How to use Python's OS module and examples
Python's os module is one of the standard libraries used to interact with the operating system. It provides many useful functions and variables for working with files and directories. The following are the usage of some common os module functions:
1. Get the current working directory:
import os cwd = os.getcwd() print(cwd)
2. Switch the current working directory:
import os os.chdir('/path/to/new/directory')
3. List the directory All files and subdirectories in:
import os files = os.listdir('/path/to/directory') print(files)
4. Check whether the given path is a directory:
import os path = '/path/to/directory' if os.path.isdir(path): print("It's a directory") else: print("It's not a directory")
5. Check whether the given path is a file:
import os path = '/path/to/file' if os.path.isfile(path): print("It's a file") else: print("It's not a file")
6. Get the size of the file in bytes:
import os path = '/path/to/file' size = os.path.getsize(path) print(size)
7. Check if the given path exists:
import os path = '/path/to/file_or_directory' if os.path.exists(path): print("It exists") else: print("It doesn't exist")
8. Create a new directory:
import os path = '/path/to/new/directory' os.mkdir(path)
9. Recursively create new directories (if the directory does not exist):
import os path = '/path/to/new/directory' os.makedirs(path, exist_ok=True)
10. Delete files or empty directories:
import os path = '/path/to/file_or_directory' os.remove(path)
11. Recursively delete directories and their contents:
import os path = '/path/to/directory' os.system('rm -rf ' + path)
Some other convenient uses:
12.os.path.splitext() method is to split a path into two parts: file name and extension. It uses the last "." in the file name as a delimiter to separate the file name and extension. For example, if the file path is "/path/to/file.txt", the os.path.splitext() method returns a tuple ("/path/to/file", ".txt").
It should be noted that if there is no "." in the file name, the returned extension is an empty string. If the file name begins with ".", it is considered a file without extension and the os.path.splitext() method will return (file path, '').
The following is an example:
import os path = '/path/to/file.txt' file_name, ext = os.path.splitext(path) print('文件名为:', file_name) print('扩展名为:', ext)
The output result is:
The file name is: /path/to/file
The extension is: .txt
13. Set file permissions:
import os os.chmod('/path/to/file', 0o777) # 设置读、写、执行权限
os.chmod() method can be used to modify the access permissions of files or directories. It accepts two parameters: file path and new permission mode. Permission mode can be represented by an octal number, with each bit representing a different permission.
Here are some examples of permission modes:
0o400: Read-only permission
0o200: Write permission
0o100: Execution permission
0o700: All permissions
14. Get the number of CPUs:
import os cpu_count = os.cpu_count() print('CPU数量为:', cpu_count)
It should be noted that the number of CPUs returned by os.cpu_count() is the number of physical CPU cores and does not include the virtual cores of hyper-threading technology. In systems with Hyper-Threading Technology, each physical CPU core is divided into two virtual cores, so the os.cpu_count() method may return a number greater than the actual number of CPU cores.
In addition, the os.cpu_count() method may have different implementations on different operating systems. On some operating systems, it may only return the number of logical CPU cores, not the number of physical CPU cores. Therefore, when using this method, it is best to consult the relevant documentation for more information.
15. Start a new process:
import os os.system('notepad.exe')
The os.system() method can execute a command on the operating system and return the command's exit status code. Its parameter is a string type command, which can be any valid system command.
The following is an example that demonstrates how to use the os.system() method to execute a simple command:
import os os.system('echo "Hello, World!"')
The above code will output the Hello, World! string and return the command's Exit status code (usually 0 for success).
It should be noted that the os.system() method will block the current process until the command execution is completed. If you want to execute the command without blocking the current process, you can consider using other methods in the subprocess module, such as subprocess.Popen().
The following is another example that demonstrates how to use the os.system() method to execute a complex command, such as using wget to download a file on a Linux system:
import os url = 'https://example.com/file.zip' output_dir = '/path/to/output' command = f'wget {url} -P {output_dir}' os.system(command)
The above code will Download the file specified by the url parameter to the directory specified by the output_dir parameter, and return the command's exit status code.
16.os.environ: This is a dictionary containing the current environment variables. You can use os.environ[key] to get the value of a specific environment variable.
17.os.exec*(): These methods allow Python programs to execute other programs in the current process, replacing the current process. For example, the os.execv() method can execute a program using a specified argument list, replacing the current process.
18.os.fork(): This method can create a child process on the Unix or Linux operating system for parallel execution of the program. The child process will copy all the memory contents of the parent process, including code, data, stack, etc., so the program can continue to execute based on the parent process.
19.os.kill(): This method is used to send a signal to the specified process. You can use the os.kill(pid, signal) method to send a specified signal to a specified process. Commonly used signals include SIGINT (interrupt signal), SIGTERM (termination signal) and SIGKILL (forced termination signal), etc.
20.os.pipe(): This method can create a pipe for communication between processes. The os.pipe() method will return two file descriptors, one for reading pipe data and the other for writing pipe data.
21.os.wait(): This method can wait for the end of the child process and then return the status code of the child process. You can use the os.waitpid(pid, options) method to wait for the specified process to end and return the status code of the process.
22.os模块可以用来操作文件路径。例如,os.path.join(path, *paths)可以将多个路径拼接成一个完整路径,os.path.abspath(path)可以将相对路径转换为绝对路径,os.path.split(path)可以将路径分割成目录和文件名。
23.遍历目录树
import os def list_files(path): for root, dirs, files inos.walk(path): for file in files: print(os.path.join(root, file)) list_files('.')
