How to convert data type to integer in Javascript
Javascript is an object-oriented programming language that provides a variety of data types, including integers, floating point numbers, strings, Boolean values, and more. In Javascript, use the parseInt() function to convert a string into an integer, and use the Math.floor() function to convert a floating point number into an integer. This article will introduce how to use these two functions to convert Javascript data types into integers.
1. The parseInt() function converts a string into an integer
The parseInt() function can convert a string containing an integer into an integer. For example, the following code converts the string "123" into the integer 123:
let a = "123"; let b = parseInt(a); console.log(b); // 输出 123
This function can also specify a base number for parsing hexadecimal or octal numbers. For example, the following code converts the binary string "1010" to the decimal integer 10:
let a = "1010"; let b = parseInt(a, 2); console.log(b); // 输出 10
If the string cannot be parsed as a number, NaN is returned. For example, the string "hello" in the following code cannot be parsed as a number, so NaN is returned:
let a = "hello"; let b = parseInt(a); console.log(b); // 输出 NaN
It should be noted that if the string starts with 0, it will be judged as an octal number. For example, the string "012" in the following code is parsed as a decimal integer 10:
let a = "012"; let b = parseInt(a); console.log(b); // 输出 10
To avoid this situation, you can specify the decimal number 10 in the parseInt() function:
let a = "012"; let b = parseInt(a, 10); console.log(b); // 输出 12
2. The Math.floor() function converts floating-point numbers into integers
The Math.floor() function can round down floating-point numbers and convert them into integers. For example, the following code converts the floating point number 1.23 to the integer 1:
let a = 1.23; let b = Math.floor(a); console.log(b); // 输出 1
If the parameter is NaN, NaN is returned:
let a = NaN; let b = Math.floor(a); console.log(b); // 输出 NaN
It should be noted that if the parameter is positive infinity or negative infinity, then Return infinity or negative infinity:
let a = Infinity; let b = Math.floor(a); console.log(b); // 输出 Infinity
3. Summary
This article introduces how to use the parseInt() function and Math.floor() function to convert Javascript data types into integers. When using the parseInt() function, you need to pay attention to the issue of base numbers; when using the Math.floor() function, you need to pay attention to the parameter value range (cannot be NaN or infinity). Correct use of these two functions can help us better process data and write efficient Javascript code.
The above is the detailed content of How to convert data type to integer in Javascript. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


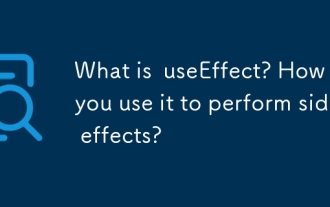
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.
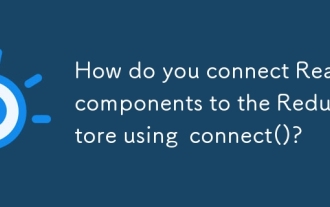
Article discusses connecting React components to Redux store using connect(), explaining mapStateToProps, mapDispatchToProps, and performance impacts.
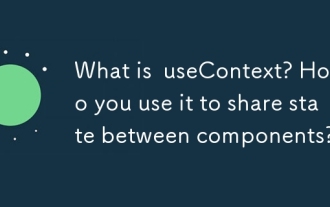
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
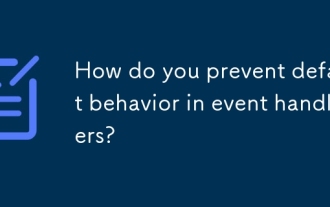
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
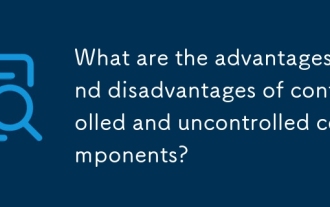
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.

React combines JSX and HTML to improve user experience. 1) JSX embeds HTML to make development more intuitive. 2) The virtual DOM mechanism optimizes performance and reduces DOM operations. 3) Component-based management UI to improve maintainability. 4) State management and event processing enhance interactivity.
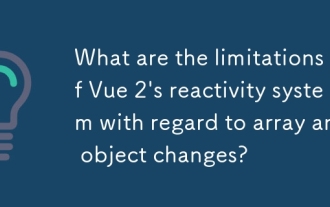
Vue 2's reactivity system struggles with direct array index setting, length modification, and object property addition/deletion. Developers can use Vue's mutation methods and Vue.set() to ensure reactivity.
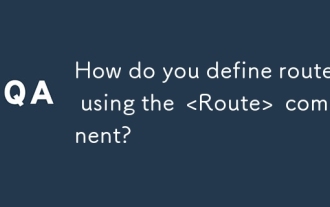
The article discusses defining routes in React Router using the <Route> component, covering props like path, component, render, children, exact, and nested routing.
