How to delete the head and tail of a php array
PHP is a popular server-side scripting language that is widely used in the development of websites and web applications. Arrays in PHP are a commonly used data structure that allow you to store and manipulate multiple values. In some cases, you may need to remove an element from the beginning or end of an array. This article will show you how to delete the first and last element of an array using PHP.
Delete the first element of the array
To delete the first element of the array, you can use the array_shift() function in PHP. This function will remove the first element from the array and will return the value of the removed element. If the array is empty, NULL is returned.
The following is an example that demonstrates how to use the array_shift() function to delete the first element from an array:
<?php $fruits = array("apple", "banana", "cherry"); echo "Original array: "; print_r($fruits); $removed_fruit = array_shift($fruits); echo "Removed fruit: " . $removed_fruit . "\n"; echo "Updated array: "; print_r($fruits); ?>
In the above example, we first define an array containing three fruit names array. We then remove the first element (i.e. "apple") using the array_shift() function and save it to the variable $removed_fruit. Finally, we print the updated array, which only contains the two fruits "banana" and "cherry".
Delete the last element of the array
To delete the last element of the array, you can use the array_pop() function in PHP. This function will remove the last element from the array and will return the value of the removed element. If the array is empty, NULL is returned.
Here is an example that demonstrates how to use the array_pop() function to remove the last element from an array:
<?php $fruits = array("apple", "banana", "cherry"); echo "Original array: "; print_r($fruits); $removed_fruit = array_pop($fruits); echo "Removed fruit: " . $removed_fruit . "\n"; echo "Updated array: "; print_r($fruits); ?>
In the above example, we first define an array containing three fruit names. array. We then remove the last element (i.e. "cherry") using the array_pop() function and save it to the variable $removed_fruit. Finally, we print the updated array, which only contains the two fruits "apple" and "banana".
Conclusion
In PHP, deleting the first and last elements of an array is relatively simple. You just need to use array_shift() or array_pop() function. These functions both delete elements and return the value of the deleted element. Understanding these functions can help you handle array operations more efficiently.
The above is the detailed content of How to delete the head and tail of a php array. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
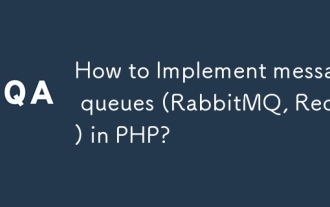
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
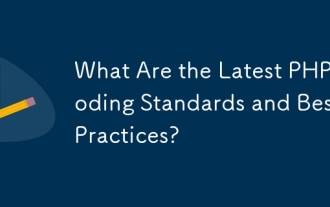
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
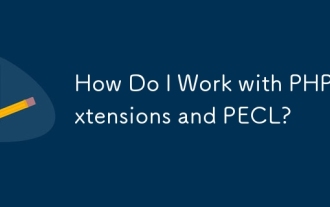
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
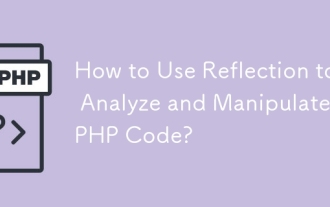
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
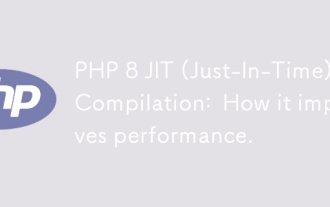
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
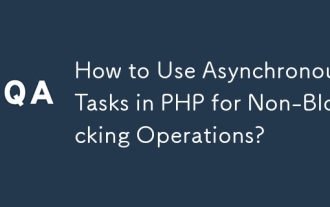
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
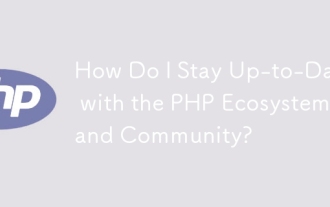
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
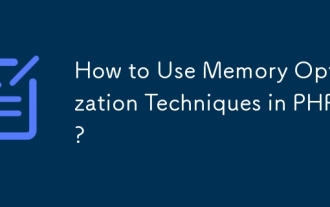
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v
