


How does Vue automatically request background data and render the page?
With the continuous updating of front-end technology, Vue.js, as an MVVM framework, is widely used in modern web application development. Vue.js frees developers from tedious DOM operations through data binding and componentization, making the development process more efficient and enjoyable. However, as applications become more complex, Vue.js requires developers to manually call APIs to request data from the backend to update content on the front-end page, which makes application development cumbersome and time-consuming. In this article, we will learn how to use Vue to automatically request background data and render the page to make development more efficient and simpler.
1. Overview of Vue components
In Vue.js, components are the basic unit for building applications, which allow developers to split applications into reusable, independent modules. Each Vue component contains HTML templates, Vue instance objects and attributes such as data and events. In Vue, components can be nested into each other based on the relationship between parent and child components to form a component tree to implement complex application functions.
2. Data transfer between Vue components
In Vue, in order to share data between different components, we need to use Vue's data binding mechanism for data transfer. There are mainly the following data binding methods in Vue:
1. Props
Props is a way to pass data from parent components to child components, similar to properties in React ( props). In the parent component, you can set the Props attribute in the child component just like setting attributes in HTML tags. The child component can directly access the data passed by the parent component through this.props.
2. Events
Events are a way to pass data from child components to parent components, similar to callbacks in React. In the child component, a custom event can be triggered through the $emit method, and data can be passed to the parent component. In the parent component, you can use v-on to bind custom events triggered by the child component just like binding native events, and you can receive data passed by the child component.
3. Vuex
Vuex is a state management method of Vue. It provides a globally unique state repository that can be accessed and modified by any component. By storing the data that needs to be shared in the Vuex state library, we can easily share and transfer data between different components.
3. Vue automatically requests background data
The life cycle hook function in Vue is an important feature of the Vue component. It provides different hook functions to facilitate developers in the Vue component life cycle. Different stages perform different operations. In Vue 2.x version, commonly used life cycle hook functions include created, mounted, updated, destroyed, etc. Among them, created and mounted are two commonly used life cycle hook functions, which are executed after the component is created and rendered to the page respectively.
In the Vue component, we can use the created and mounted life cycle hook functions to automatically request background data and update the content on the front-end page after the component is rendered to the page. The specific implementation steps are as follows:
1. Create a Vue component
<template> <div> <h1>Users List</h1> <ul> <li v-for="user in users" :key="user.id">{{ user.name }}</li> </ul> </div> </template> <script> export default { data() { return { users: [] } }, created() { this.fetchUsers() }, methods: { async fetchUsers() { const response = await fetch('/api/users') const data = await response.json() this.users = data } } } </script>
In the above code, we created a Vue component named UsersList, which contains a ul list to display the data from the background Requested user list data. In the data attribute of the component, we define an array named users to store user data requested from the background. In the component's create lifecycle hook function, we called the fetchUsers method to request background data. In the fetchUsers method, we use async/await syntax sugar to request background data asynchronously and store the results in the data attribute of the component.
2. Start the Vue application
import Vue from 'vue' import UsersList from './UsersList.vue' new Vue({ render: h => h(UsersList) }).$mount('#app')
In the above code, we introduced the Vue and UsersList components, and created a Vue instance object through the new Vue method. In the Vue instance object, we render the UsersList component to the page through the render function and mount it to the DOM node through the $mount method. In this way, after starting the Vue application, Vue will automatically call the created lifecycle hook function of the UsersList component to request data from the background and update the content on the front-end page.
4. Vue automatically refreshes the page
In the development of applications, we often need to implement the function of automatic page update, that is, when the background data changes, the front-end page can automatically update and display the latest data. In Vue, we can use Vue's responsive data mechanism and WebSocket protocol to implement the function of automatically updating the page.
1. Use Vue’s responsive data mechanism
In Vue, when the data attribute of the component changes, Vue will automatically re-render the content on the front-end page. Therefore, we can store the background data in the data attribute of the component, and regularly update the data attribute of the component through a timer or other methods to achieve the effect of automatic page updating.
2. Using the WebSocket protocol
The WebSocket protocol is a two-way communication protocol that can achieve full-duplex communication on the same persistent connection. In the application, we can use the WebSocket protocol to implement the function of the background server pushing data to the front end. When the background data changes, the background server can actively send data to the front-end client and update the content on the front-end page in real time.
5. Summary
As an important technology for modern Web application development, Vue.js has the characteristics of two-way data binding, componentization, data drive, etc., making the development of front-end applications more efficient and Pleasure. In this article, we learned how to use Vue components and life cycle hook functions to automatically request background data and render front-end pages. At the same time, we also introduced how Vue automatically refreshes the page, providing developers with more technical choices. I hope this article can be helpful to everyone and improve work efficiency.
The above is the detailed content of How does Vue automatically request background data and render the page?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
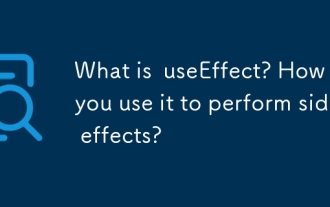
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.

Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.

Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
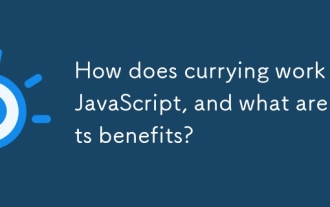
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
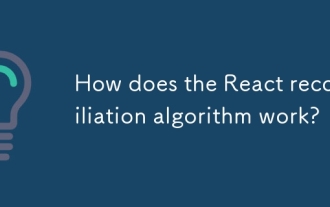
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
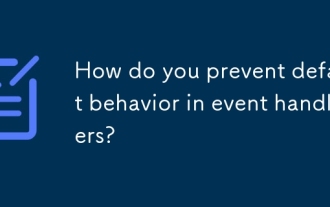
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
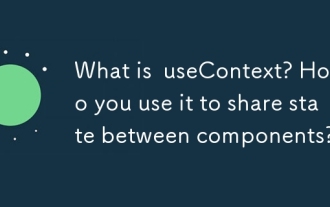
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
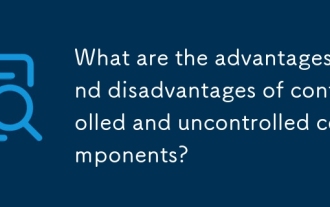
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.
