How to query mongo in golang
Golang is an open source programming language that is widely used in cloud computing, networks, distributed systems and other fields. MongoDB is a high-performance, scalable, document storage-oriented database that is favored by many developers. This article will introduce how to use Golang to query the MongoDB database.
1. Install the database driver
Before using Golang to connect to MongoDB, you need to install the Go language driver of MongoDB. It can be installed by executing the following command:
go get gopkg.in/mgo.v2
The name of this Go language driver is "mgo", which allows us to pass Go programs operate MongoDB databases.
2. Connect to MongoDB database
Before starting to query MongoDB, you need to establish a connection with the MongoDB database. This can be achieved by the following code:
package main import ( "fmt" "gopkg.in/mgo.v2" ) func main() { session, err := mgo.Dial("mongodb://localhost:27017") if err != nil { panic(err) } defer session.Close() // ... }
In this code, we use the "Dial" method in the "mgo" package to connect to the MongoDB database. When calling, we need to pass it the address of the MongoDB database. In actual applications, the correct MongoDB database address needs to be passed to it.
3. Query the MongoDB database
After you have a session connected to MongoDB, you can perform query operations. In the example below, we will get all the documents from the collection named "testdb".
package main import ( "fmt" "gopkg.in/mgo.v2" "gopkg.in/mgo.v2/bson" ) type Person struct { Name string Phone string } func main() { session, err := mgo.Dial("mongodb://localhost:27017") if err != nil { panic(err) } defer session.Close() // 获取指定数据库的集合 c := session.DB("test").C("testdb") // 查询集合中的所有文档 var result []Person err = c.Find(bson.M{}).All(&result) if err != nil { panic(err) } // 输出结果 for _, v := range result { fmt.Println("Name:", v.Name, "Phone:", v.Phone) } }
In this example, we create a structure named "Person". The "Name" and "Phone" fields of this structure are used to store data in the document. Then we will perform query operations through the "Find" and "All" methods in the "mgo" package. When performing a query operation, we use the "bson.M" function to create an empty bson object so that we can use it in the query. We then store the results in a variable of type "[]Person" and iterate over the results and output the results.
4. Conditional query
In actual queries, we usually need to filter the documents in the collection based on conditions. Here is an example query for information about a person named "Joe".
// 查询名字为“Joe”的人的信息 err = c.Find(bson.M{"Name": "Joe"}).All(&result) if err != nil { panic(err) } // 输出结果 for _, v := range result { fmt.Println("Name:", v.Name, "Phone:", v.Phone) }
In this example, we complete the query operation by passing a bson.M containing the "Name" field value of "Joe" as the query condition.
The following is an example to query the information of everyone whose phone number contains "123".
// 查询电话号码包含“123”的人的信息 err = c.Find(bson.M{"Phone": bson.M{"$regex": "123"}}).All(&result) if err != nil { panic(err) } // 输出结果 for _, v := range result { fmt.Println("Name:", v.Name, "Phone:", v.Phone) }
In this example, we complete the query operation by passing a bson.M containing the "Phone" field value "$regex:123" as the query condition. Among them, "$regex" is a special character representing a regular expression, which is used to match people whose phone numbers contain "123".
Summary
This article introduces how to use Golang to connect to MongoDB, and demonstrates how to perform simple query operations through some examples. In practical applications, the method of querying MongoDB can be adjusted according to specific needs. At the same time, Golang's excellent performance and simple-to-use syntax also provide a better choice for operating MongoDB databases.
The above is the detailed content of How to query mongo in golang. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


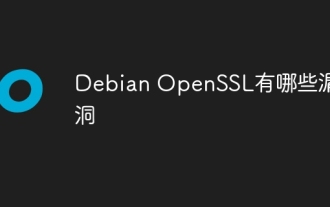
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
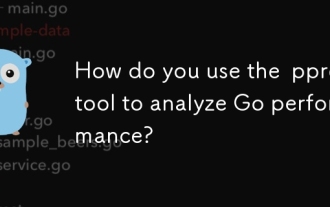
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
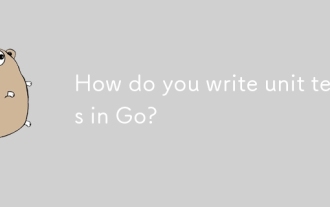
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
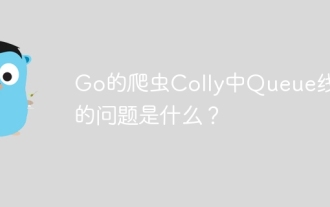
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
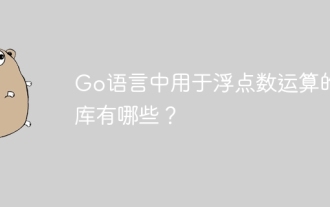
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
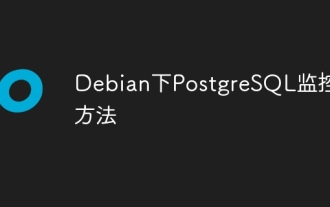
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
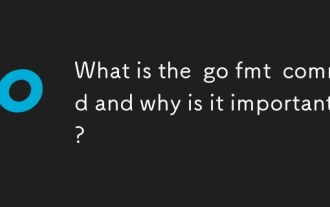
The article discusses the go fmt command in Go programming, which formats code to adhere to official style guidelines. It highlights the importance of go fmt for maintaining code consistency, readability, and reducing style debates. Best practices fo
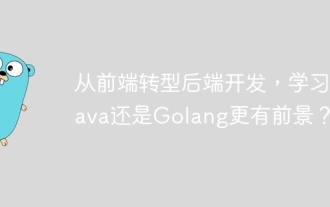
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
