How to determine whether an array or an object in php
In PHP, arrays and objects are two commonly used data types. Although they have some similar properties, they also need to be treated differently when dealing with them. Determining whether a variable is an array or an object can help us process data more accurately in programming.
Judge Array
To determine whether a variable is an array, you can use the is_array function in PHP. The return value of this function is of Boolean type. If the variable is an array, it returns true, otherwise it returns false.
The following is a sample code that uses the is_array function to determine an array:
$arr = array(1, 2, 3); if(is_array($arr)){ echo "This is an array."; }else{ echo "This is not an array."; }
Run the above code, the output result is "This is an array."
Judge object
To determine whether a variable is an object, you can use the is_object function in PHP. The return value of this function is of Boolean type. If the variable is an object, it returns true, otherwise it returns false.
The following is a sample code that uses the is_object function to determine the object:
class Person{ public $name; public $age; } $person = new Person(); if(is_object($person)){ echo "This is an object."; }else{ echo "This is not an object."; }
Run the above code, the output result is "This is an object."
Distinguish between arrays and objects
In PHP, the syntax of arrays and objects has some similarities. For example, they both use methods similar to $variable->key to access their elements. So sometimes there will be situations where the judgment variable can be either an array or an object.
In this case, you can first determine whether the variable is an object, and if it is an object, then determine whether it is an instance of the stdClass class. If it is an instance of the stdClass class, then you can conclude that the variable is an object, otherwise it is determined to be an array.
The following is a complete sample code for judging arrays and objects:
function getTypeofVar($var){ if(is_object($var)){ if(get_class($var) == "stdClass"){ return "object"; }else{ return "unknown"; } }elseif(is_array($var)){ return "array"; }else{ return "unknown"; } }
Run the above code, you can judge whether a variable is an array or an object through the getTypeofVar function.
Summary
To determine whether a variable is an array or an object, you can use PHP's built-in is_array and is_object functions. If you need to determine whether a variable is an array or an object, you can first determine whether the variable is an object, and then determine whether the object is an instance of the stdClass class. This can help us process data more accurately and improve programming efficiency.
The above is the detailed content of How to determine whether an array or an object in php. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
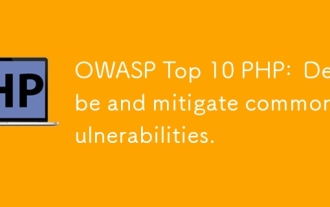
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
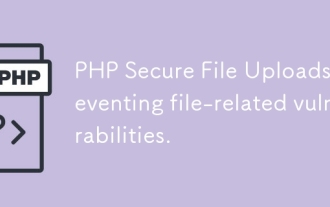
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.

Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
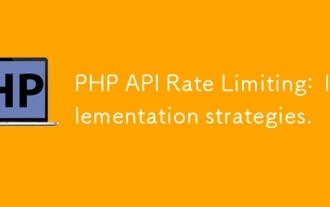
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
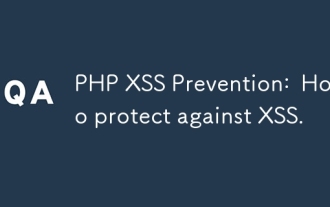
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.
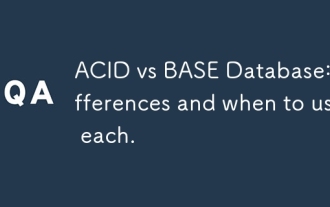
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
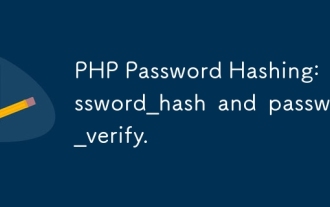
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
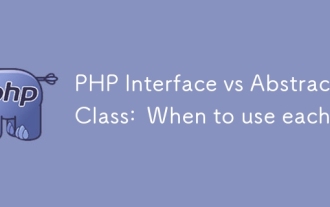
The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct
