Let's talk about the traversal method of arrays in php
Array in PHP is a very common data structure. During the development process, we often need to traverse the array in order to obtain all elements or process each element. PHP provides a variety of methods for traversing arrays. This article will introduce the methods for traversing arrays in PHP.
- for loop traverses arrays
The for loop is a basic loop structure that can also be used to traverse arrays in PHP. The sample code is as follows:
$fruits = array('apple', 'banana', 'cherry'); for ($i = 0; $i < count($fruits); $i++) { echo $fruits[$i] . "<br>"; }
In the above code, a for loop is used to traverse the array $fruits. The count function is used to obtain the number of array elements. $i represents the loop variable. Each loop will output the an element.
- foreach loop traverses an array
The foreach loop in PHP is a very convenient way to traverse an array. The foreach loop can iterate over any type of array, including associative arrays and ordinary arrays. The sample code is as follows:
$fruits = array('apple', 'banana', 'cherry'); foreach ($fruits as $fruit) { echo $fruit . "<br>"; }
In the above code, a foreach loop is used to traverse the array $fruits, where $fruit represents the current element in each loop. The foreach loop executes the loop body once on each element without using loop variables.
- While loop traverses the array
The while loop is another basic loop structure that can also be used to traverse the array in PHP. The sample code is as follows:
$fruits = array('apple', 'banana', 'cherry'); $i = 0; while ($i < count($fruits)) { echo $fruits[$i] . "<br>"; $i++; }
In the above code, a while loop is used to traverse the array $fruits, $i represents the loop variable, and each loop will output an element in the array. This traversal method is similar to a for loop, except that a while statement is used.
- do-while loop traverses the array
The do-while loop is also a basic loop structure and can also be used to traverse the array in PHP. The sample code is as follows:
$fruits = array('apple', 'banana', 'cherry'); $i = 0; do { echo $fruits[$i] . "<br>"; $i++; } while ($i < count($fruits));
In the above code, a do-while loop is used to traverse the array $fruits, $i represents the loop variable, and each loop will output an element in the array. This traversal method is similar to the while loop, except that the do-while loop will execute the loop body at least once.
- array_walk function traverses the array
There is also a powerful function array_walk in PHP, which can be used to traverse the array and perform some operations on each element. The sample code is as follows:
$fruits = array('apple', 'banana', 'cherry'); function printFruit($value) { echo $value . "<br>"; } array_walk($fruits, 'printFruit');
In the above code, the array_walk function is used to traverse the array $fruits. The first parameter is the array to be traversed, and the second parameter is the name of the function to be executed. The printFruit function is used to output the elements in the array. The array_walk function executes the function once on each element.
Summary
There are many array traversal methods in PHP, among which for loop, foreach loop, while loop and do-while loop are the most basic methods, and the array_walk function is one Advanced methods that can be used to customize the handling of each element. In actual development, different scenarios may require the use of different traversal methods, and the most appropriate method needs to be selected according to specific requirements.
The above is the detailed content of Let's talk about the traversal method of arrays in php. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
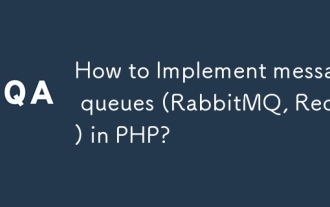
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
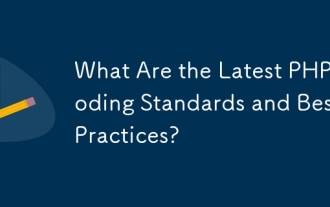
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
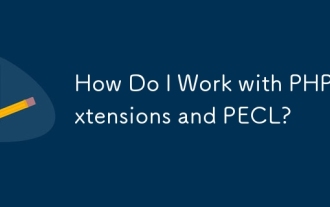
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
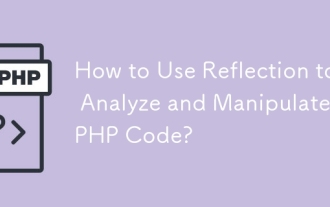
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
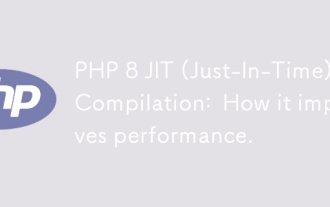
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
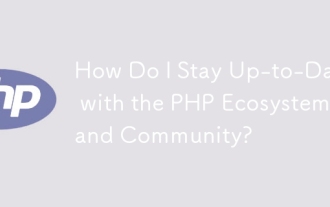
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
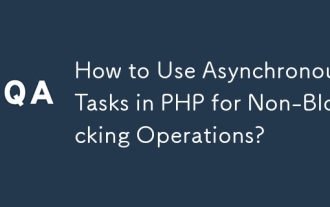
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
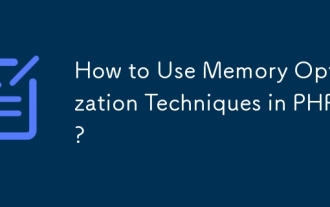
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v
