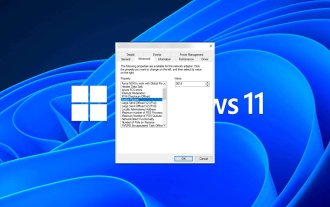
If you're suddenly experiencing a slow internet connection on Windows 11 and you've tried every trick in the book, it might have nothing to do with your network and everything to do with your maximum transmission unit (MTU). Problems may occur if your system sends or receives data with the wrong MTU size. In this post, we will learn how to change MTU size on Windows 11 for smooth and uninterrupted internet connection. What is the default MTU size in Windows 11? The default MTU size in Windows 11 is 1500, which is the maximum allowed. MTU stands for maximum transmission unit. This is the maximum packet size that can be sent or received on the network. every support network
![WLAN expansion module has stopped [fix]](https://img.php.cn/upload/article/000/465/014/170832352052603.gif?x-oss-process=image/resize,m_fill,h_207,w_330)
If there is a problem with the WLAN expansion module on your Windows computer, it may cause you to be disconnected from the Internet. This situation is often frustrating, but fortunately, this article provides some simple suggestions that can help you solve this problem and get your wireless connection working properly again. Fix WLAN Extensibility Module Has Stopped If the WLAN Extensibility Module has stopped working on your Windows computer, follow these suggestions to fix it: Run the Network and Internet Troubleshooter to disable and re-enable wireless network connections Restart the WLAN Autoconfiguration Service Modify Power Options Modify Advanced Power Settings Reinstall Network Adapter Driver Run Some Network Commands Now, let’s look at it in detail
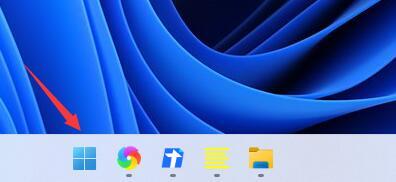
We need to use the correct DNS when connecting to the Internet to access the Internet. In the same way, if we use the wrong dns settings, it will prompt a dns server error. At this time, we can try to solve the problem by selecting to automatically obtain dns in the network settings. Let’s take a look at the specific solutions. How to solve win11 network dns server error. Method 1: Reset DNS 1. First, click Start in the taskbar to enter, find and click the "Settings" icon button. 2. Then click the "Network & Internet" option command in the left column. 3. Then find the "Ethernet" option on the right and click to enter. 4. After that, click "Edit" in the DNS server assignment, and finally set DNS to "Automatic (D
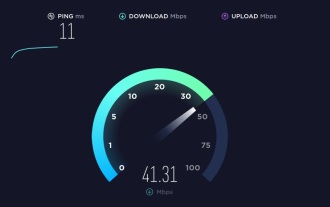
What is the "Network error download failed" issue? Before we delve into the solutions, let’s first understand what the “Network Error Download Failed” issue means. This error usually occurs when the network connection is interrupted during downloading. It can happen due to various reasons such as weak internet connection, network congestion or server issues. When this error occurs, the download will stop and an error message will be displayed. How to fix failed download with network error? Facing “Network Error Download Failed” can become a hindrance while accessing or downloading necessary files. Whether you are using browsers like Chrome or platforms like Google Drive and Google Photos, this error will pop up causing inconvenience. Below are points to help you navigate and resolve this issue
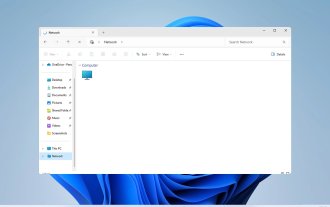
If WDMyCloud is not showing up on the network in Windows 11, this can be a big problem, especially if you store backups or other important files in it. This can be a big problem for users who frequently need to access network storage, so in today's guide, we'll show you how to fix this problem permanently. Why doesn't WDMyCloud show up on Windows 11 network? Your MyCloud device, network adapter, or internet connection is not configured correctly. The SMB function is not installed on the computer. A temporary glitch in Winsock can sometimes cause this problem. What should I do if my cloud doesn't show up on the network? Before we start fixing the problem, you can perform some preliminary checks:
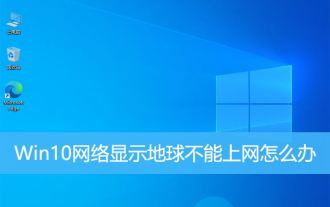
This article will introduce the solution to the problem that the globe symbol is displayed on the Win10 system network but cannot access the Internet. The article will provide detailed steps to help readers solve the problem of Win10 network showing that the earth cannot access the Internet. Method 1: Restart directly. First check whether the network cable is not plugged in properly and whether the broadband is in arrears. The router or optical modem may be stuck. In this case, you need to restart the router or optical modem. If there are no important things being done on the computer, you can restart the computer directly. Most minor problems can be quickly solved by restarting the computer. If it is determined that the broadband is not in arrears and the network is normal, that is another matter. Method 2: 1. Press the [Win] key, or click [Start Menu] in the lower left corner. In the menu item that opens, click the gear icon above the power button. This is [Settings].
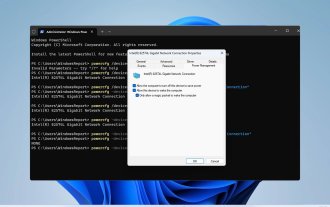
Wake on LAN is a network feature on Windows 11 that allows you to remotely wake your computer from hibernation or sleep mode. While casual users don't use it often, this feature is useful for network administrators and power users using wired networks, and today we'll show you how to set it up. How do I know if my computer supports Wake on LAN? To use this feature, your computer needs the following: The PC needs to be connected to an ATX power supply so that you can wake it from sleep mode remotely. Access control lists need to be created and added to all routers in the network. The network card needs to support the wake-up-on-LAN function. For this feature to work, both computers need to be on the same network. Although most Ethernet adapters use
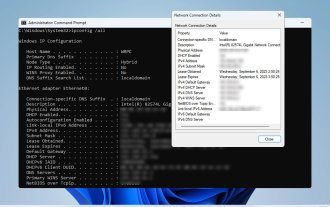
In order to make sure your network connection is working properly or to fix the problem, sometimes you need to check the network connection details on Windows 11. By doing this, you can view a variety of information including your IP address, MAC address, link speed, driver version, and more, and in this guide, we'll show you how to do that. How to find network connection details on Windows 11? 1. Use the "Settings" app and press the + key to open Windows Settings. WindowsI Next, navigate to Network & Internet in the left pane and select your network type. In our case, this is Ethernet. If you are using a wireless network, select a Wi-Fi network instead. At the bottom of the screen you should see
