


How to determine whether a value is in a two-dimensional array in PHP (two methods)
In PHP, there are many ways to determine whether a value is in a two-dimensional array. This article will introduce the two most commonly used methods.
Method 1: Use foreach loop to traverse
This method is more intuitive. You can use foreach loop to traverse the entire two-dimensional array, and then determine whether each sub-array contains the target value. If the target value is found, you can return true directly, otherwise it will return false after the loop ends.
The following is a code example:
function isValueInArray($array, $value) { foreach ($array as $subArray) { if (in_array($value, $subArray)) { return true; } } return false; }
This function accepts two parameters: the two-dimensional array to be queried and the target value. Inside the function, we use a foreach loop to iterate through the entire array, and use the in_array function to determine whether the target value exists in each sub-array. If the target value is found, you can return true directly, otherwise it will return false after the loop ends.
Method 2: Use the array_column function
PHP’s array_column function can return all values of the specified key from a two-dimensional array. We can use this function to get a column in a two-dimensional array, and then use the in_array function to determine whether the target value exists in the column. If it exists, it can return true, otherwise it returns false.
The following is a code example:
function isValueInArray($array, $value) { foreach ($array as $subArray) { if (in_array($value, array_column($array, 'key'))) { return true; } } return false; }
The implementation of this function is basically the same as the above function. The difference is that we use the array_column function to obtain the "key" column in the two-dimensional array, and then Use the in_array function to determine whether the target value appears in the column.
Summary
The above are two common methods to determine whether a value is in a two-dimensional array in PHP. Using a foreach loop is more intuitive, but performance may be affected when the array is large. Using the array_column function can get the specified column faster, but this function may not be supported for some older PHP versions. Therefore, a combination of considerations is needed when choosing which method to use.
The above is the detailed content of How to determine whether a value is in a two-dimensional array in PHP (two methods). For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


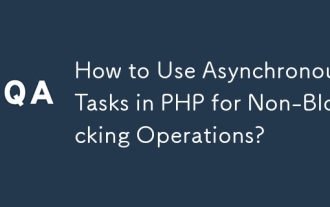
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
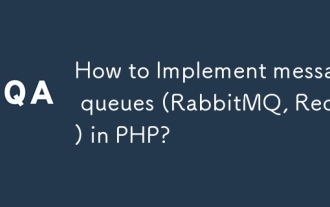
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
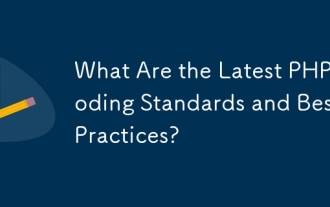
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
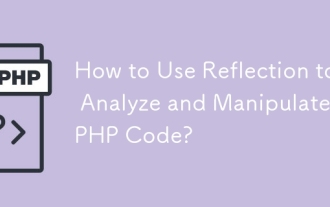
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
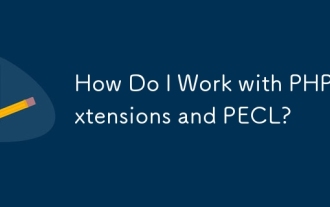
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
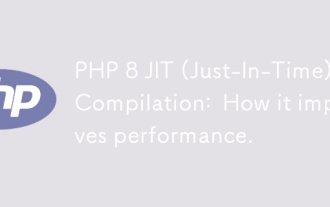
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
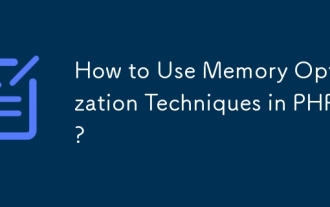
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v
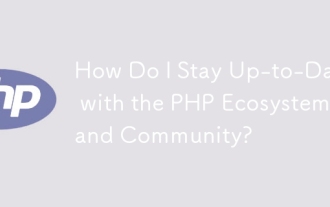
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
