How to convert php objects into arrays
In PHP, we often need to convert objects into arrays for processing or passing in different applications. Converting objects into arrays can help us manipulate data and perform data processing more conveniently. This article will introduce methods and techniques for converting PHP objects into arrays.
1. Method of converting objects into arrays
In PHP, objects can be converted into arrays through type conversion or using built-in functions.
- Using cast type conversion
In PHP, we can use the type conversion symbol to convert an object into an array. The following are the objects that need to be converted:
class Person { public $name = 'Tom'; public $age = 30; } $person = new Person;
Using forced type conversion, we can convert the above objects into arrays:
$array = (array) $person;
The resulting array structure is as follows:
Array ( [name] => Tom [age] => 30 )
- Use object to array function
In PHP, we can use the built-in function get_object_vars()
to convert an object into an associative array. This function returns an associative array consisting of object property names and property values, with property names as keys and property values as values.
The following is an example of using the get_object_vars()
function to convert an object into an array:
class Person { public $name = 'Tom'; public $age = 30; } $person = new Person; $array = get_object_vars($person);
The resulting array structure is as follows:
Array ( [name] => Tom [age] => 30 )
2. Object Tips for converting to an array
When converting an object into an array, we need to pay attention to the following points:
- Private properties cannot be converted
If The attributes of the object are private, and the attribute value cannot be obtained using the get_object_vars()
function. This is because private properties cannot be accessed outside the object. If we want to get the value of a private property, we need to use the object’s magic method __get()
.
- Object methods cannot be converted
When the object is converted into an array, the object method cannot be converted. If you need to convert the function into an array, you can use the PHP built-in function get_class_methods()
to get all the methods of the object, and convert the function into an array through loop traversal.
- Numbers and Boolean variables will be coerced into integers and Boolean types
When converting an object into an array, the numeric and Boolean variables in the object properties will be Cast to integer and boolean.
The following is an example:
class Person { public $name = 'Tom'; public $age = 30; public $isMale = true; } $person = new Person; $array = (array) $person;
The obtained array structure is as follows:
Array ( [name] => Tom [age] => 30 [isMale] => 1 )
- When there is an object in the object attribute, it can be converted recursively
If there are other objects in the object properties, we can also recursively convert them into arrays. The following is an example:
class Address { public $street = '123 Main St'; } class Person { public $name = 'Tom'; public $age = 30; public $address; function __construct() { $this->address = new Address; } } $person = new Person; $array = (array) $person;
The resulting array structure is as follows:
Array ( [name] => Tom [age] => 30 [address] => Array ( [street] => 123 Main St ) )
Summary
In PHP, we can cast the type or use the built-in function get_object_vars( )
Convert object to array. There are some techniques that need to be paid attention to during the conversion process. For example, private attributes need to be obtained through the magic method __get()
. Numbers and Boolean variables will be forced to be converted into integers and Boolean types. When other objects exist in the object attributes, they can be recursed. conversion etc. Mastering the methods and techniques of converting objects into arrays can help us perform data operations and processing more conveniently.
The above is the detailed content of How to convert php objects into arrays. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
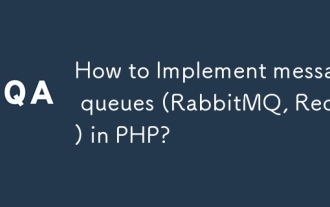
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
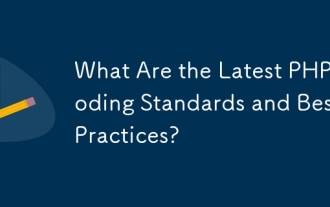
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
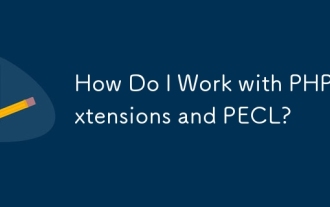
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
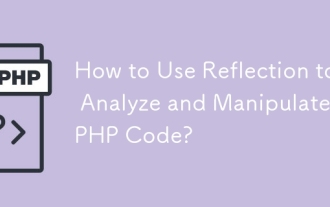
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
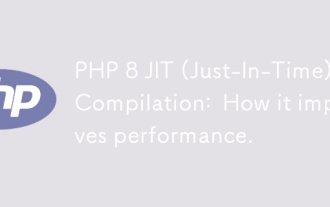
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
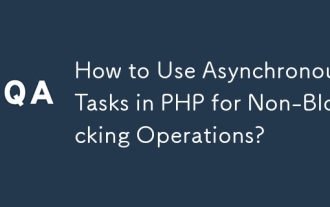
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
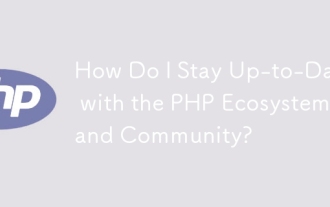
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
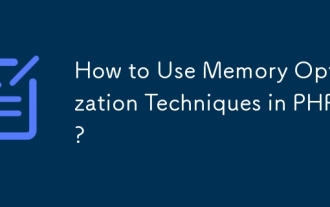
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v