这段代码可以遍历当前工作目录及其子目录下的所有文件,并打印出它们的完整路径。
os.walk()是os模块中一个非常有用的函数,用于遍历指定目录及其子目录下的所有文件和目录。它返回一个三元组(root, dirs, files),其中root是当前目录的路径,dirs是当前目录下的子目录列表,files是当前目录下的文件列表。下面是一个os.walk()的详细解释和示例:
for root, dirs, files in os.walk(top, topdown=True, onerror=None, followlinks=False): # Do something with root, dirs, and files
top是指定的目录路径,可以是相对路径或绝对路径。
topdown是一个布尔值,表示遍历时是否先遍历当前目录,再遍历子目录。如果为True(默认值),则先遍历当前目录,再遍历子目录;如果为False,则先遍历子目录,再遍历当前目录。
onerror是一个可选的错误处理函数,如果在遍历过程中出现错误,则会调用这个函数。
followlinks是一个布尔值,表示是否跟随符号链接。如果为True,则会跟随符号链接遍历目录;如果为False(默认值),则会忽略符号链接。
在遍历过程中,os.walk()会依次遍历指定目录及其子目录下的所有文件和目录,并返回当前目录的路径、子目录列表和文件列表。可以通过遍历返回的三元组来处理目录和文件。例如,可以使用下面的代码列出指定目录下的所有文件和子目录:
import os def list_files_and_dirs(path): for root, dirs, files in os.walk(path): print(f'Directory: {root}') for file in files: print(f' File: {os.path.join(root, file)}') for dir in dirs: print(f' Subdirectory: {os.path.join(root, dir)}') list_files_and_dirs('.')
这段代码会遍历当前工作目录及其子目录下的所有文件和目录,并输出相应的信息。
需要注意的是,os.walk()只会遍历当前目录及其子目录下的文件和目录,不会遍历符号链接所指向的文件或目录。如果需要遍历符号链接所指向的文件或目录,需要设置followlinks=True。
The above is the detailed content of How to use Python's OS module and examples. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


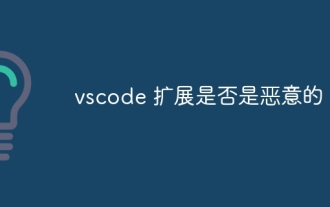
VS Code extensions pose malicious risks, such as hiding malicious code, exploiting vulnerabilities, and masturbating as legitimate extensions. Methods to identify malicious extensions include: checking publishers, reading comments, checking code, and installing with caution. Security measures also include: security awareness, good habits, regular updates and antivirus software.
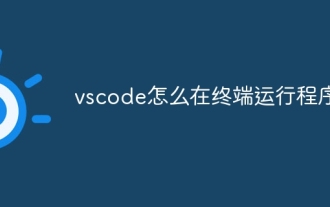
In VS Code, you can run the program in the terminal through the following steps: Prepare the code and open the integrated terminal to ensure that the code directory is consistent with the terminal working directory. Select the run command according to the programming language (such as Python's python your_file_name.py) to check whether it runs successfully and resolve errors. Use the debugger to improve debugging efficiency.
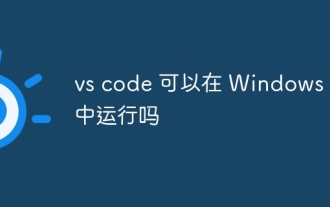
VS Code can run on Windows 8, but the experience may not be great. First make sure the system has been updated to the latest patch, then download the VS Code installation package that matches the system architecture and install it as prompted. After installation, be aware that some extensions may be incompatible with Windows 8 and need to look for alternative extensions or use newer Windows systems in a virtual machine. Install the necessary extensions to check whether they work properly. Although VS Code is feasible on Windows 8, it is recommended to upgrade to a newer Windows system for a better development experience and security.
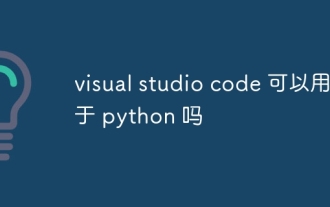
VS Code can be used to write Python and provides many features that make it an ideal tool for developing Python applications. It allows users to: install Python extensions to get functions such as code completion, syntax highlighting, and debugging. Use the debugger to track code step by step, find and fix errors. Integrate Git for version control. Use code formatting tools to maintain code consistency. Use the Linting tool to spot potential problems ahead of time.
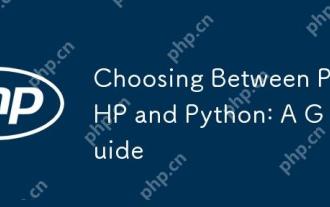
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
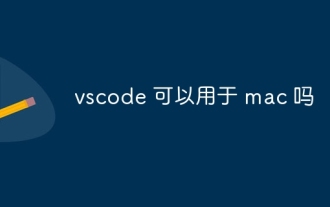
VS Code is available on Mac. It has powerful extensions, Git integration, terminal and debugger, and also offers a wealth of setup options. However, for particularly large projects or highly professional development, VS Code may have performance or functional limitations.
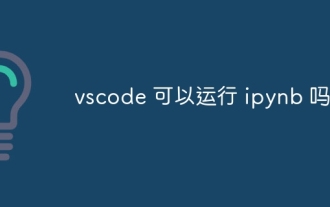
The key to running Jupyter Notebook in VS Code is to ensure that the Python environment is properly configured, understand that the code execution order is consistent with the cell order, and be aware of large files or external libraries that may affect performance. The code completion and debugging functions provided by VS Code can greatly improve coding efficiency and reduce errors.
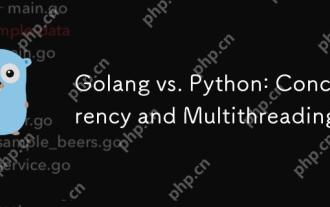
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
