How to pass an array to the front end in php
In PHP, there are many ways to pass an array to the front end. This article will introduce several simple and easy-to-use methods so that beginners can easily understand them.
- Use the json_encode() function to convert the array to JSON format
JSON (JavaScript Object Notation) is a lightweight data exchange format. In PHP, we can use the json_encode() function to convert an array into JSON format, and then output the JSON string to the front-end page through the echo statement.
The following is a simple example:
<?php $array = array('name' => '张三', 'age' => 20, 'sex' => '男'); $json = json_encode($array); echo $json; ?>
This example converts an associative array into a JSON string and outputs it to the front-end page. On the front-end page, we can use JavaScript's JSON.parse() method to convert the JSON string back to a JavaScript object and then operate on it.
- Use the foreach statement to traverse the array and use echo to output each element
If you do not need to pass the entire array to the front end, you can use the foreach statement to traverse the array and use echo The statement outputs each element one by one.
The following is a sample code:
<?php $array = array('苹果', '香蕉', '橘子', '桃子'); foreach ($array as $key => $value) { echo "第".$key."个元素是".$value."<br/>"; } ?>
This example iterates through an indexed array and outputs each element using an echo statement. The output is:
第0个元素是苹果 第1个元素是香蕉 第2个元素是橘子 第3个元素是桃子
- Store the array in a JavaScript variable and use it on the front-end page
On the front-end page, we can also store the array in JavaScript variables and then perform operations. In PHP, we can use the json_encode() function to convert the array into JSON format and then output the result into a JavaScript variable.
The following is a sample code:
<?php $array = array('name' => '张三', 'age' => 20, 'sex' => '男'); $json = json_encode($array); echo "<script>var data=".$json.";</script>"; ?>
This example converts an associative array into a JSON string and outputs it to the JavaScript variable data. On the front-end page, we can use this variable to operate.
- Use Ajax asynchronous request to obtain the array
If the array is large or needs to be dynamically updated, you can use Ajax asynchronous request to obtain the array content. In PHP, we can use the json_encode() function to convert the array into JSON format, and then output the result to the front-end page or interface.
The following is a sample code:
<?php $array = array('苹果', '香蕉', '橘子', '桃子'); $json = json_encode($array); echo $json; ?>
This example converts an index array into a JSON string and outputs it to the front-end page. On the front-end page, we can use jQuery's get() method or native JavaScript's XMLHttpRequest object to send an asynchronous request to obtain the array content.
Summary:
In PHP, there are many ways to pass an array to the front end, including converting the array to JSON format, using the foreach statement to traverse the array, and storing the array in a JavaScript variable and use Ajax asynchronous requests to obtain arrays, etc. Different methods are suitable for different scenarios, and it is necessary to choose the appropriate method for the specific situation.
The above is the detailed content of How to pass an array to the front end in php. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
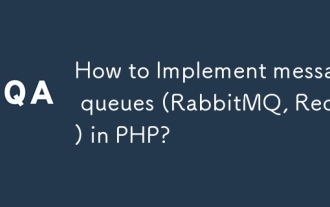
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
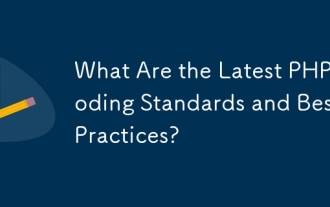
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
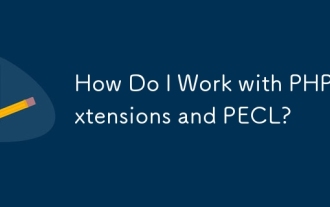
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
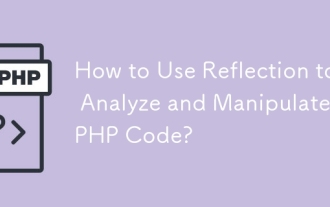
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
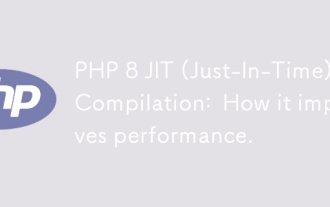
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
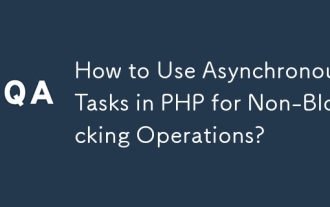
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
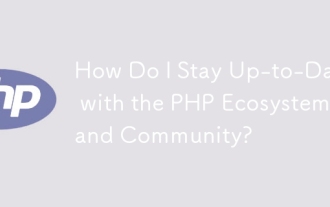
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
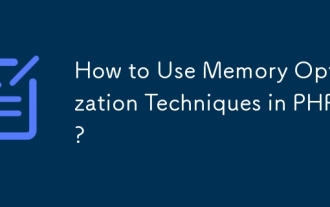
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v
