How to convert string to json format in php
In PHP, converting a string to a JSON string array is a common operation. JSON (JavaScript Object Notation) is a lightweight data exchange format often used in web applications. There are many ways to convert strings to JSON. Below we will introduce some of the most commonly used methods in detail.
- json_encode() function
json_encode() function is one of the easiest ways to convert PHP data types to JSON strings. It accepts a PHP value and returns a JSON encoded string.
The following is an example:
<?php $string = '{"name":"John", "age":30, "city":"New York"}'; $jsonArray = json_encode($string); echo $jsonArray; ?>
In the above code, we use the json_encode() function to convert a string into a JSON string array. In the output, we can see the JSON string array.
- json_decode() function
json_decode() function is one of the most common methods to convert a JSON string array to a PHP array. This function will accept a JSON string and return a PHP variable.
The following is an example:
<?php $json = '{"name":"John", "age":30, "city":"New York"}'; $array = json_decode($json); print_r($array); ?>
In the above code, we have used the json_decode() function to convert the string into a PHP array.
- Using Array Functions
If you have an array consisting of strings and need to return it as a JSON string, then you can use the PHP array functions The json_encode() function does this.
The following is an example:
<?php $stringArray = array('John', 'Jane', 'Peter'); $jsonArray = json_encode($stringArray); echo $jsonArray; ?>
In the above code, we have used the json_encode() function to convert the string array into a JSON string. In the output, we can see the JSON string array.
Summary:
In PHP, there are many ways to convert strings to JSON string arrays. Whether you want to convert a string to a JSON string array, or a JSON string array to a PHP array, the above method can do it easily. Using one of these methods, you can save time and code and focus on other aspects of development.
The above is the detailed content of How to convert string to json format in php. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


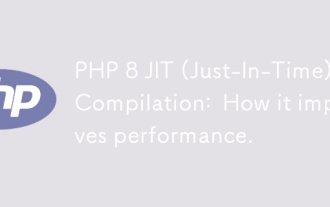
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
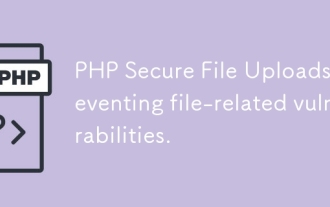
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
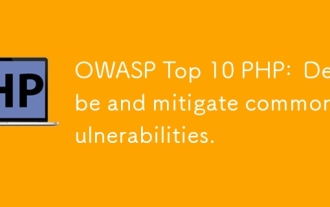
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
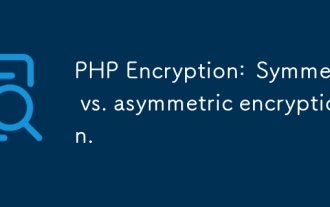
The article discusses symmetric and asymmetric encryption in PHP, comparing their suitability, performance, and security differences. Symmetric encryption is faster and suited for bulk data, while asymmetric is used for secure key exchange.

The article discusses implementing robust authentication and authorization in PHP to prevent unauthorized access, detailing best practices and recommending security-enhancing tools.

Prepared statements in PHP enhance database security and efficiency by preventing SQL injection and improving query performance through compilation and reuse.Character count: 159
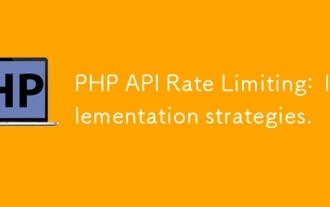
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
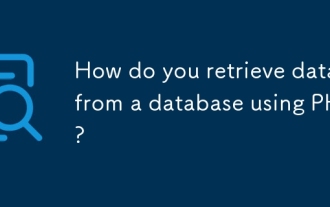
Article discusses retrieving data from databases using PHP, covering steps, security measures, optimization techniques, and common errors with solutions.Character count: 159
