How to find the median from an unordered array in php
In PHP, array is a very powerful data structure. With PHP arrays, we can easily store and manipulate large amounts of data. But what do we do when we need to find the median from an unordered array?
The median (also called the median) is the middle value in an array. It divides the array into two parts. All values on the left are smaller than it and all values on the right are larger. . If the array has an even number of elements, the median is the average of the middle two elements.
Although PHP provides the sort() function, which can be used to sort arrays, when sorting large arrays, the time complexity of this function will reach O(nlogn) and the sorting of the array will be modified. order, this is not what we want.
So, how do we find the median of an unordered array in PHP without changing the order of the array? Let us take a look below.
- Use quick sort to find the median number
The quick sort algorithm is a comparison-based sorting algorithm, and its time complexity under average circumstances is O(nlogn) , and does not require additional memory space. Therefore, we can use quick sort to sort the array and find the median.
The main idea of the quick sort algorithm is to select a benchmark element in the array, and then divide the array into two parts. One part contains all values that are smaller than the benchmark element, and the other part contains all values that are greater than the benchmark element. . These two parts are then sorted recursively until the entire array is sorted.
In order to find the median of an unordered array, we can use the quick sort algorithm to sort it first, and then determine the median based on the length of the sorted array.
The following is an example of PHP code that uses quick sort to find the middle number:
function quickSort($arr){ $len = count($arr); if($len <= 1){ return $arr; } $pivot = $arr[0]; $left_arr = array(); $right_arr = array(); for($i=1; $i<$len; $i++){ if($arr[$i] <= $pivot){ $left_arr[] = $arr[$i]; }else{ $right_arr[] = $arr[$i]; } } $left_arr = quickSort($left_arr); $right_arr = quickSort($right_arr); return array_merge($left_arr, array($pivot), $right_arr); } $arr = array(3, 9, 2, 7, 1, 5, 6); $sorted_arr = quickSort($arr); $len = count($sorted_arr); if($len % 2 == 0){ $middle = ($sorted_arr[$len/2-1] + $sorted_arr[$len/2])/2; }else{ $middle = $sorted_arr[($len-1)/2]; } echo "中数为:".$middle;
- Uses heap sort to find the middle number
The heap sort algorithm is a Sorting algorithm for heap-based tree data structures. In heap sort, we sort the array by building a max-heap or min-heap. We can use max heap to find the median of an unordered array.
Maximum heap is a binary tree that satisfies the following properties:
1. The value of each node of the heap is greater than or equal to the value of its left and right child nodes.
2. The heap is always a complete binary tree.
For an unordered array, we can sort it by building a max heap. Then, we can find the median based on the properties of the maximum heap.
The following is an example of PHP code that uses heap sort to find the median:
function buildMaxHeap(&$arr, $index, $heapSize){ $left = 2*$index+1; $right = 2*$index+2; $max = $index; if($left<$heapSize && $arr[$left]>$arr[$max]){ $max = $left; } if($right<$heapSize && $arr[$right]>$arr[$max]){ $max = $right; } if($max != $index){ $temp = $arr[$index]; $arr[$index] = $arr[$max]; $arr[$max] = $temp; buildMaxHeap($arr, $max, $heapSize); } } function heapSort(&$arr){ $heapSize = count($arr); for($i=intval($heapSize/2)-1; $i>=0; $i--){ buildMaxHeap($arr, $i, $heapSize); } for($i=$heapSize-1; $i>=1; $i--){ $temp = $arr[0]; $arr[0] = $arr[$i]; $arr[$i] = $temp; $heapSize--; buildMaxHeap($arr, 0, $heapSize); } } $arr = array(3, 9, 2, 7, 1, 5, 6); heapSort($arr); $len = count($arr); if($len % 2 == 0){ $middle = ($arr[$len/2-1] + $arr[$len/2])/2; }else{ $middle = $arr[($len-1)/2]; } echo "中数为:".$middle;
Summary
The above are two methods of finding the median in an unordered array in PHP. Although their time complexity is different, they can all implement the functions of quickly sorting unordered arrays and finding the median. If you need to sort a large number of unordered arrays and find the median, it is recommended that you use the heap sort algorithm, which has better time complexity performance.
The above is the detailed content of How to find the median from an unordered array in php. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
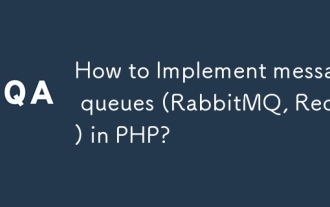
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
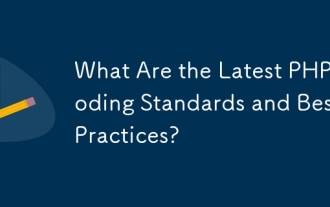
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
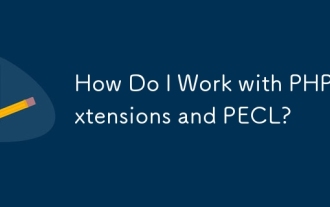
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
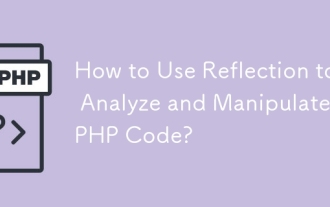
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
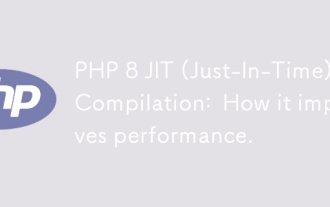
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
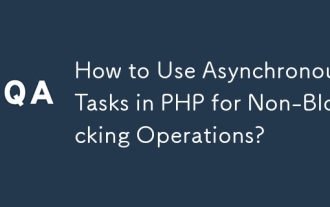
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
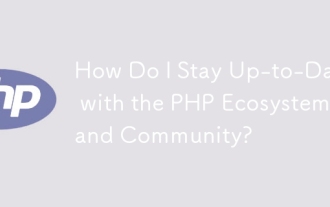
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
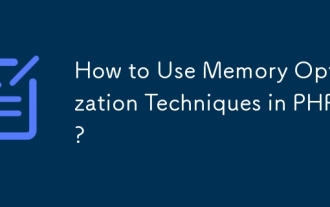
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v
