How to implement the function of replacing fonts in PDF in Java
Introducing jar
Configure pom.xml in the Maven program:
<repositories> <repository> <id>com.e-iceblue</id> <url>https://repo.e-iceblue.cn/repository/maven-public/</url> </repository> </repositories> <dependencies> <dependency> <groupId>e-iceblue</groupId> <artifactId>spire.pdf.free</artifactId> <version>5.1.0</version> </dependency> </dependencies>
If you need to import manually, you can download the Jar package locally, then unzip it and find Spire in the lib folder .Pdf.jar file. Open "Project Structure" in IDEA and import the jar under the local path into the program, as shown in the figure:
Java code
1. Replace all fonts
Idea: After loading the PDF document, obtain the fonts in the source document, then define new fonts, replace the original fonts, and finally save the document.
Java
import com.spire.pdf.*; import com.spire.pdf.graphics.PdfFont; import com.spire.pdf.graphics.PdfFontFamily; import com.spire.pdf.graphics.PdfFontStyle; import com.spire.pdf.graphics.fonts.PdfUsedFont; public class ReplaceAllFonts { public static void main(String[] args) throws Exception{ //创建PdfDocument类的对象 PdfDocument pdf = new PdfDocument(); //加载PDF文档 pdf.loadFromFile("input.pdf"); //获取文档中的所有字体 PdfUsedFont[] fonts = pdf.getUsedFonts(); //遍历所有字体 for (PdfUsedFont font: fonts) { //获取字体大小 float fontSize = font.getSize(); //创建新字体 PdfFont newfont = new PdfFont(PdfFontFamily.Times_Roman, fontSize, PdfFontStyle.Italic); //替换原有字体 font.replace(newfont); } //保存文档 pdf.saveToFile("ReplaceAllFonts.pdf"); pdf.dispose(); } }
2. Replace the specified font
Idea: Load PDF After searching the document, search and obtain the specified font in the document, then define a new font, replace the found original font, and finally save the document.
Java
import com.spire.pdf.PdfDocument; import com.spire.pdf.graphics.*; import com.spire.pdf.graphics.fonts.PdfUsedFont; public class ReplaceSpecificFont { public static void main(String[] args) throws Exception{ //创建PdfDocument类的对象 PdfDocument pdf = new PdfDocument(); //加载PDF文档 pdf.loadFromFile("sample.pdf"); //获取文档中的所有字体 PdfUsedFont[] fonts = pdf.getUsedFonts(); //遍历所有字体 for (PdfUsedFont font: fonts) { //判断符合条件的字体 if(font.getName().equals("Calibri")) { //获取字体大小 float fontSize = font.getSize(); //创建新字体 PdfFont newfont = new PdfFont(PdfFontFamily.Times_Roman, fontSize, PdfFontStyle.Italic); //替换Calibri字体 font.replace(newfont); } } //保存文档 pdf.saveToFile("ReplaceSpecificFont.pdf"); pdf.dispose(); } }
The above is the detailed content of How to implement the function of replacing fonts in PDF in Java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
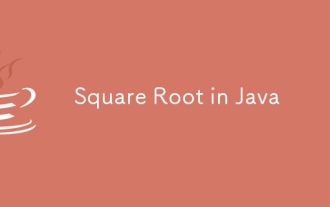
Guide to Square Root in Java. Here we discuss how Square Root works in Java with example and its code implementation respectively.
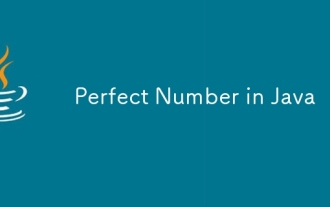
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
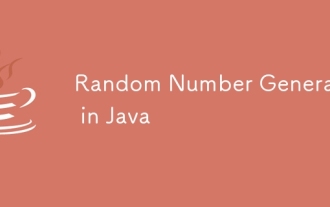
Guide to Random Number Generator in Java. Here we discuss Functions in Java with examples and two different Generators with ther examples.
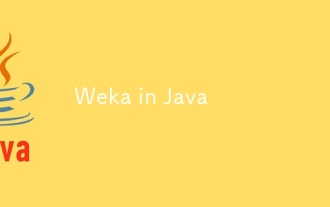
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
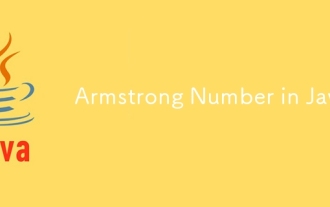
Guide to the Armstrong Number in Java. Here we discuss an introduction to Armstrong's number in java along with some of the code.
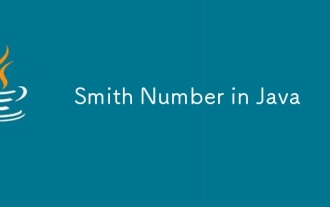
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
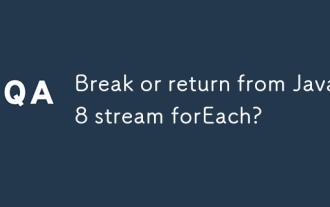
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
