


How to determine whether there are the same values in an array in php
In PHP, we can use some functions to determine whether the same value exists in the array. This article will introduce several methods to determine whether the same value exists in an array.
Method 1: Use the in_array() function
In PHP, we can use the in_array() function to determine whether a value exists in the array. This function returns true if an array contains the same value.
The following is a sample code that uses the in_array() function to determine whether the same value exists in the array:
$array = array("apple", "banana", "orange", "apple"); if (in_array("apple", $array)) { echo "Array contains apple"; } else { echo "Array does not contain apple"; }
In the above example, we first define an array containing the same value, and then use The in_array() function determines whether the array contains the value "apple". If the array contains the value "apple", output "Array contains apple", otherwise output "Array does not contain apple".
Method 2: Use the array_count_values() function
Use the array_count_values() function to count the number of occurrences of each value in an array. If identical values exist in an array, the function returns an associative array containing the identical values and their number of occurrences.
The following is a sample code that uses the array_count_values() function to determine whether the same value exists in an array:
$array = array("apple", "banana", "orange", "apple"); $count = array_count_values($array); if (max($count) > 1) { echo "Array contains duplicate values"; } else { echo "Array does not contain duplicate values"; }
In the above example, we first define an array containing the same value, and then use The array_count_values() function counts the number of occurrences of each value in the array. If the maximum value is greater than 1, it means that the same value exists in the array, and "Array contains duplicate values" is output. Otherwise, "Array does not contain duplicate values" is output.
Method 3: Use the array_unique() function and count() function
We can also use the array_unique() function to remove duplicate values in the array, and use the count() function to count the length of the array. If the same value exists in an array, the length of the array after deduplication is different from the length of the original array.
The following is a sample code that uses the array_unique() function and count() function to determine whether the same value exists in the array:
$array = array("apple", "banana", "orange", "apple"); $unique = array_unique($array); if (count($array) == count($unique)) { echo "Array does not contain duplicate values"; } else { echo "Array contains duplicate values"; }
In the above example, we first define an array containing the same value array, then use the array_unique() function to remove duplicate values in the array, and use the count() function to count the length of the array. If the length after deduplication is the same as the length of the original array, it means that the same value does not exist in the array, and "Array does not contain duplicate values" is output. Otherwise, "Array contains duplicate values" is output.
Through the introduction of the above three methods, we can choose the appropriate method according to our needs to determine whether the same value exists in an array.
The above is the detailed content of How to determine whether there are the same values in an array in php. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


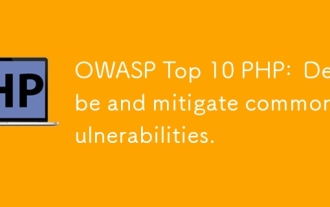
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
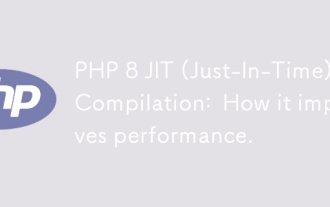
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
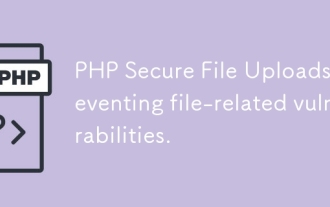
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
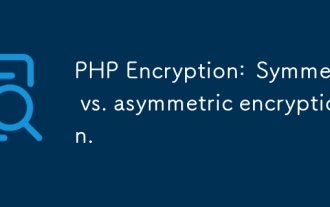
The article discusses symmetric and asymmetric encryption in PHP, comparing their suitability, performance, and security differences. Symmetric encryption is faster and suited for bulk data, while asymmetric is used for secure key exchange.

The article discusses implementing robust authentication and authorization in PHP to prevent unauthorized access, detailing best practices and recommending security-enhancing tools.
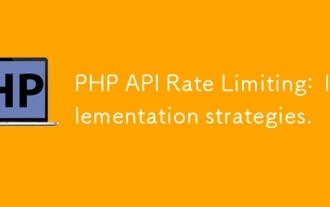
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
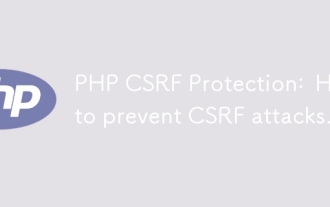
The article discusses strategies to prevent CSRF attacks in PHP, including using CSRF tokens, Same-Site cookies, and proper session management.

Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
