Java 9's HTTP2 protocol support and non-blocking HTTP API analysis
1. Introduction to HTTP/2
HTTP/2 aims to alleviate the pain caused by maintaining the complex infrastructure of HTTP/1.1 and has good performance. Although HTTP/2 is still backwards compatible with HTTP/1.1, it is no longer a text-based protocol.
HTTP/2 multiplexing enables a single connection to handle multiple bidirectional streams, allowing clients to download multiple resources simultaneously over a single connection.
HTTP 1.x protocol is text-based, so the messages are very lengthy. Sometimes, the same set of HTTP headers are exchanged over and over again. HTTP/2 maintains HTTP Headers across requests, eliminating repeated exchange of data and greatly reducing the bandwidth required for data interaction.
HTTP/2 Data Push
You may think that HTTP/2’s server-side data push is some kind of continuation or upgrade to WebSockets, but this is not the case. While WebSockets are a method of full-duplex communication between a client and a server so that the server sends data to the client after a TCP connection is established, HTTP/2 offers a different solution.
HTTP/2 push is to actively send resources to the client without initiating resource requests from the client's perspective. This means that the server may know from a request what other resources the website will need further and can send them all together (in advance) long before the client requests them again.
Java HTTP client that currently supports HTTP/2
Jetty
Netty
OkHttp
- ##Vert.x
- Firefly
jdk.incubator.httpclient
module com.springui.echo.client { requires jdk.incubator.httpclient; }
HttpClient client = HttpClient .newBuilder() .version(Version.HTTP_2) //支持HTTP2 .build();
HttpResponse<String> response = client.send( HttpRequest .newBuilder(TEST_URI) //请求地址 .POST(BodyProcessor.fromString("Hello world")) //POST报文数据 .build(), BodyHandler.asString() //请求响应数据处理,接收字符串 );
List<CompletableFuture<String>> responseFutures = IntStream.of(1,2,3,4,5,6,7,8,9,10) //10个整数形成IntStream,Java 8的语法 .mapToObj(String::valueOf) //10个整数转换成字符串,Java 8的语法 .map(message -> client.sendAsync( //将10个整数字符串作为内容,发送10个异步请求 HttpRequest.newBuilder(TEST_URI) .POST(HttpRequest.BodyProcessor.fromString(message)) .build(), HttpResponse.BodyHandler.asString() ).thenApply(HttpResponse::body) //以CompletableFuture<HttpResponse.body()>作为流处理的返回值 ) .collect(Collectors.toList()); //将Stream转成List
responseFutures.stream().forEach(future -> { LOGGER.info("Async response: " + future.getNow(null)); });
Map<HttpRequest,CompletableFuture<HttpResponse<String>>> responses = client.sendAsync( //注意这里只发送一次请求 HttpRequest.newBuilder(TEST_URI) .POST(HttpRequest.BodyProcessor.fromString(TEST_MESSAGE)) .build(), HttpResponse.MultiProcessor.asMap( //多个资源的响应结果 request -> Optional.of(HttpResponse.BodyHandler.asString()) ) ).join(); responses.forEach((request, responseFuture) -> { LOGGER.info("Async response: " + responseFuture.getNow(null)); });
The above is the detailed content of Java 9's HTTP2 protocol support and non-blocking HTTP API analysis. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
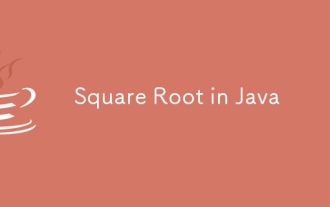
Guide to Square Root in Java. Here we discuss how Square Root works in Java with example and its code implementation respectively.
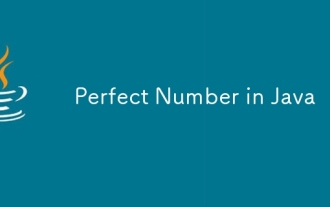
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
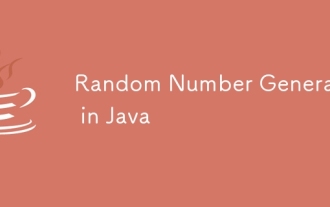
Guide to Random Number Generator in Java. Here we discuss Functions in Java with examples and two different Generators with ther examples.
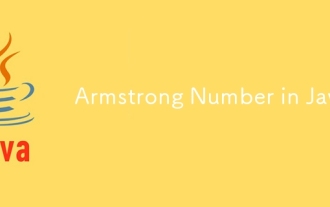
Guide to the Armstrong Number in Java. Here we discuss an introduction to Armstrong's number in java along with some of the code.
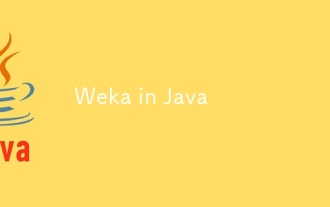
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
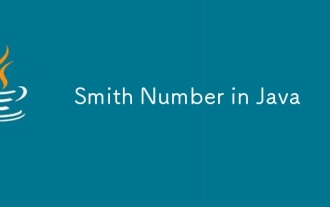
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
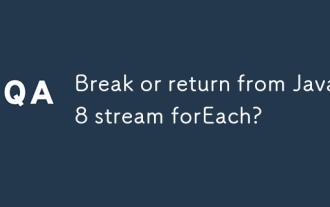
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
