Why nodejs cannot inherit
In recent years, Node.js (hereinafter referred to as Node) has become one of the most popular server-side JavaScript platforms in the world. Although it has many features, whether it is running speed, memory footprint or supported libraries and frameworks, Node still has its own limitations in some aspects. One of the most prominent problems is that Node.js cannot be inherited.
In object-oriented programming, inheritance is an important feature that allows developers to create a base class (parent class) and create other subclasses based on it. These subclasses can override methods in the parent class or add new methods to extend and reuse functionality. In the context of Node.js, inheritance usually takes the form of copying properties and methods between classes. However, due to the asynchronous model of Node.js and its interplay with the flexibility of JavaScript, inheritance is not very intuitive in Node and is sometimes difficult to implement. This article will explore why Node.js cannot inherit and some alternatives.
1. The problem of implementing inheritance in Node.js
There are two key factors in realizing the problem of inheritance in Node.js:
- Asynchronous programming model
One of the features of Node.js is its asynchronous programming model, which is closely related to JavaScript. Since Node.js is single-threaded, it must use an asynchronous model in order to handle multiple client requests and other asynchronous tasks. The asynchronous model makes Node.js more efficient, but conflicts with the inherited implementation. Because in asynchronous programming, function calls are made based on timers. After calling an asynchronous function, the program will continue running and will not return to the function until the asynchronous callback completes.
Consider the following piece of code:
class Person { constructor(name) { this.name = name; } greet() { console.log(`Hello, my name is ${this.name}.`); } } class User extends Person { constructor(name, email) { super(name); this.email = email; } static log(email) { console.log(`User ${email} logged in.`); } login() { User.log(this.email); this.greet(); } } const alice = new User('Alice', 'alice@example.com'); alice.login();
In this example, we have a class Person
and another class User
, which inherits from Person
. When we call the login
method on a User
instance, we will first call a log
method unique to the User
class, and then call the parent The greet
method of the class. But since the function is executed asynchronously, the result will not be as we expected:
User alice@example.com logged in. undefined
In fact, the calling order is incorrect. Since this.greet
is called based on asynchronous behavior, the execution of this method is performed after the callback of the asynchronous User.log
method. That's why undefined
is printed on the screen.
- Object-oriented JavaScript
The second problem is JavaScript’s own support for the object-oriented programming pattern. JavaScript is not a traditional object-oriented language and uses a prototype-based object model rather than a class-based object model. The prototype model does not directly support class inheritance like inheritance. If you want to implement inheritance in Node.js, you need to use some JavaScript object-oriented programming skills.
In the prototype-based inheritance model, developers need to use the Object.create()
method to create an object and pass it to subclasses as a prototype. The advantage of this inheritance pattern is that it is more flexible because it allows you to inherit not only the methods of the class, but also the properties.
The following is a prototype-based example:
class Person { constructor(name) { this.name = name; } greet() { console.log(`Hello, my name is ${this.name}.`); } } const User = Object.create(Person.prototype, { email: { value: null, enumerable: true, writable: true } }); User.constructor = User; User.constructor('Tom', 'tom@example.com'); console.log(User); User.greet();
In this example, we manually create an object and then point its prototype to Person.prototype
. When defining User
, we did not directly inherit Person
, but used the Object.create
method to create an object based on the parent class prototype. User registers the user through the User.constructor
function call.
2. Solution
Although Node.js has some limitations, there are some solutions to achieve inherited functions. Here we introduce some common solutions:
- Module-based inheritance
Node.js has a module-based architecture, and you can use this model to create modules that implement inheritance. . Node.js’s module system allows you to import functions, objects, and classes in different JavaScript files and combine them into a single module. The way to implement inheritance is to create a parent class module and a child class module, and use the functions, objects, and classes of the parent class module in the child class module.
Here is an example of inheriting the Person class
//person.js module.exports = class Person { constructor(name) { this.name = name; } greet() { console.log(`Hello, my name is ${this.name}.`); } }; //user.js const Person = require('./person'); class User extends Person { constructor(name, email) { super(name); this.email = email; } static log(email) { console.log(`User ${email} logged in.`); } login() { User.log(this.email); this.greet(); } } const user = new User('Tom', 'tom@example.com'); user.login();
In this example, we have a Person
module and a User
module. The latter inherits from the former. Note that we used require('./person')
in the User
module, imported the Person
module, and used extends Person
Inherit User
from Person
. Finally, we create a User
object and attempt to call the greet
method. The result this time was no problem.
