How to implement array deduplication in PHP
In PHP development, array deduplication is a frequently used operation. When processing array data, if duplicate elements appear, this will affect subsequent data processing and results, so the array needs to be deduplicated. This article will introduce how to implement array deduplication in PHP.
There are many ways to deduplicate arrays in PHP. You can use loop traversal, PHP built-in functions, etc. Next we will introduce them one by one.
- Use loop traversal
The most primitive method is of course to loop through the array and then remove duplicates based on element comparison. The code is as follows:
function unique($array) { $result = array(); foreach ($array as $value) { if (!in_array($value, $result)) { $result[] = $value; } } return $result; } $array = array(1, 2, 3, 2, 4, 3); print_r(unique($array)); // [1, 2, 3, 4]
In the above code, we use the foreach
statement and the in_array
function to loop through the array and compare whether the element already exists in the result array. If it does not exist, the element is added to the resulting array.
Using loop traversal can achieve array deduplication, but when there are a large number of elements in the array, this method will be very inefficient, perform poorly, and is not suitable for processing large-scale data.
- Use array_unique function
The built-in array_unique
function in PHP can directly perform deduplication operations on arrays. This function can receive an array as input and return a deduplicated array:
$array = array(1, 2, 3, 2, 4, 3); print_r(array_unique($array)); // [1, 2, 3, 4]
This function can easily deduplicate the array, which is very convenient. However, it should be noted that this function can only remove adjacent duplicate elements, so when there are non-adjacent duplicate elements in the array, this function is powerless.
- Use the array_flip and array_keys functions
The array_flip
function in PHP can swap the keys and values of the array to obtain a new array. If there are duplicate values in the array, the keys will be overwritten, and finally we can use the array_keys
function to get the deduplicated array. The code is as follows:
$array = array(1, 2, 3, 2, 4, 3); $array = array_flip($array); $array = array_keys($array); print_r($array); // [1, 2, 3, 4]
- Using the array_reduce function
The array_reduce
function in PHP can reduce an array to a single value while iterating it. We can use this function to deduplicate arrays. The code is as follows:
function unique($array) { return array_reduce($array, function($result, $value) { if (!in_array($value, $result)) { $result[] = $value; } return $result; }, array()); } $array = array(1, 2, 3, 2, 4, 3); print_r(unique($array)); // [1, 2, 3, 4]
In the above code, we use the array_reduce
function and anonymous function (closure) to deduplicate the array. At each iteration, the current element is compared with the elements that have been processed, duplicate elements are removed, and the result array is returned.
Summary
There are many ways to implement array deduplication in PHP. We can use loop traversal, built-in functions and other methods. In actual development, selection should be made based on actual conditions in order to achieve better performance and effects.
The above is the detailed content of How to implement array deduplication in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


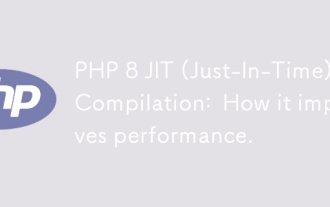
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
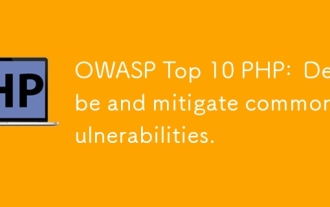
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
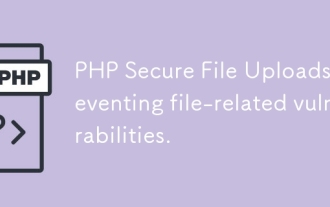
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
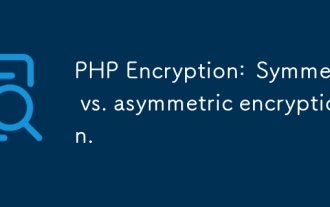
The article discusses symmetric and asymmetric encryption in PHP, comparing their suitability, performance, and security differences. Symmetric encryption is faster and suited for bulk data, while asymmetric is used for secure key exchange.

The article discusses implementing robust authentication and authorization in PHP to prevent unauthorized access, detailing best practices and recommending security-enhancing tools.
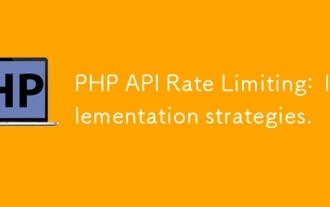
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
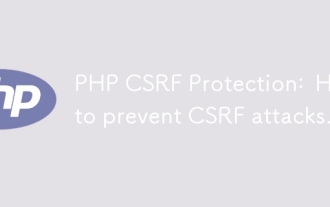
The article discusses strategies to prevent CSRF attacks in PHP, including using CSRF tokens, Same-Site cookies, and proper session management.

Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
