What should I do if golang reads garbled files?
As a development language, golang can be said to be relatively convenient in terms of file reading and writing, but there are still some problems encountered in actual development, such as garbled characters after files are read. This article will introduce the reasons and solutions for reading garbled files in golang.
1. Problem background
When we use golang to read files, sometimes the content after reading will be garbled, as shown in the following figure:
2. Cause of the problem
There are many reasons for garbled characters. The following are some common situations:
2.1. File encoding format does not match
The file encoding format refers to the encoding format of the file content when it is stored, not the extension. When golang reads files, it reads them in UTF-8 encoding format by default. If the read file is not in UTF-8 encoding format, garbled characters will appear.
For example, we can create a txt text file through the cmd command line tool of the Windows system and save it using the "gbk" encoding format, as shown in the following figure:
Then, we use the golang program to read, as shown in the following figure:
It can be found that the content of the read file is garbled. This is due to The default encoding format of golang is UTF-8.
2.2. Endianness is not handled correctly
In golang, when reading files, if the encoding format is UTF-16 (including UTF-16LE and UTF-16BE), you need to Correctly handle endianness. UTF-16LE means that in memory, the low-order bytes are stored in front and the high-order bytes are stored in the back, while UTF-16BE is the opposite.
If we do not handle the byte order correctly when reading UTF-16 files, garbled characters will appear.
2.3. Other encoding format conversion issues
Sometimes, we may need to convert files in other formats (such as CSV, XML, etc.) to the format supported by golang for reading, but during the conversion There may be encoding format conversion problems resulting in garbled characters.
3. Solution
For the above situations, we can adopt the following solutions:
3.1. Confirm the file encoding format and read it
If we already know the encoding format of the file, we need to specify the corresponding encoding format when reading the file.
golang provides a ReadFile
method of the ioutil
package, which can be used to read files very conveniently. When using this method, you can specify the file encoding format through methods such as bufio.NewReader
and ioutil.NopCloser
. The code is as follows:
func ReadFileWithCharset(filename string, charset string) ([]byte, error) { f, err := os.Open(filename) if err != nil { return nil, err } defer f.Close() r, err := charset.NewReader(f) if err != nil { return nil, err } defer r.Close() return ioutil.ReadAll(r) }
where The charset.NewReader
method will generate a new ReadCloser
object according to the specified encoding format, and use this object to read the file.
3.2. Use the unicode/utf16
library for byte order conversion
When using the unicode/utf16
library, you need to pay attention to the maximum size in the library The length is 32767 bytes, if the file size exceeds this limit, segmented reading is required.
Code example:
package main import ( "fmt" "io/ioutil" "unicode/utf16" ) func readUTF16File(filename string) ([]byte, error) { data, err := ioutil.ReadFile(filename) if err != nil { return nil, err } u := utf16.Decode(data) return []byte(string(u)), nil } func main() { data, _ := readUTF16File("test.txt") fmt.Println(string(data)) }
3.3. Use golang.org/x/text
library for encoding format conversion
golang.org/ The x/text
library provides a very detailed encoding format conversion function that can solve most problems related to encoding formats.
Code example:
package main import( "fmt" "io/ioutil" "golang.org/x/text/encoding/charmap" ) func ReadFileWithCharset(filename string, charset string) ([]byte, error) { data, err := ioutil.ReadFile(filename) if err != nil { return nil, err } charmap := charmap.Windows1252.NewDecoder() return charmap.Bytes(data) } func main() { data,_:=ReadFileWithCharset("test.txt","UTF-8") fmt.Println(string(data)) }
4. Summary
The problem of garbled characters is a very common problem in development, and in golang, solutions should be chosen according to the specific situation. If the file encoding format is determined, the corresponding encoding format should be specified during the file reading process; if byte order issues are involved, you need to use the unicode/utf16
library for byte order conversion; for other For encoding format conversion issues, you can use the golang.org/x/text
library for conversion. Through the above methods, the problem of reading garbled characters in golang files can be effectively solved and development efficiency improved.
The above is the detailed content of What should I do if golang reads garbled files?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
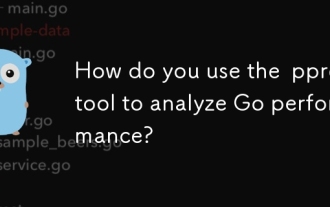
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
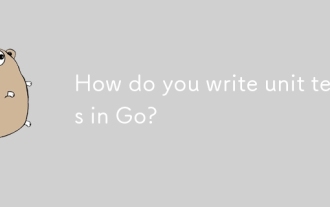
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
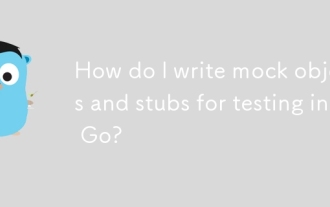
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
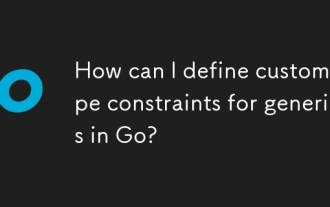
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
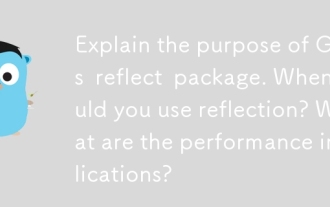
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
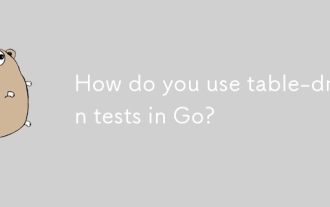
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
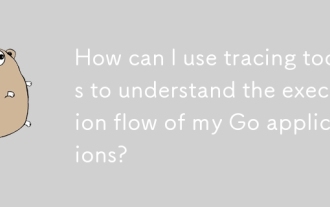
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
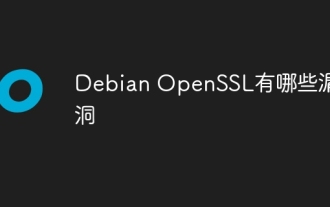
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
