How to implement dynamic methods in Go language
As a statically typed language, Go language usually requires that data types must be clearly expressed when writing code, which helps the compiler perform optimization and error checking during compilation. However, sometimes we also need to dynamically modify types and their methods at runtime. This article will introduce how to implement dynamic methods in Go language.
Dynamic types and dynamic methods in Go language are implemented through type assertion and reflection mechanisms. Type assertion is a way of converting an interface type to its underlying type. Its basic syntax is "x.(T)", where x is a variable of interface type and T is a concrete type. If the underlying type of x is T, then this assertion will return a value of type T. If x is not a variable of type T, this assertion will produce a runtime error.
Another mechanism for implementing dynamic methods is reflection. Reflection is a powerful tool that provides the runtime type information of the program and the structure information of the program itself, giving the program the ability to operate its own structure at runtime.
Now, let us take a look at how to use type assertion and reflection to implement dynamic methods in Go language.
First, we need to define a dynamic type. This type will contain a structure that defines a field value and a name representing the type. This type also needs to implement a method call, which will use reflection to call related methods.
package main
import (
"fmt" "reflect"
)
type DynamicType struct {
value interface{} // 动态类型的值 name string // 动态类型的名称
}
// Define a dynamic method
func (dt *DynamicType) call(method string, args ...interface{}) (result []interface{}, err error) {
// 获取动态类型的值的类型信息 valueType := reflect.TypeOf(dt.value) // 获取动态类型的值的值信息 valueValue := reflect.ValueOf(dt.value) // 获取方法信息 methodName := reflect.ValueOf(method) methodValue := valueValue.MethodByName(method) // 检查是否存在该方法 if !methodValue.IsValid() { return nil, fmt.Errorf("method %s does not exist", method) } // 定义方法的参数 var input []reflect.Value for _, arg := range args { input = append(input, reflect.ValueOf(arg)) } // 调用方法 resultValue := methodValue.Call(input) // 定义返回值 for _, rv := range resultValue { result = append(result, rv.Interface()) } return result, nil
}
Next, we can define a structure as an instance of the dynamic type.
type Person struct {
Name string Age int
}
Suppose we have an Add method that adds 1 to the age.
func (p *Person) Add() {
p.Age += 1
}
We can create an instance of a dynamic type using the following method.
p := &Person{
Name: "Tom", Age: 20,
}
dynamicType := &DynamicType{
value: p, name: "Person",
}
Now, we can call dynamic Method Call:
, = dynamicType.Call("Add")
If we want to add a method dynamically, we can use the following function:
func AddMethod(dynamicType *DynamicType, methodName string, method func(interface{})) error {
// 获取动态类型的值的类型信息 valueType := reflect.TypeOf(dynamicType.value) // 获取动态类型的值的值信息 valueValue := reflect.ValueOf(dynamicType.value) // 判断方法是否已经存在 _, ok := valueType.MethodByName(methodName) if ok { return fmt.Errorf("method %s already exists", methodName) } // 定义方法 methodValue := reflect.MakeFunc(reflect.FuncOf([]reflect.Type{valueType}, []reflect.Type{}, false), func(args []reflect.Value) []reflect.Value { method(args[0].Interface()) return nil }) // 新增方法 valuePtr := reflect.New(valueType).Elem() valuePtr.Set(valueValue) valuePtr.Addr().MethodByName(methodName).Set(methodValue) // 更新动态类型的值信息 dynamicType.value = valuePtr.Interface() return nil
}
Finally, we can use the following code to add a Subtract method to the Person type:
AddMethod(dynamicType, "Subtract", func(value interface{}) {
p := value.(*Person) p.Age -= 1
})
Now, we can use the dynamic method Subtract to reduce Tom's age .
, = dynamicType.Call("Subtract")
The above is a simple method to implement dynamic methods using Go language. Although this mechanism may not be as efficient as defining complete types and methods at compile time, it allows us to perform dynamic types and dynamic methods at runtime, which is indispensable for dynamism and flexibility.
The above is the detailed content of How to implement dynamic methods in Go language. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
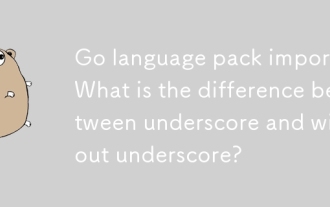
This article explains Go's package import mechanisms: named imports (e.g., import "fmt") and blank imports (e.g., import _ "fmt"). Named imports make package contents accessible, while blank imports only execute t

This article explains Beego's NewFlash() function for inter-page data transfer in web applications. It focuses on using NewFlash() to display temporary messages (success, error, warning) between controllers, leveraging the session mechanism. Limita
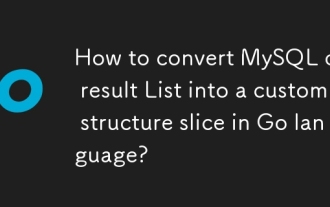
This article details efficient conversion of MySQL query results into Go struct slices. It emphasizes using database/sql's Scan method for optimal performance, avoiding manual parsing. Best practices for struct field mapping using db tags and robus
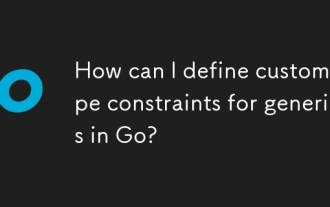
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
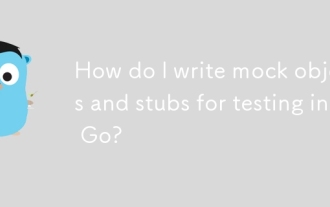
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
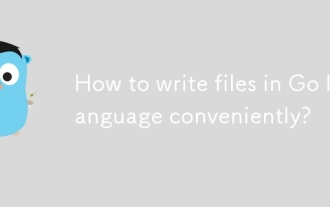
This article details efficient file writing in Go, comparing os.WriteFile (suitable for small files) with os.OpenFile and buffered writes (optimal for large files). It emphasizes robust error handling, using defer, and checking for specific errors.
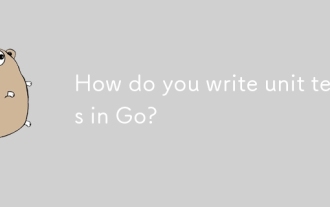
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
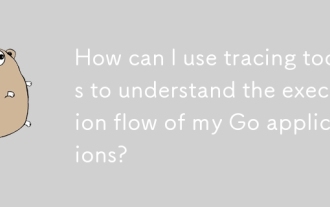
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
