How to use Vue to implement login page jump output parameters
With the rapid development and popularization of front-end technology, more and more companies are beginning to apply Vue technology to build their own websites. In the application for corporate websites, login is a link that cannot be ignored, and Vue has its own unique method for realizing the login function. This article will introduce how to use Vue to implement login page jump output parameters, and then implement user login verification.
1. Preliminary preparation
Before we start to implement jump output parameters, we need to complete the following steps:
(1) Install Vue-CLI
Vue-CLI is Vue’s official scaffolding tool that simplifies configuration operations. Especially in terms of project creation, development and packaging construction, it can quickly get you into Vue development.
Installation method:
npm install -g @vue/cli
(2) Create a Vue project
We can create it using the command line in the terminal Vue project, enter the project root directory, use the command line to execute the following statement:
vue create my-project
2. Implement logic
1) Login page
In the login page, we need to enter the username and password for verification and jump display.
After the Vue project is created, we need to import the Vue plug-in vue-router to implement page jumps.
First, we enter the src directory of the project, create a new login.vue file, and perform the login operation on the page.
<div> <label>用户名</label> <input type="text" v-model="username"/> <label>密码</label> <input type="password" v-model="password"/> <button @click="submit">登录</button> </div>
<script><br>import { mapActions } from "vuex";<br>export default {</p> <div class="code" style="position:relative; padding:0px; margin:0px;"><pre class="brush:php;toolbar:false">data() { return { username: '', password: '' } }, methods: { ...mapActions(['login']), // 登录 async submit() { await this.login({ username: this.username, password: this.password }); } }</pre><div class="contentsignin">Copy after login</div></div> <p>}<br></script>
2) Establish a server interface for website background operations
We need to provide a RESTful API interface on the server side to process login requests and return the corresponding result data. In the Vue project, we can use the Axios library for request interface operations.
In the root directory of the Vue project, use the command line to execute:
npm install axios
In the root directory of the Vue project, create a new service.js file, encapsulating it based on Axios API request process and provide it as a separate Vue plug-in for the entire application.
//Introduce axios library
import axios from "axios";
//Create axios instance
const service = axios.create({
baseURL: "http://192.168.0.102", timeout: 10000
});
export default ({ Vue }) => {
Vue.prototype.$axios = service;
}
In the index.js file in the src directory, we need to use the service.js file we just encapsulated as Vue plugin to register.
import Vue from 'vue'
import service from './service' //Introduce service
//Register service plug-in
Vue.use(service, { Vue })
When we click the button and execute the login logic, we need to call the background to verify and obtain the login result data. The login result data is stored in Vuex and registered through the Vue plug-in Vuex.
In the src directory, create a new store directory to store Vuex-related operations. First, create a new types.js file in the store directory to define the operation type of Vuex:
// types.js
export const LOGIN_SUCCESS = "LOGIN_SUCCESS"; // Login successful
export const LOGIN_FAIL = "LOGIN_FAIL"; // Login failed
Create a new actions.js file in the store directory, and encapsulate Vuex actions to trigger these actions in the Vue component and update the state.
import * as types from './types.js'
export default {
login: ({ commit }, param) => new Promise((resolve, reject) => { const { username, password } = param; this.$axios.post('/login', { username, password }).then(res => { const data = res.data; if (data.code === 200) { commit(types.LOGIN_SUCCESS, { data }); resolve(); } else { commit(types.LOGIN_FAIL, { message: data.message }); reject(data.message); } }) })
}
In the src/store directory, create a new store.js file , create related content such as stata, mutations and actions.
// store.js
import Vue from 'vue'
import Vuex from 'vuex'
import actions from './actions.js'
import * as types from './types.js'
Vue.use(Vuex)
const store = new Vuex.Store({
state: { info: { isLogged: false, // 是否登录 name: "", // 登录用户名 } }, mutations: { [types.LOGIN_SUCCESS](state, { data }) { state.info.isLogged = true; state.info.name = data.name; }, [types.LOGIN_FAIL](state, { message }) { state.info.isLogged = false; state.info.name = ""; console.log(message) } }, actions
})
export default store
In the main.js file in the src directory, we need to register store.js into Vue, as follows:
import Vue from 'vue'
import App from '. /App.vue'
import router from './router'
import store from './store/store.js'
Vue.config.productionTip = false
new Vue( {
router, store, // 注册Vuex render: h => h(App)
}).$mount('#app')
3) Jump to the page and output the parameter results
Introduce the state parameters isLogged and name into Vuex, When the login is successful, the status is updated in Vuex. After successful login, when jumping to a new page through routing, we need to pass the login information and output result parameters to the new page.
