How to implement laravel facade principle
Apr 23, 2023 am 09:13 AMLaravel is an extremely popular PHP framework, and many of its features have become standards for PHP development. Laravel Facade is a very commonly used feature that simplifies development and makes code easier to read, understand, and maintain. What’s the rationale behind the facade? In this article, we will discuss how the facade pattern works and how to implement it in Laravel.
Facade Pattern
Facade pattern is a design pattern used to provide a simplified access interface for an existing complex code base. This interface is typically a static method that can call complex, object-oriented code bases, simplifying access in a simple or easy-to-understand manner. The implementation of the Facade pattern can simplify code and improve readability and maintainability.
Users of the facade mode do not need to understand complex implementation details and only need to call the static interface to complete the target operation. Therefore, facades provide a better interface to hide complexity and provide an easier-to-use API.
In Laravel, facades are used to represent services in Laravel. For example, when accessing a cache service, you can use Laravel's Cache facade.
Laravel Facade Principle
In Laravel, the facade is an abstract class used to access services. Laravel provides many services, which can be accessed through the facade. The facade hides the implementation details of the service container, making it easier for developers to use and maintain the service.
Laravel’s built-in facade is the same as the facade you define. However, facade implementation in Laravel is somewhat special.
The facade has two main parts: the facade base class belonging to Laravel, and the facade class you define.
Facade base class
Laravel's facade base class provides a lot of conveniences for your facade. It has the following functions:
- Static proxy
- Access service container
- Instantiate facade class
Laravel provides an Illuminate\ The Support\Facades namespace can be used to define facades. The DoSomething facade can be defined as:
namespace Illuminate\Support\Facades; class DoSomething extends Facade { protected static function getFacadeAccessor() { return 'Something'; } }
This facade class inherits from Illuminate\Support\Facades\Facade. This class has a protected getFacadeAccessor method that returns "Something". Laravel then uses this method to retrieve the instance associated with the facade. In this case, it will retrieve an instance named Something from the service container.
Facade class
The name of the facade class is arbitrary, but usually the name is the same as the service name, and both use camel case naming.
The facade class is where the facade is actually used. It is usually a singleton instance and can be used to accomplish many different tasks.
For example, the following is an example of a facade class:
namespace App\Facades; use Illuminate\Support\Facades\Facade; class MyService extends Facade { protected static function getFacadeAccessor() { return 'my-service'; } }
- The base class determines how the Facade class implements operations, not the facade class. The Facade class does not need to implement any functions.
- The facade class implements all operations.
In this example, the facade class "MyService" inherits from the Facade base class and implements the getFacadeAccessor() method to retrieve the instance in the service container. In this example, the facade uses an instance named " my-service ".
Usage of Facade
Laravel facade is one of the most common methods used to access services. Used extensively in the architecture, it enables you to manage and consume services easily.
Here is an example of how to use facade in a Laravel application:
use App\Facades\MyService; // 调用门面静态方法 MyService::doSomething();
When you call the facade, Laravel further uses the facade base class and facade class to handle the request. Laravel uses the facade base class to instantiate the facade class and calls back the static doSomething() method of the facade class.
Another common use case is advanced configuration. Facades can also be used to access configuration options in configuration files:
use Illuminate\Support\Facades\Config; // 获取配置选项 $debug_mode = Config::get('app.debug');
When you call the facade, Laravel retrieves the contents of the configuration file. The Config facade reads the configuration file and returns an option named "debug" in the config/app.php file. It doesn't need to specify anything else.
Benefits of the facade
The facade pattern has several benefits:
- Provides a simple interface that can access more complex code bases.
- Supports user-level access control and allows you to hide the implementation details of your classes.
- Simplify the code, making it easier to understand and maintain.
In Laravel, facades provide the same benefits at a higher level:
- Simplify nested code that accesses services.
- Improved scalability by making the service management system more accessible.
- Supports advanced configuration to enable the program to adapt to more environments.
Conclusion
The Facade pattern is a useful design pattern for accessing more complex code bases. The Laravel facade facilitates access to services and provides a more visually appealing interface, reducing code complexity and maintenance difficulty.
Understanding how facades work in Laravel is crucial to using and implementing them correctly. Laravel's built-in facades provide a convenient and secure way to access services, but you can also create your own facade classes if needed.
Using facades can greatly simplify code and liberate developers' attention from complex implementation details, allowing them to better focus on business logic. If you haven't tried facades yet, now is the time to start.
The above is the detailed content of How to implement laravel facade principle. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
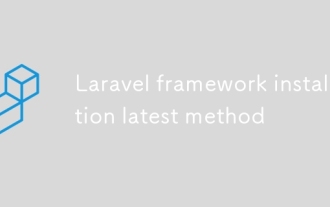
Laravel framework installation latest method
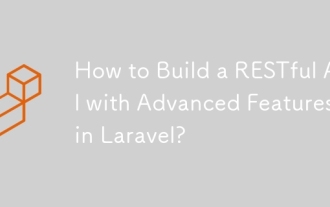
How to Build a RESTful API with Advanced Features in Laravel?
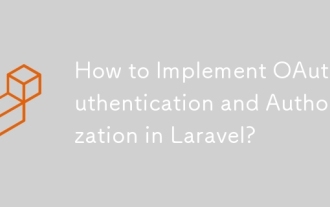
How to Implement OAuth2 Authentication and Authorization in Laravel?
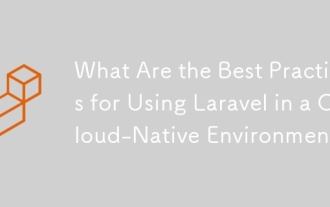
What Are the Best Practices for Using Laravel in a Cloud-Native Environment?
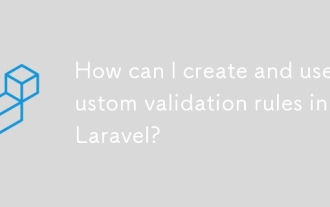
How can I create and use custom validation rules in Laravel?
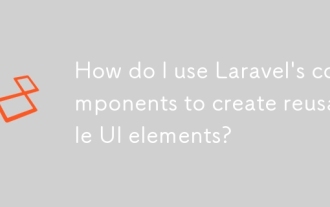
How do I use Laravel's components to create reusable UI elements?
