How to make a call with parameters in Oracle stored procedure
Oracle stored procedure is a pre-compiled reusable SQL statement that is stored in the database and can be executed and called at any time. In Oracle stored procedures, you can use parameters to achieve more flexible calling. This article explains how to use parameters in Oracle stored procedures.
Create a stored procedure and define parameters
In Oracle database, creating a stored procedure requires the use of the CREATE PROCEDURE
statement. Here is a simple example:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
|
The above code creates a stored procedure named get_employee_details
that has four parameters: employee_id
(IN input parameters of type), employee_name
, hire_date
, and salary
(output parameters of type OUT). The function of the stored procedure is to query the employee's detailed information based on the employee_id
parameter and store the query results in the output parameter.
Call stored procedure
When the stored procedure is successfully created, you can use the EXECUTE
statement to call it. The following is the code to call the stored procedure:
1 2 3 4 5 6 7 8 9 10 |
|
In the above code, first declare three variables emp_name
, emp_hire_date
and emp_salary
, Then call the stored procedure get_employee_details
and pass in the parameter 101
, and assign the output parameters to the variables declared above. Finally, use the PUT_LINE
function in the DBMS_OUTPUT
package to output the query results.
It should be noted that the DECLARE
and BEGIN
statements are necessary because they represent the beginning and end of a code block. In the code block, you can declare variables, call stored procedures, execute various SQL statements, etc.
Types of parameters
When defining parameters of a stored procedure, you can use the following types:
-
IN
: Indicates input parameters, these Parameters are used to pass values into stored procedures. -
OUT
: Indicates output parameters, which are used to return values from stored procedures. -
IN OUT
: Indicates that it is both an input parameter and an output parameter. These parameters can both pass values to and return values from stored procedures.
In addition to the above types, you can also use the NOCOPY
keyword to define parameters, which can avoid memory copying during parameter transfer, thereby improving execution efficiency.
Application scenarios
In actual development, parameter calling of stored procedures is very common, and it can be applied in multiple scenarios. The following are some typical application scenarios:
- Parameterized query: In the stored procedure, input parameters can be defined so that the stored procedure can execute different query statements based on different input parameters, thereby achieving parameterization. Inquire.
- Batch operation: In the stored process, you can define input parameters and output parameters, so that the stored process can perform batch operations based on the input parameters, and at the same time store the processing results in the output parameters, reducing the number of subsequent operations.
- Transaction processing: In the stored procedure, input parameters can be used to control the submission or rollback of the transaction, thereby achieving a more flexible transaction processing method.
Summary
This article introduces the method of using parameter calls in Oracle stored procedures. Typically, parameterized calls to stored procedures can greatly improve code reusability and flexibility, and can provide applications with more flexible functionality. Therefore, in actual development, parameterized calls of stored procedures should be fully utilized to improve system performance and maintainability.
The above is the detailed content of How to make a call with parameters in Oracle stored procedure. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










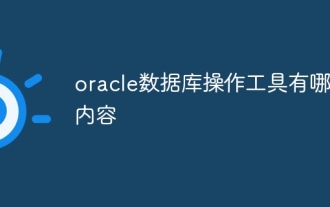
In addition to SQL*Plus, there are tools for operating Oracle databases: SQL Developer: free tools, interface friendly, and support graphical operations and debugging. Toad: Business tools, feature-rich, excellent in database management and tuning. PL/SQL Developer: Powerful tools for PL/SQL development, code editing and debugging. Dbeaver: Free open source tool, supports multiple databases, and has a simple interface.
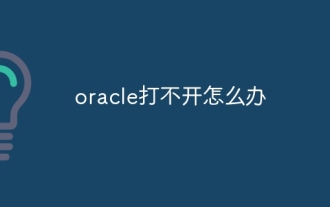
Solutions to Oracle cannot be opened include: 1. Start the database service; 2. Start the listener; 3. Check port conflicts; 4. Set environment variables correctly; 5. Make sure the firewall or antivirus software does not block the connection; 6. Check whether the server is closed; 7. Use RMAN to recover corrupt files; 8. Check whether the TNS service name is correct; 9. Check network connection; 10. Reinstall Oracle software.
