How to use Ajax and JavaScript in JSP to achieve cascading effects
With the rapid development of Web applications, front-end technology is becoming more and more important. In this article, we'll take a deep dive into how to use Ajax and JavaScript in JSP to achieve cascading effects.
1. What is cascade?
Cascading means that after selecting an option in one drop-down list, the options in another drop-down list will also change accordingly. For example, if you select Beijing in the "Province" drop-down list, the options in the "City" drop-down list will automatically change to the city to which Beijing belongs.
2. Ajax and JavaScript in JSP
In JSP, we can use Ajax and JavaScript to interact with the interface. Among them, Ajax is the abbreviation of Asynchronous JavaScript and XML (asynchronous JavaScript and XML). It uses JavaScript and XML technologies to update a portion of a page without reloading the entire page. JavaScript is a scripting language that can be run in the browser. It can operate HTML pages and achieve dynamic effects on web pages.
3. How to realize cascading?
- Front-end part
In the front-end, we need to define the code for the two drop-down lists. For example, we define a "Province" and a "City" drop-down list:
<label for="province">省份</label> <select id="province"> <option value="0">请选择</option> <option value="1">北京市</option> <option value="2">上海市</option> <option value="3">广东省</option> </select> <label for="city">城市</label> <select id="city"> <option value="0">请选择</option> </select>
Here we use the id attribute to name the drop-down lists so that they can be operated in JavaScript.
- Backend part
In the background, we need to define an interface to obtain a city list. For example, we can use the Spring MVC framework to implement this function:
@RestController @RequestMapping("/city") public class CityController { @GetMapping("/{provinceId}") public List<City> getCityList(@PathVariable int provinceId) { List<City> cityList = null; // 查询城市列表的代码 return cityList; } @Data public static class City { private int id; private String name; } }
Here we define a CityController class and obtain and return the city list in its getCityList method. Note that we also define an inner class City to represent city information.
- JavaScript part
In JavaScript, we need to perform a series of operations, including:
- Listen to the selection of the "Province" drop-down list Event
- Send Ajax request to the background to get the city list
- Dynamicly update the contents of the "City" drop-down list based on the city list
For example, we can use jQuery Library to implement this function:
$(document).ready(function () { $("#province").change(function () { var provinceId = $(this).val(); if (provinceId > 0) { $.get("/city/" + provinceId, function (data) { var citySelect = $("#city"); citySelect.empty().append('<option value="0">请选择</option>'); $.each(data, function (index, city) { citySelect.append('<option value="' + city.id + '">' + city.name + '</option>'); }); }); } else { $("#city").empty(); } }); });
In this JavaScript code, we define a ready method to be executed after the page is loaded. Then, we listened to the selection event of the "Province" drop-down list and obtained its value. If the value is greater than 0, it means that the user has selected a valid province, and we use the $.get method to send an Ajax request to the background to obtain the corresponding city list. After successfully obtaining the city list, we dynamically updated the contents of the "City" drop-down list. Otherwise, if the user selects "Please select", we clear the "City" drop-down list.
4. Summary
This article deeply explores the method of using Ajax and JavaScript in JSP to achieve cascading effects. By defining drop-down lists on the front end, defining interfaces on the backend, and invoking JavaScript events and Ajax methods, we can easily achieve cascading effects, improve user experience, and make web applications more convenient and efficient.
The above is the detailed content of How to use Ajax and JavaScript in JSP to achieve cascading effects. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


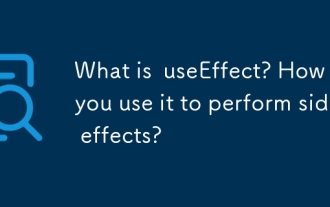
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.
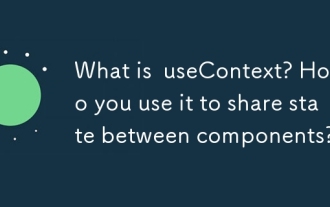
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
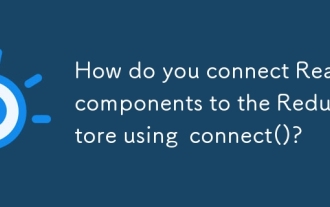
Article discusses connecting React components to Redux store using connect(), explaining mapStateToProps, mapDispatchToProps, and performance impacts.
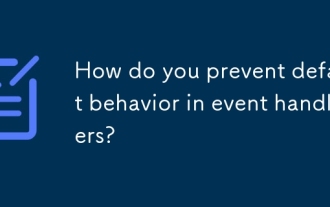
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.

React combines JSX and HTML to improve user experience. 1) JSX embeds HTML to make development more intuitive. 2) The virtual DOM mechanism optimizes performance and reduces DOM operations. 3) Component-based management UI to improve maintainability. 4) State management and event processing enhance interactivity.
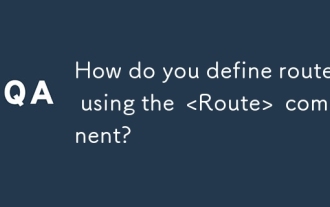
The article discusses defining routes in React Router using the <Route> component, covering props like path, component, render, children, exact, and nested routing.
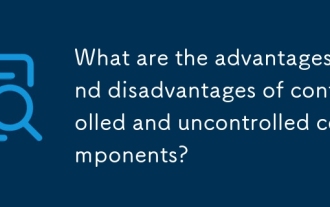
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.
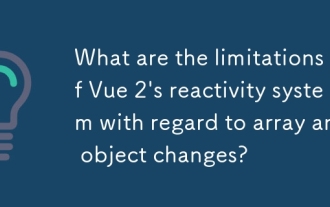
Vue 2's reactivity system struggles with direct array index setting, length modification, and object property addition/deletion. Developers can use Vue's mutation methods and Vue.set() to ensure reactivity.
