How to split an array into a string array in php
During the PHP development process, we often encounter the need to split an array into a string array. This requirement is very common in data processing and database operations, and can be easily achieved through PHP's built-in functions. In this article, we will introduce several ways to split an array into a string array in PHP.
1. Use the implode function
The implode function is PHP's built-in string function, which is used to splice the elements of the array into a string. The syntax of this function is as follows:
string implode ( string $glue , array $pieces )
Among them, $glue is the separator for splicing elements, and $pieces is the array that needs to be split.
The code for using the implode function to split an array into a string array is as follows:
$array = array('apple', 'banana', 'orange'); $string = implode(',', $array); $stringArray = explode(',', $string); print_r($stringArray);
The above code splices the array $array
through the implode
function into a string, and then use the explode
function to split the string into a string array using commas as delimiters, and finally print the string array. The output results are as follows:
Array ( [0] => apple [1] => banana [2] => orange )
2. Use json_encode and json_decode functions
json_encode
function can convert PHP objects or arrays into JSON format strings, and The json_decode
function can convert a JSON-formatted string into a PHP object or array. You can split an array into a string array by using json_encode
to convert the array into a JSON-formatted string, and then using json_decode
to convert the JSON-formatted string into a PHP array. The code is as follows:
$array = array('apple', 'banana', 'orange'); $json = json_encode($array); $stringArray = json_decode($json); print_r($stringArray);
The above code converts the array $array
into a string in JSON format through the json_encode
function, and then uses the json_decode
function to The string in JSON format is converted into a PHP array, and finally the string array is output and printed. The output result is as follows:
Array ( [0] => apple [1] => banana [2] => orange )
3. Use the array_map function
array_map
The function can apply a callback function to each element in an array, and the return value of the callback function as a new array. By defining a callback function to return the converted string form of the current element, and then applying the array_map
function, the array can be split into a string array. The code is as follows:
$array = array('apple', 'banana', 'orange'); $stringArray = array_map('strval', $array); print_r($stringArray);
The above code defines the callback function strval
, which is used to convert the current element into string form. Then apply the array_map
function to apply the callback function to each element, resulting in an array of strings. The output results are as follows:
Array ( [0] => apple [1] => banana [2] => orange )
Conclusion
By using the above three methods, we can easily split the PHP array into a string array and apply it in the actual development process. It should be noted that when using the implode
function and the json_encode
function, you can specify the delimiter for splicing elements and conversion format; when using the array_map
function, A callback function needs to be defined to convert elements, and the parameters and return value type of the callback function can be specified as needed. In actual development, you can choose the appropriate method according to your own needs.
The above is the detailed content of How to split an array into a string array in php. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


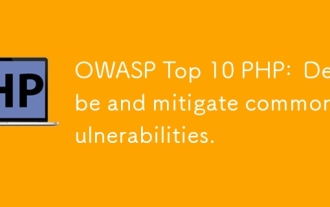
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
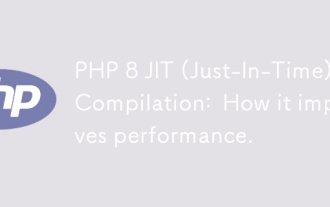
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
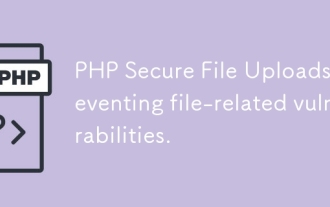
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
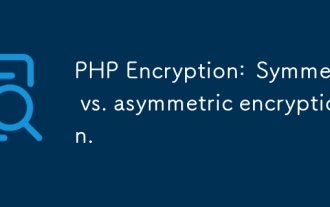
The article discusses symmetric and asymmetric encryption in PHP, comparing their suitability, performance, and security differences. Symmetric encryption is faster and suited for bulk data, while asymmetric is used for secure key exchange.

The article discusses implementing robust authentication and authorization in PHP to prevent unauthorized access, detailing best practices and recommending security-enhancing tools.
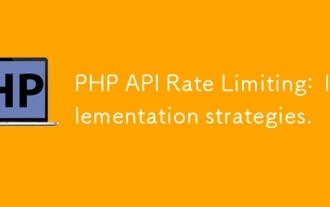
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
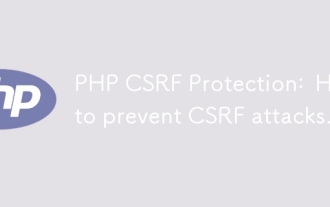
The article discusses strategies to prevent CSRF attacks in PHP, including using CSRF tokens, Same-Site cookies, and proper session management.

Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
