Discuss how to loop through arrays in PHP
PHP is a commonly used server-side scripting language that often uses arrays to store a set of related data, such as some product information, user information, etc. In PHP, looping an array is also a very basic and commonly used operation. This article will explore how to loop through arrays in PHP.
1. for loop
The for loop is the most basic loop statement in PHP and is usually used to traverse arrays. For example, we have an array with 5 elements, each element represents a person's age:
$ages = array(18, 25, 30, 40, 50);
To To output each element in the array in turn, you can use the following code:
for($i = 0; $i < count($ages); $i ) {
echo $ages[$i] . "<br>";</p> <p>}</p> <p>In the above code, we use $i as the loop variable, starting from 0 and ending the loop with the array length -1. Each loop outputs the element at the corresponding position in the array. </p> <p>2. foreach loop<br>In addition to the for loop, PHP also provides a more advanced method for looping through the array - the foreach loop. The foreach statement can directly traverse the entire array without using a loop variable, as shown below: </p> <p>foreach($ages as $age) {</p> <pre class="brush:php;toolbar:false">echo $age . "<br>";
}
in the above In the code, we define an $age variable to store the array elements for each loop. The foreach statement will automatically assign each element in the array to the $age variable, and then execute the code in the loop body. This will loop through the entire array and output the value of each element.
When using a foreach loop to traverse the array, you can also get the key name of each element in the array. If the array is an associative array, the key name is the subscript of the array element; if it is an indexed array, the key name is the position of the array element. The following is an example of using a foreach loop to output an associative array:
$person = array("name"=>"Tom", "age"=>25, "gender"=>"male" );
foreach($person as $key => $value) {
echo $key . ": " . $value . "<br>";
}
In the above code, we use a foreach loop to traverse the $person array , each loop obtains a key name and corresponding value. The variable $key stores the key name, the variable $value stores the key value, and then the combination of the two is output.
3. While loop
In PHP, you can also use a while loop to traverse an array. The while loop is suitable for situations where the same loop body needs to be executed repeatedly, such as looping to read data in a file. The following is an example of using a while loop to iterate through an array:
$names = array("Tom", "Jerry", "Mike", "Kitty");
$count = count($names) ;
$i = 0;
while($i < $count) {
echo $names[$i] . "<br>"; $i++;
}
In the above code, we use while loop to traverse $names array, variable $i represents the position of the array element. As long as $i is less than the array length, the code in the loop body is executed. Each loop outputs the element at the current position and increments the value of $i by 1 until the entire array is traversed.
To sum up, there are three common ways to loop through arrays in PHP, namely for loop, foreach loop and while loop. Among them, the for loop is the most basic and common method, while the foreach loop is more advanced and can directly traverse the entire array and obtain key names and key values. The while loop is suitable for situations where the same loop body needs to be executed repeatedly. It can be used to traverse the array and perform operations that need to be executed repeatedly. According to different requirements and situations, we can choose different loop methods to traverse the array.
The above is the detailed content of Discuss how to loop through arrays in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
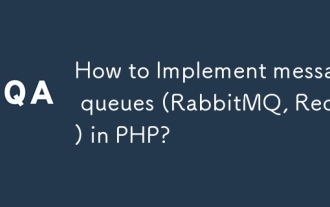
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
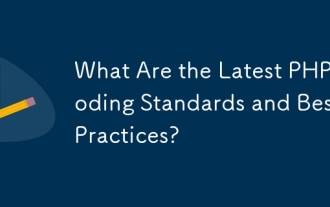
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
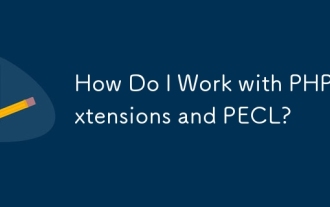
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
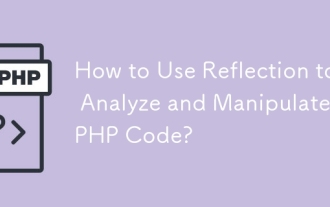
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
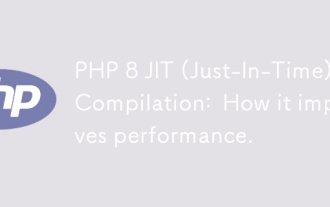
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
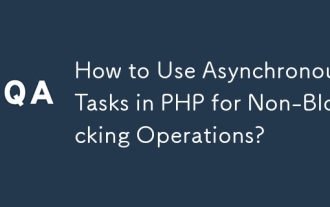
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
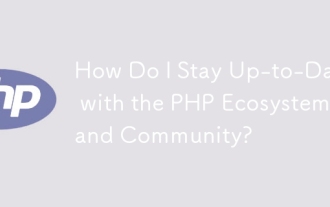
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
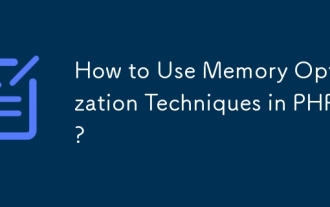
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v
