How to convert PHP collection to array
In the PHP development process, we often need to process collection type data. Collection type data in PHP are usually arrays composed of objects. When we need to process these collection data, we sometimes need to convert them into array format to facilitate subsequent processing operations. This article will introduce how to convert a PHP collection into an array.
1. Use foreach loop
The most common way to convert a PHP collection into an array is to use a foreach loop. For example, consider the following collection:
$col = new Collection();
$col->add(new Foo("Bill", "Denver"));
$col->add(new Foo("Jane", "Seattle"));
$col->add(new Foo("Ted", "San Francisco"));
It can be easily converted to an array using a foreach loop:
$arr = array();
foreach ($col as $item) {
$arr[] = $item->getName();
}
In this way, $arr stores the name attributes of all Foo objects.
2. Use array_map function
Another method is to use PHP’s built-in array_map() function. The array_map() function can accept an array and an anonymous function as parameters, and then pass each element in the array to the anonymous function for conversion processing. For example, suppose we have the following collection:
$col = new Collection();
$col->add(new Foo("Bill", "Denver"));
$col->add(new Foo("Jane", "Seattle"));
$col->add(new Foo("Ted", "San Francisco") );
To extract the name attributes of all Foo objects into an array, we can use the following code:
$arr = array_map(function($item) {
return $item->getName();
}, $col->toArray());
In this way, $arr stores the name attributes of all Foo objects.
3. Use the array_column function
PHP also provides a convenient built-in function array_column(), which can extract a column from a multi-dimensional array to form a new one-dimensional array. For example, suppose we have the following collection:
$data = array(
array('id' => 1, 'name' => 'Bill', 'city' = > 'Denver'),
array('id' => 2, 'name' => 'Jane', 'city' => 'Seattle'),
array('id' => 3, 'name' => 'Ted', 'city' => 'San Francisco')
);
To list all the cities To extract attributes as an array, you can use the following code:
$arr = array_column($data, 'city');
In this way, all city attribute values are stored in $arr.
In summary, when processing PHP collection data, converting it into array format can facilitate subsequent processing operations. This article introduces three commonly used methods: using foreach loop, using array_map function and using array_column function. Developers can choose the most suitable method according to the actual situation.
The above is the detailed content of How to convert PHP collection to array. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


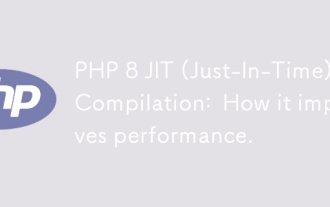
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
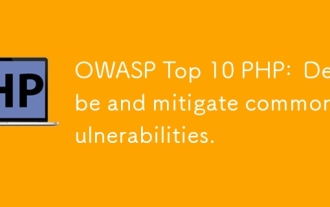
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
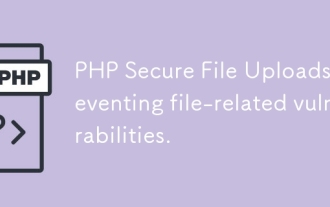
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
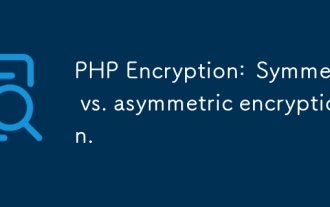
The article discusses symmetric and asymmetric encryption in PHP, comparing their suitability, performance, and security differences. Symmetric encryption is faster and suited for bulk data, while asymmetric is used for secure key exchange.

Prepared statements in PHP enhance database security and efficiency by preventing SQL injection and improving query performance through compilation and reuse.Character count: 159
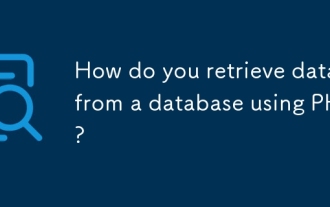
Article discusses retrieving data from databases using PHP, covering steps, security measures, optimization techniques, and common errors with solutions.Character count: 159

The article discusses implementing robust authentication and authorization in PHP to prevent unauthorized access, detailing best practices and recommending security-enhancing tools.
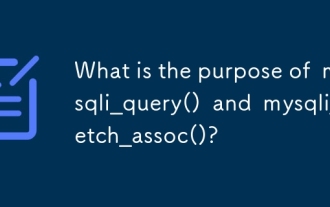
The article discusses the mysqli_query() and mysqli_fetch_assoc() functions in PHP for MySQL database interactions. It explains their roles, differences, and provides a practical example of their use. The main argument focuses on the benefits of usin
