How does js receive an array from php?
With the development of modern web development, the requirements for web applications are becoming more and more complex. Front-end development pays more attention to performance and user experience, while back-end development pays more attention to data processing and logic implementation. PHP and JavaScript are two high-level languages widely used in web development. In actual development, PHP is usually used for back-end data processing, while JavaScript is used for front-end control and user interaction. When these two languages need to communicate, how can the transmission and sharing of data be completed? This article will introduce how to receive PHP arrays in JavaScript.
1. Understanding PHP arrays
In PHP, arrays are a very common data type. It is essentially an ordered, key-value pair data structure, where the keys are strings or integers and the values can be variables of any type. PHP arrays can be used to store multiple values and perform operations on these values. In PHP, you can define an array in the following way:
$arr = array('apple', 'orange', 'banana');
At this time, $arr is an array containing three elements, namely 'apple', 'orange', and 'banana'. You can get the elements in the array in the following ways:
echo $arr[0]; //输出apple echo $arr[1]; //输出orange echo $arr[2]; //输出banana
2. Convert the PHP array to JSON format
In JavaScript, PHP variables cannot be directly accessed, so you need to convert the PHP array In a format that JavaScript can parse. A common way is to convert PHP arrays into JSON format. JSON, short for JavaScript Object Notation, is a lightweight data exchange format that is easy to read and write. Use the json_encode function in PHP to convert an array into a string in JSON format, for example:
$arr = array('apple', 'orange', 'banana'); echo json_encode($arr);
This code will output the following JSON string:
["apple","orange","banana"]
3. Receive PHP through JavaScript Array
In JavaScript, you can use the built-in JSON object to parse JSON strings and convert them into JavaScript objects. When receiving an array from PHP, you first need to receive the JSON string passed by PHP in JavaScript, and then convert it into a JavaScript object through the JSON.parse function.
// 假设PHP端传递过来的JSON字符串为'["apple","orange","banana"]' // 第一步:通过XMLHttpRequest获取JSON字符串 var xhr = new XMLHttpRequest(); xhr.open('GET', 'path/to/phpfile.php', true); xhr.send(); xhr.onreadystatechange = function() { if (xhr.readyState == 4 && xhr.status == 200) { var data = JSON.parse(xhr.responseText); //解析JSON字符串为JavaScript对象 //TODO: 对data对象进行操作 } };
In the above code, obtain the JSON string passed from the PHP side through XMLHttpRequest, convert it into a JavaScript object using the JSON.parse method, and then operate on the object.
4. Notes
When receiving PHP arrays through JavaScript, you need to pay attention to the following issues:
- Arrays in JSON format must be passed in PHP Generated by the json_encode function, otherwise it cannot be parsed in JavaScript.
- It is necessary to ensure the security of data during the transmission process to avoid malicious tampering of data.
- When an array in PHP is converted to JSON format, only the index array will be converted into a JSON array. If it is an associative array, it needs to be nested in a JSON object in the form of key-value pairs.
5. Summary
This article introduces how to receive PHP arrays in JavaScript. By converting PHP arrays into JSON strings and then parsing them into JavaScript objects in JavaScript, data transmission and sharing can be easily achieved. In actual development, we need to consider the security and validity of data to ensure the correctness and integrity of the data.
The above is the detailed content of How does js receive an array from php?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
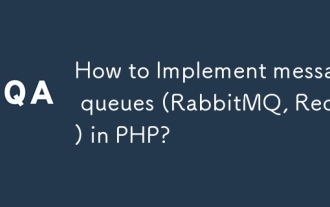
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
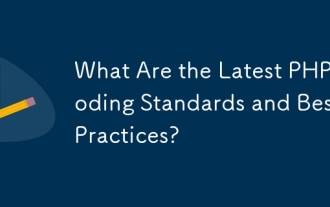
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
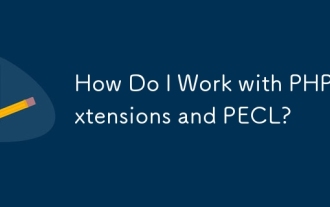
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
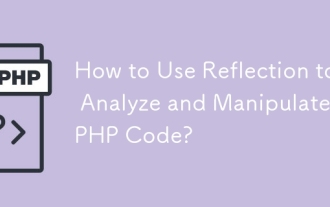
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
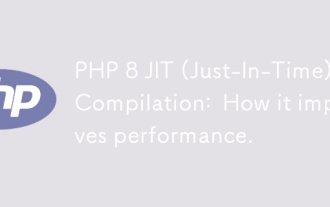
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
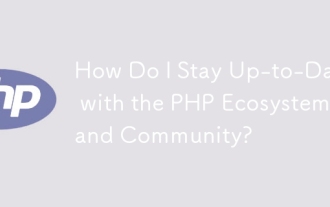
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
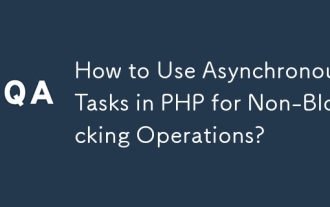
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
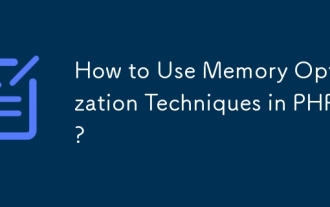
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v