In the Vue project, we can choose the router programming method to jump to the new page through code.
In the router.js file, define two key fields to describe routing and login verification status, as well as output result parameters.
import Vue from 'vue'
import Router from 'vue-router'
import Home from './views/Home.vue'
import Login from './views/Login .vue'
Vue.use(Router)
const router = new Router({
mode: 'history', routes: [ { path: '/', name: 'home', component: Home, meta: { requiresAuth: true } // requireAuth用于表示页面是否需要登录才能访问,本例中仅仅对home页面设置了登录验证 }, { path: '/login', name: 'login', component: Login } ]
})
router.beforeEach((to, from, next) => { / / Perform page login verification when routing is switched
if (to.matched.some(record => record.meta.requiresAuth)) { const isLogged = store.state.info.isLogged; if (!isLogged) next({ name: 'login' }) else next() } else { next() }
})
When the login is successful, we need to use the router to jump and pass the output result parameters to the required components , this component needs to introduce the queryString library:
在登录成功的方法中:
import router from '../router'
import { stringify } from 'querystring'
commit(types.LOGIN_SUCCESS, { data })
const queryString = stringify({ name: data.name }) // 使用 queryString 库,将参数转化为字符串
router.push({ name: "home", query: { authInfo: window.btoa(queryString) }}) // 使用 router 进行跳转,这里使用了 Base64 编码策略,有兴趣的可以了解一下
在Home.vue组件中,我们通过created钩子,获取路由中的输入参数并将其输出。
export default {
name: 'home', created() { if (this.$route.query.authInfo !== undefined) { // 如果存在 authInfo const res = atob(this.$route.query.authInfo) // Base64解码操作 this.formData = qs.parse(res) // 请求参数解码操作 console.log(this.formData) // 输出参数信息 } }, data() { return { formData: {} } }
}
至此,我们已经成功使用Vue实现登录页跳转输出参数的功能。
结语
本文介绍了如何利用 Vue 实现跳转输出参数,通过 Vue-CLI、Axios、Vuex 和 Vue-Router 等技术栈,实现了基于Vue的网站登录验证功能。当然了,本文的实现是源于作者的参考和思考,如果您也有更好的实现方式,请跟我们一起分享并探讨。
The above is the detailed content of How to use Vue to implement login page jump output parameters. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
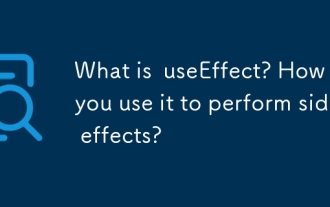
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.

Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.

Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
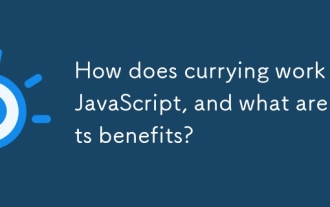
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
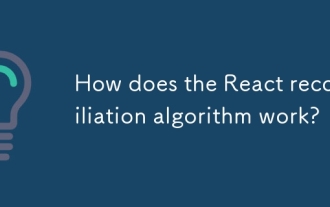
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
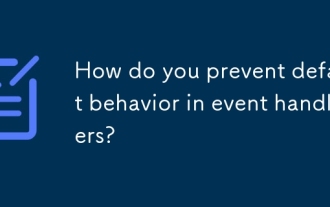
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
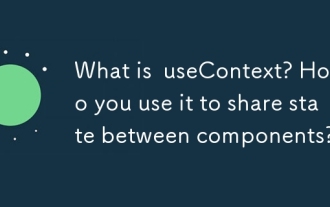
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
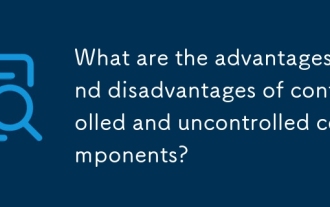
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.
