How to remove specified subscript array elements in php
In PHP, an array is a very commonly used data type that can store multiple values and access each value through a unique key. Sometimes we need to remove an element at a specified index from an array because it may not be needed later in the code, or it may be a wrong value. This article will introduce how to remove array elements with specified subscripts in PHP.
First, we need to know how to create and access arrays in PHP. There are many ways to create an array, such as using the array() function or the [] method. Here is some sample code:
// 使用 array() 函数创建一个数组 $my_array = array('apple', 'banana', 'orange'); // 使用 [] 方式创建一个数组 $my_array = ['apple', 'banana', 'orange']; // 访问数组中的元素 echo $my_array[0]; // 输出 'apple' echo $my_array[1]; // 输出 'banana' echo $my_array[2]; // 输出 'orange'
Now assume we want to remove the element with index 1 ('banana'). PHP provides some built-in functions for this purpose.
- unset()
In PHP, the unset() function is used to destroy variables. When the unset() function is applied to an array element, it removes the element from the array. The following is an example of using the unset() function to delete the element with the specified subscript:
$my_array = array('apple', 'banana', 'orange'); unset($my_array[1]); // 删除下标为 1 的元素 // 输出数组中的元素 echo $my_array[0]; // 输出 'apple' echo $my_array[1]; // 输出 'orange'
In the above code, we use the unset() function to delete the element with the subscript 1 in the array ('banana' ). When we access the array again, we can see that only 'apple' and 'orange' are left in the output.
- array_splice()
array_splice() function is another method for removing array elements. This function deletes one or more array elements and returns the deleted elements. This function requires three parameters: the array, the index to start deleting, and the number of elements to delete. The following is an example of using the array_splice() function to delete the element with the specified subscript:
$my_array = array('apple', 'banana', 'orange'); // 从下标为 1 的位置开始删除 1 个元素 $removed_element = array_splice($my_array, 1, 1); // 输出被删除的元素 echo $removed_element[0]; // 输出 'banana' // 输出数组中的元素 echo $my_array[0]; // 输出 'apple' echo $my_array[1]; // 输出 'orange'
In the above code, we use the array_splice() function to delete the element with the subscript 1 ('banana' ). This function returns an array containing the deleted elements. When we access the array again, we can see that only 'apple' and 'orange' are left in the output.
It should be noted that using the unset() function can only delete the element with the specified subscript, but it cannot move all elements following the element forward. The array_splice() function can be used to delete the element at the specified subscript and move all elements following the element forward.
In actual development, we need to choose which method to use based on the specific situation. If you need to rearrange the array, it is best to use the array_splice() function, otherwise use the unset() function.
This article introduces how to remove array elements with specified subscripts in PHP, including the unset() function and array_splice() function. Use these methods to easily remove specified elements from an array and rearrange the array. During the development process, we need to choose the appropriate method according to the specific situation.
The above is the detailed content of How to remove specified subscript array elements in php. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
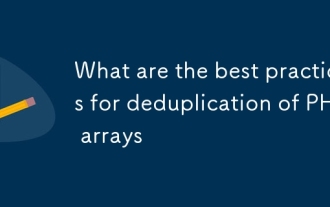
This article explores efficient PHP array deduplication. It compares built-in functions like array_unique() with custom hashmap approaches, highlighting performance trade-offs based on array size and data type. The optimal method depends on profili
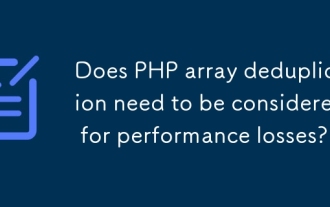
This article analyzes PHP array deduplication, highlighting performance bottlenecks of naive approaches (O(n²)). It explores efficient alternatives using array_unique() with custom functions, SplObjectStorage, and HashSet implementations, achieving
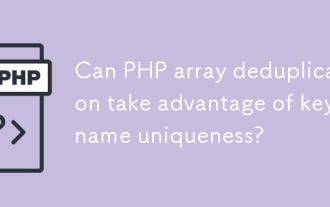
This article explores PHP array deduplication using key uniqueness. While not a direct duplicate removal method, leveraging key uniqueness allows for creating a new array with unique values by mapping values to keys, overwriting duplicates. This ap
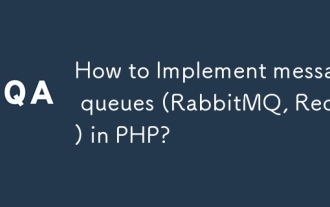
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
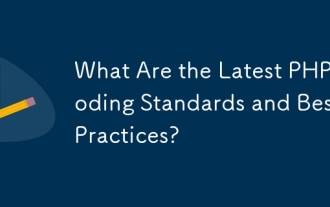
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
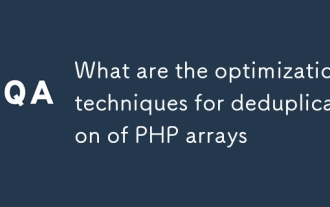
This article explores optimizing PHP array deduplication for large datasets. It examines techniques like array_unique(), array_flip(), SplObjectStorage, and pre-sorting, comparing their efficiency. For massive datasets, it suggests chunking, datab
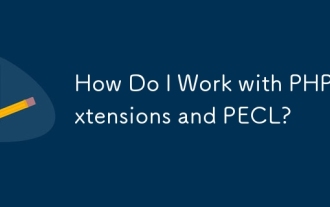
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
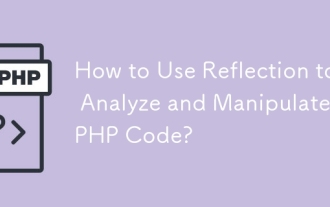
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