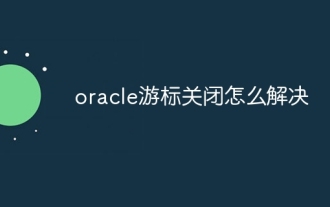
The method to solve the Oracle cursor closure problem includes: explicitly closing the cursor using the CLOSE statement. Declare the cursor in the FOR UPDATE clause so that it automatically closes after the scope is ended. Declare the cursor in the USING clause so that it automatically closes when the associated PL/SQL variable is closed. Use exception handling to ensure that the cursor is closed in any exception situation. Use the connection pool to automatically close the cursor. Disable automatic submission and delay cursor closing.
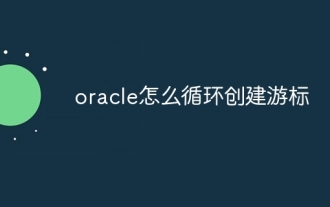
In Oracle, the FOR LOOP loop can create cursors dynamically. The steps are: 1. Define the cursor type; 2. Create the loop; 3. Create the cursor dynamically; 4. Execute the cursor; 5. Close the cursor. Example: A cursor can be created cycle-by-circuit to display the names and salaries of the top 10 employees.
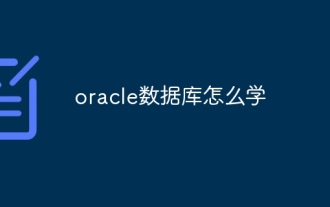
There are no shortcuts to learning Oracle databases. You need to understand database concepts, master SQL skills, and continuously improve through practice. First of all, we need to understand the storage and management mechanism of the database, master the basic concepts such as tables, rows, and columns, and constraints such as primary keys and foreign keys. Then, through practice, install the Oracle database, start practicing with simple SELECT statements, and gradually master various SQL statements and syntax. After that, you can learn advanced features such as PL/SQL, optimize SQL statements, and design an efficient database architecture to improve database efficiency and security.
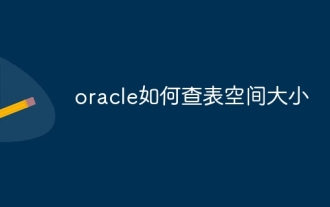
To query the Oracle tablespace size, follow the following steps: Determine the tablespace name by running the query: SELECT tablespace_name FROM dba_tablespaces; Query the tablespace size by running the query: SELECT sum(bytes) AS total_size, sum(bytes_free) AS available_space, sum(bytes) - sum(bytes_free) AS used_space FROM dba_data_files WHERE tablespace_
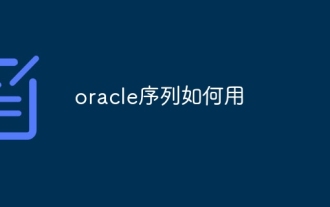
Oracle sequences are used to generate unique sequences of numbers, usually used as primary keys or identifiers. Creating a sequence requires specifying the sequence name, starting value, incremental value, maximum value, minimum value, cache size, and loop flags. When using a sequence, use the NEXTVAL keyword to get the next value of the sequence.
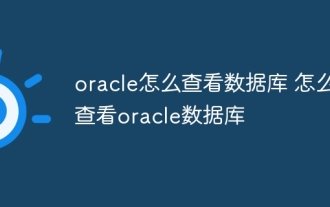
To view Oracle databases, you can use SQL*Plus (using SELECT commands), SQL Developer (graphy interface), or system view (displaying internal information of the database). The basic steps include connecting to the database, filtering data using SELECT statements, and optimizing queries for performance. Additionally, the system view provides detailed information on the database, which helps monitor and troubleshoot. Through practice and continuous learning, you can deeply explore the mystery of Oracle database.