- Use ES6 Proxy
Another way to solve the problem in Node.js is to use ES6 Proxy. Proxy is a new feature in JavaScript that allows us to run hook functions on objects or functions and change their behavior based on our requests.
Here is an example:
class Person { constructor(name) { this.name = name; } greet() { console.log(`Hello, my name is ${this.name}.`); } } class User extends Person { constructor(name, email) { super(name); this.email = email; this.proxy = new Proxy(this, { get(target, propKey) { return target[propKey] || target.__proto__[propKey]; }, }); } static log(email) { console.log(`User ${email} logged in.`); } login() { User.log(this.email); this.proxy.greet(); } } const user = new User('Tom', 'tom@example.com'); user.login();
在这个例子中,我们使用了一个新的属性 this.proxy
。该属性是使用 new Proxy()
创建一个新的 Proxy 实例,该实例包装了当前的对象。 我们在 get 钩子函数中进行了一些重点的操纵,当我们通过 this.proxy.greet()
调用 greet
方法时,它会在当前对象上执行搜索不到,然后自动查找其原型,并在原型上找到该方法。
三、总结
继承是面向对象编程中的一个重要特性。然而,在 Node.js 的环境中,由于其异步性和 JavaScript 本身的面向对象编程模型,继承并不像在传统面向对象语言中那样简单直观。但是,我们可以通过模块模式和 ES6 Proxy 等解决方案来实现继承,以实现更完整的面向对象编程体验。无论使用哪种方案,重要的是确保我们在开发过程中遵循最佳实践,以确保代码的可维护性和稳健性。
The above is the detailed content of Why nodejs cannot inherit. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


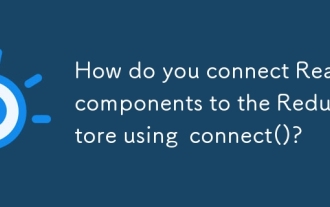
Article discusses connecting React components to Redux store using connect(), explaining mapStateToProps, mapDispatchToProps, and performance impacts.

React combines JSX and HTML to improve user experience. 1) JSX embeds HTML to make development more intuitive. 2) The virtual DOM mechanism optimizes performance and reduces DOM operations. 3) Component-based management UI to improve maintainability. 4) State management and event processing enhance interactivity.
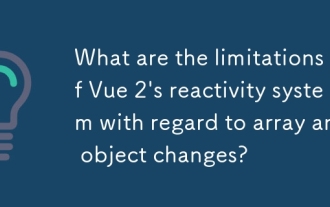
Vue 2's reactivity system struggles with direct array index setting, length modification, and object property addition/deletion. Developers can use Vue's mutation methods and Vue.set() to ensure reactivity.
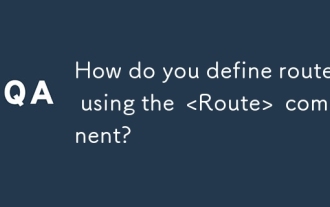
The article discusses defining routes in React Router using the <Route> component, covering props like path, component, render, children, exact, and nested routing.
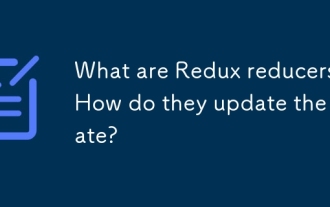
Redux reducers are pure functions that update the application's state based on actions, ensuring predictability and immutability.
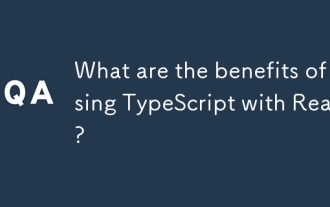
TypeScript enhances React development by providing type safety, improving code quality, and offering better IDE support, thus reducing errors and improving maintainability.
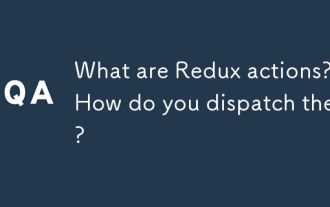
The article discusses Redux actions, their structure, and dispatching methods, including asynchronous actions using Redux Thunk. It emphasizes best practices for managing action types to maintain scalable and maintainable applications.
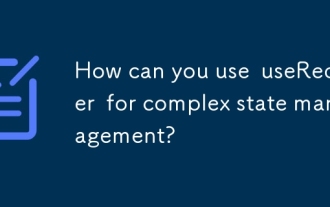
The article explains using useReducer for complex state management in React, detailing its benefits over useState and how to integrate it with useEffect for side effects.
