How to modify data in multiple tables in PHP
PHP is a popular programming language that can be used with a variety of database management systems. When we need to modify data in multiple tables, using PHP can greatly simplify the process. This article will introduce how to modify the data of multiple tables in PHP.
- Connect to the database
First, we need to connect to the database in the PHP code. Assuming we are using a MySQL database, we can use the following code to connect to the database:
<?php $servername = "localhost"; $username = "username"; $password = "password"; $dbname = "myDB"; // 创建连接 $conn = mysqli_connect($servername, $username, $password, $dbname); // 检查连接 if (!$conn) { die("连接失败: " . mysqli_connect_error()); } echo "连接成功"; ?>
- Preparing SQL query statements
Next, we need to prepare the SQL query to be executed statement. Suppose we want to modify data in two tables, such as the user table and the orders table. We can use the following SQL statement:
UPDATE users, orders SET users.name = 'New Name', orders.price = 50 WHERE users.id = 1 AND orders.user_id = 1;
This SQL statement will modify the name and price fields of the qualified data in the users table and orders table to "New Name" and 50.
- Execute SQL query statement
Once we have prepared the SQL query statement, we can execute it in the PHP code. We can use the mysqli_query function to execute the query statement, as shown below:
$sql = "UPDATE users, orders SET users.name = 'New Name', orders.price = 50 WHERE users.id = 1 AND orders.user_id = 1"; if (mysqli_query($conn, $sql)) { echo "数据已更新"; } else { echo "更新数据时出错: " . mysqli_error($conn); }
This code block will execute our prepared SQL query statement and output the corresponding information.
- Full PHP code
The following is the complete PHP code for modifying data in multiple tables:
<?php $servername = "localhost"; $username = "username"; $password = "password"; $dbname = "myDB"; // 创建连接 $conn = mysqli_connect($servername, $username, $password, $dbname); // 检查连接 if (!$conn) { die("连接失败: " . mysqli_connect_error()); } echo "连接成功"; // 准备SQL查询语句 $sql = "UPDATE users, orders SET users.name = 'New Name', orders.price = 50 WHERE users.id = 1 AND orders.user_id = 1"; // 执行SQL查询语句 if (mysqli_query($conn, $sql)) { echo "数据已更新"; } else { echo "更新数据时出错: " . mysqli_error($conn); } // 关闭连接 mysqli_close($conn); ?>
- Summary
Modifying data in multiple tables in PHP requires the following steps:
- Connect to the database
- Prepare SQL query statements
- Execute SQL query statement
- Close the connection
The above steps can simplify the operation and allow us to easily modify data in multiple tables.
The above is the detailed content of How to modify data in multiple tables in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


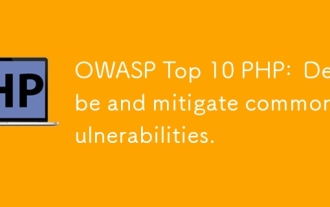
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
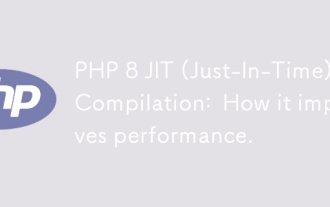
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
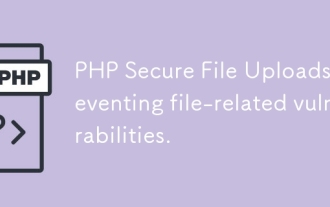
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
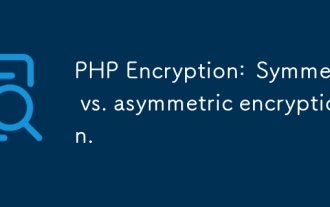
The article discusses symmetric and asymmetric encryption in PHP, comparing their suitability, performance, and security differences. Symmetric encryption is faster and suited for bulk data, while asymmetric is used for secure key exchange.

The article discusses implementing robust authentication and authorization in PHP to prevent unauthorized access, detailing best practices and recommending security-enhancing tools.
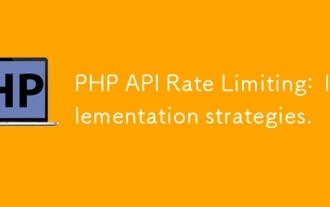
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
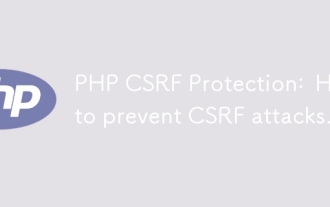
The article discusses strategies to prevent CSRF attacks in PHP, including using CSRF tokens, Same-Site cookies, and proper session management.

Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
