How to convert string to xml format in javascript
JavaScript is a scripting language widely used in front-end web page development. In web development, we usually need to send data to the server in XML format, or receive XML data from the server and parse it. In such cases, it is very important to convert the JavaScript string to XML format.
In this article, we will take an in-depth look at how to convert a string into XML format using JavaScript. This will help web developers write more efficient XML code and process web application data quickly.
First step, understand the XML format
Before converting strings to XML, we need to understand what XML is and its basic structure. XML is Extensible Markup Language (XML), used to describe the structure and content of data. XML can be used for data transmission and data storage, and can also be used to describe application configuration information.
XML consists of markup and text. Tags are XML elements used to describe data. Text and other tags can be included between tags. Each XML element consists of a start tag and an end tag. The start tag contains the element name and attributes, and the end tag only contains the element name. The following is an example of an XML element:
<book id="1234"> <title>JavaScript 字符串转换XML</title> <author>John Doe</author> <price>29.99</price> </book>
In the above example, we can see that the <book>
element is an XML element that contains text and other markup. It also has an attribute named "id" which has the value "1234". In the closing tag, we only see the tag name and nothing else.
Second step, use JavaScript to create an XML string
With an understanding of XML, now we can start converting the string into XML format. In JavaScript, we can use string manipulation and DOM manipulation to create XML strings.
We can use string concatenation to create XML strings, for example:
let xmlStr = '<book id="1234">'; xmlStr += '<title>JavaScript 字符串转换XML</title>'; xmlStr += '<author>John Doe</author>'; xmlStr += '<price>29.99</price>'; xmlStr += '</book>';
In the above example, we use the plus sign to concatenate multiple strings and save them to variables xmlStr
in. After all the necessary XML elements and attributes are added, we add the </book>
tag as the closing tag.
DOM manipulation is also a way to create XML strings. We can use the JavaScript DOM API to create XML nodes and build XML structures by adding nodes to the DOM tree. For example, in the following code snippet, we create an XML element and add it to the XML document:
let xmlDoc = document.implementation.createDocument("", "", null); let bookElem = xmlDoc.createElement("book"); let titleElem = xmlDoc.createElement("title"); let titleText = xmlDoc.createTextNode("JavaScript 字符串转换 XML"); titleElem.appendChild(titleText); bookElem.appendChild(titleElem); xmlDoc.appendChild(bookElem);
In the above code snippet, we first use the createDocument()
method Create an XML document object, and then use the createElement()
method to create an XML element named "book". Next, we create another element called "title" within the XML element and add text to that element by using the createTextNode()
method. Finally, we add the "title" element to the XML document object.
The third step is to parse the string into XML
When sending XML data to the server or receiving XML data from the server, we need to convert the string into an XML object for easy parsing. and operations. In JavaScript, we can use the DOMParser
object to parse a string into an XML document.
For example, in the following code snippet, we parse a string named xmlStr
into an XML document:
let xmlDoc; if (window.DOMParser) { let parser = new DOMParser(); xmlDoc = parser.parseFromString(xmlStr, "text/xml"); } else { xmlDoc = new ActiveXObject("Microsoft.XMLDOM"); xmlDoc.loadXML(xmlStr); }
In the above code snippet, we first Check if the browser supports DOMParser object. If supported, the xmlStr
string is parsed into an XML document using the parseFromString()
method. Otherwise, we use ActiveXObject objects (only available in Internet Explorer browsers) to parse the XML string.
The fourth step, XML data parsing
Once we parse the XML string into an XML document object, we can start parsing and manipulating the XML data. In JavaScript, we can use the getElementsByTagName()
method to access XML elements, and the getAttribute()
method to access the attributes of XML elements. For example, in the following code snippet, we use the XML document object to get the <book>
element:
let bookElem = xmlDoc.getElementsByTagName("book");
We can then get the # using the getAttribute()
method ##<book>The value of the "id" attribute of the element:
let bookId = bookElem[0].getAttribute("id");
<book> element and put They are saved to the variable
bookElem. We then get the "id" attribute of the first instance of the
bookElem element and save it into the variable
bookId.
The above is the detailed content of How to convert string to xml format in javascript. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


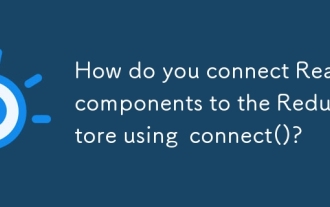
Article discusses connecting React components to Redux store using connect(), explaining mapStateToProps, mapDispatchToProps, and performance impacts.

React combines JSX and HTML to improve user experience. 1) JSX embeds HTML to make development more intuitive. 2) The virtual DOM mechanism optimizes performance and reduces DOM operations. 3) Component-based management UI to improve maintainability. 4) State management and event processing enhance interactivity.
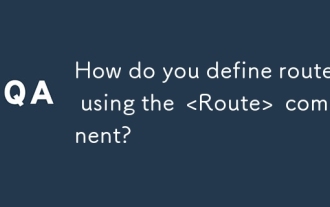
The article discusses defining routes in React Router using the <Route> component, covering props like path, component, render, children, exact, and nested routing.
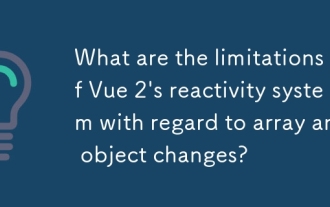
Vue 2's reactivity system struggles with direct array index setting, length modification, and object property addition/deletion. Developers can use Vue's mutation methods and Vue.set() to ensure reactivity.
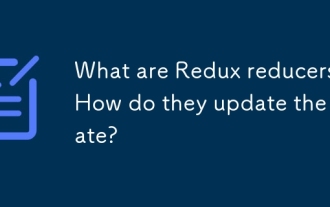
Redux reducers are pure functions that update the application's state based on actions, ensuring predictability and immutability.
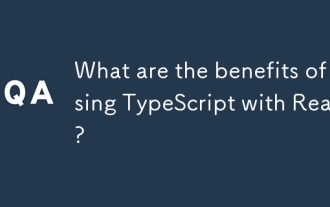
TypeScript enhances React development by providing type safety, improving code quality, and offering better IDE support, thus reducing errors and improving maintainability.
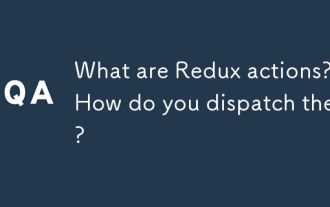
The article discusses Redux actions, their structure, and dispatching methods, including asynchronous actions using Redux Thunk. It emphasizes best practices for managing action types to maintain scalable and maintainable applications.
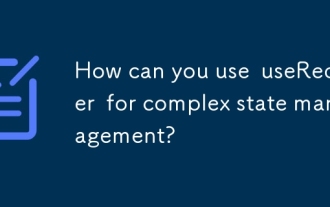
The article explains using useReducer for complex state management in React, detailing its benefits over useState and how to integrate it with useEffect for side effects.
