How to remove css in jq
JQuery (jq for short) is a popular JavaScript library that is widely used in web development. In jq, there is a very convenient method to remove css styles, which is very useful for web designers and developers. This article will introduce how to use jq to remove css styles.
First, we need to understand two jq methods: .removeAttr() and .css(). removeAttr() is used to remove the specified attribute from the element. For example, we can use the following code to remove the title attribute from a div element:
$('div').removeAttr('title');
.css() method is used to get or set the value of the CSS property. For example, we can set the color of a div element to red using the following code:
$('div').css('color', 'red');
Now, let’s take a look at how to use these two methods to remove css styles.
Suppose we have an html structure like this:
<div class="demo" style="color: red; font-size: 16px;">Some content</div>
We want to remove specific styles from this div element, such as color and font-size. Here's how to remove css styles using jq:
$('.demo').removeAttr('style').css({ 'color': '', 'font-size': '' });
Here, we first remove the style attribute from the element using the .removeAttr() method. We then use the .css() method to set the style to be removed to an empty string. This will overwrite the original value and remove the style from the element.
You can also use a simpler method to remove all styles. The following is the code:
$('.demo').attr('style', '');
Here, we use the .attr() method to set the value of the style attribute to an empty string. This will remove all styles from the element.
It should be noted that these methods only remove inline styles. If the element's styles are inherited from an external style sheet or internal style block, these methods will not remove them. If you want to completely remove the style, you need to use other methods, such as setting the element's class to a new style or overriding the original style.
In actual use, removing css styles may be very useful in some specific situations. For example, when an element has too many inline styles that are difficult to refactor, it may be more convenient to remove them. In addition, if you want to implement dynamic style switching, removing the original style is also a necessary step.
To summarize, this article introduces how to use jq to remove css styles. We learned about the use of the .removeAttr() and .css() methods, and saw how they can be combined to remove styles from an element. These methods can be very useful if you need to remove css styles from a real project.
The above is the detailed content of How to remove css in jq. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
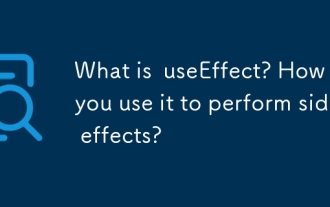
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.

Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.

Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
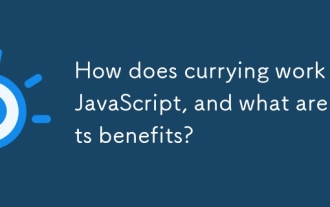
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
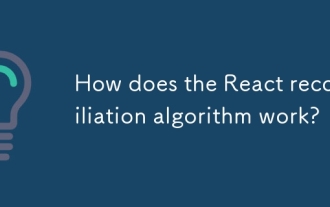
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
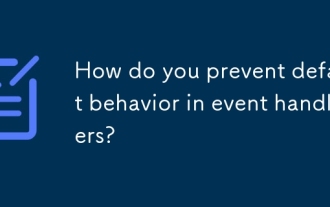
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
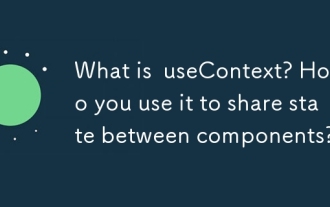
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
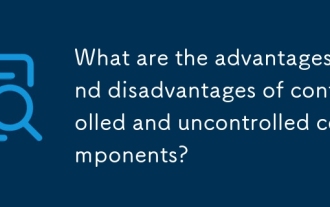
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.
