How to use mgo to query MongoDB in golang
In Golang, querying MongoDB is a very important task. mgo is a very popular MongoDB driver that provides a rich API for CRUD operations. This article will introduce how to use mgo to query MongoDB.
Install mgo
To use mgo, you first need to install it. It can be installed using the go get command. Open a terminal and run the following command:
go get gopkg.in/mgo.v2
This will download mgo from the Github repository and add it to the vendor directory in the Golang project.
Import mgo package
When using mgo in code, you need to import its package. You can write the following code at the top of the file to import the mgo package and create an alias for it.
import (
"gopkg.in/mgo.v2" "gopkg.in/mgo.v2/bson"
)
Querying documents in a collection
Querying MongoDB starts with querying documents in a collection. Using the Find() method of mgo.Collection, you can query the documents in the collection. Here are some basic query examples.
- Query all documents
If you don’t want to add any conditional filtering, you can use the Find() method to return all documents in the collection.
func getAllEmployees() ([]Employee, error) {
session := GetSession() defer session.Close() collection := session.DB("company").C("employees") var employees []Employee err := collection.Find(nil).All(&employees) return employees, err
}
In the above code, the session.DB() method is used to open a database, and select the collection to be operated on. The Find() method returns a cursor that can be used to retrieve all documents that meet the criteria. The All() method reads all documents into the specified slice and returns an error.
- Conditional filtering
You can filter the returned documents by passing a conditional document. The following is an example of querying employees whose salary is greater than 1000:
func getEmployeesWithSalaryGreaterThan1000() ([]Employee, error) {
session := GetSession() defer session.Close() collection := session.DB("company").C("employees") var employees []Employee err := collection.Find(bson.M{"salary": bson.M{"$gt": 1000}}).All(&employees) return employees, err
}
In the above code, bson.M represents a document in MongoDB. The generated condition document is
{
"salary": { "$gt": 1000 }
}
The above conditions will filter out all employees with a salary greater than $1,000. In the Find() method, pass the conditional document as a parameter. The $gt operator means greater than.
- Return only specific fields
Using the Select() method, you can specify the fields to be returned. As shown below:
func getEmployeeNameAndAge() ([]Employee, error) {
session := GetSession() defer session.Close() collection := session.DB("company").C("employees") var employees []Employee err := collection.Find(nil).Select(bson.M{"name": 1, "age": 1}).All(&employees) return employees, err
}
In the above code, the Select() method is used to select the The fields returned. In this example, the name and age are returned.
Note: At least one field must be set to 1. If all fields are set to 0, the query will return an empty document.
- Paging
Use the Skip() and Limit() methods to paginate the results. As shown below:
func getPaginatedEmployees(page int, limit int) ([]Employee, error) {
session := GetSession() defer session.Close() collection := session.DB("company").C("employees") var employees []Employee err := collection.Find(nil).Skip((page - 1) * limit).Limit(limit).All(&employees) return employees, err
}
In the above code, Skip() method is used to skip a specified number of documents, and the Limit() method specifies the number of documents to be returned.
Summary
This article introduces how to use mgo to query MongoDB in Golang. Query the documents in the collection by using the Find() method, and limit the documents returned by passing filtering and paging options. Use the Select() method to return only specific fields. mgo also provides other query methods and options that can be used as needed.
The above is the detailed content of How to use mgo to query MongoDB in golang. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
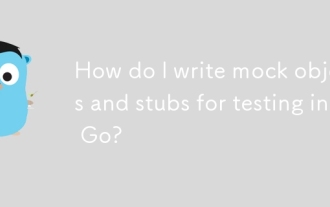
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
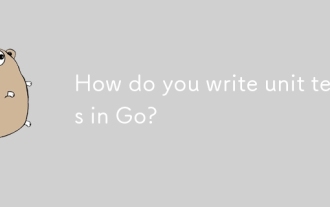
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
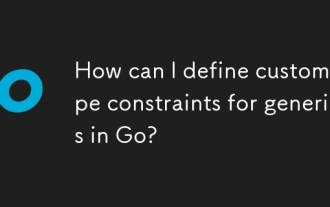
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
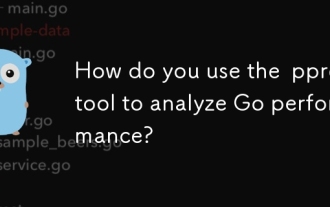
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
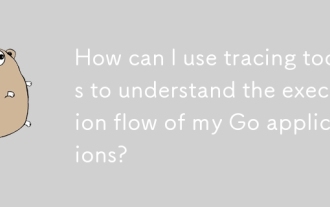
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
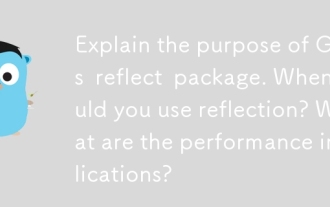
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
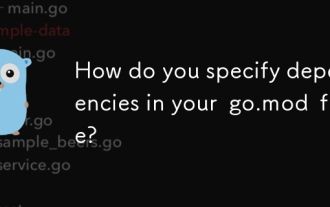
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
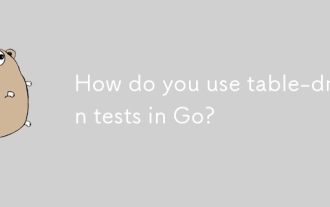
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
