


How to use python artificial intelligence algorithm artificial neural network
Artificial Neural Network
(Artificial Neural Network, ANN) is a mathematical model that imitates the structure and function of biological neural networks. Its purpose is to be able to process unknown input data through learning and training. Carry out complex non-linear mapping relationships to achieve adaptive intelligent decision-making. It can be said that ANN is the most basic and core algorithm among artificial intelligence algorithms.
The basic structure of the ANN model includes input layer, hidden layer and output layer. The input layer receives input data, the hidden layer is responsible for multi-level, high-dimensional transformation and processing of data, and the output layer outputs the processed data. The training process of ANN is to continuously adjust the weights of each layer in the neural network through multiple iterations, so that the neural network can correctly predict and classify the input data.
Artificial Neural Network Algorithm Example
Next look at a simple artificial neural network algorithm example:
import numpy as np class NeuralNetwork(): def __init__(self, layers): """ layers: 数组,包含每个层的神经元数量,例如 [2, 3, 1] 表示 3 层神经网络,第一层 2 个神经元,第二层 3 个神经元,第三层 1 个神经元。 weights: 数组,包含每个连接的权重矩阵,默认值随机生成。 biases: 数组,包含每个层的偏差值,默认值为 0。 """ self.layers = layers self.weights = [np.random.randn(a, b) for a, b in zip(layers[1:], layers[:-1])] self.biases = [np.zeros((a, 1)) for a in layers[1:]] def sigmoid(self, z): """Sigmoid 激活函数.""" return 1 / (1 + np.exp(-z)) def forward_propagation(self, a): """前向传播.""" for w, b in zip(self.weights, self.biases): z = np.dot(w, a) + b a = self.sigmoid(z) return a def backward_propagation(self, x, y): """反向传播.""" nabla_w = [np.zeros(w.shape) for w in self.weights] nabla_b = [np.zeros(b.shape) for b in self.biases] a = x activations = [x] zs = [] for w, b in zip(self.weights, self.biases): z = np.dot(w, a) + b zs.append(z) a = self.sigmoid(z) activations.append(a) delta = self.cost_derivative(activations[-1], y) * self.sigmoid_prime(zs[-1]) nabla_b[-1] = delta nabla_w[-1] = np.dot(delta, activations[-2].transpose()) for l in range(2, len(self.layers)): z = zs[-l] sp = self.sigmoid_prime(z) delta = np.dot(self.weights[-l+1].transpose(), delta) * sp nabla_b[-l] = delta nabla_w[-l] = np.dot(delta, activations[-l-1].transpose()) return (nabla_w, nabla_b) def train(self, x_train, y_train, epochs, learning_rate): """训练网络.""" for epoch in range(epochs): nabla_w = [np.zeros(w.shape) for w in self.weights] nabla_b = [np.zeros(b.shape) for b in self.biases] for x, y in zip(x_train, y_train): delta_nabla_w, delta_nabla_b = self.backward_propagation(np.array([x]).transpose(), np.array([y]).transpose()) nabla_w = [nw+dnw for nw, dnw in zip(nabla_w, delta_nabla_w)] nabla_b = [nb+dnb for nb, dnb in zip(nabla_b, delta_nabla_b)] self.weights = [w-(learning_rate/len(x_train))*nw for w, nw in zip(self.weights, nabla_w)] self.biases = [b-(learning_rate/len(x_train))*nb for b, nb in zip(self.biases, nabla_b)] def predict(self, x_test): """预测.""" y_predictions = [] for x in x_test: y_predictions.append(self.forward_propagation(np.array([x]).transpose())[0][0]) return y_predictions def cost_derivative(self, output_activations, y): """损失函数的导数.""" return output_activations - y def sigmoid_prime(self, z): """Sigmoid 函数的导数.""" return self.sigmoid(z) * (1 - self.sigmoid(z))
Use the following code example to instantiate and use this simple neural network algorithm Network class:
x_train = [[0, 0], [1, 0], [0, 1], [1, 1]] y_train = [0, 1, 1, 0] # 创建神经网络 nn = NeuralNetwork([2, 3, 1]) # 训练神经网络 nn.train(x_train, y_train, 10000, 0.1) # 测试神经网络 x_test = [[0, 0], [1, 0], [0, 1], [1, 1]] y_test = [0, 1, 1, 0] y_predictions = nn.predict(x_test) print("Predictions:", y_predictions) print("Actual:", y_test)
Output results:
Predictions: [0.011602156431658403, 0.9852717774725432, 0.9839448924887225, 0.020026540429992387]
Actual: [0, 1, 1, 0]
The above is the detailed content of How to use python artificial intelligence algorithm artificial neural network. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


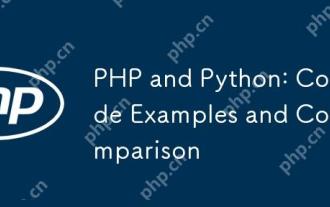
PHP and Python have their own advantages and disadvantages, and the choice depends on project needs and personal preferences. 1.PHP is suitable for rapid development and maintenance of large-scale web applications. 2. Python dominates the field of data science and machine learning.
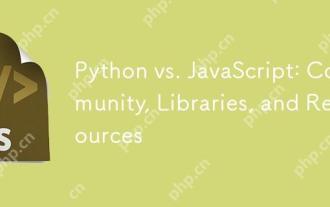
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
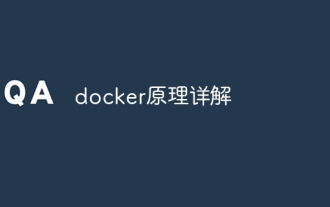
Docker uses Linux kernel features to provide an efficient and isolated application running environment. Its working principle is as follows: 1. The mirror is used as a read-only template, which contains everything you need to run the application; 2. The Union File System (UnionFS) stacks multiple file systems, only storing the differences, saving space and speeding up; 3. The daemon manages the mirrors and containers, and the client uses them for interaction; 4. Namespaces and cgroups implement container isolation and resource limitations; 5. Multiple network modes support container interconnection. Only by understanding these core concepts can you better utilize Docker.
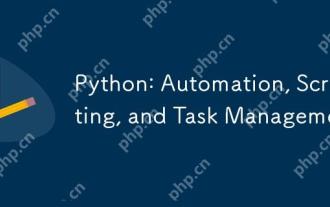
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
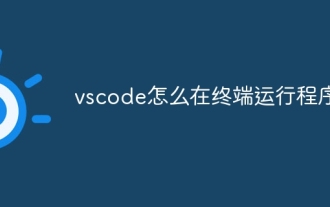
In VS Code, you can run the program in the terminal through the following steps: Prepare the code and open the integrated terminal to ensure that the code directory is consistent with the terminal working directory. Select the run command according to the programming language (such as Python's python your_file_name.py) to check whether it runs successfully and resolve errors. Use the debugger to improve debugging efficiency.
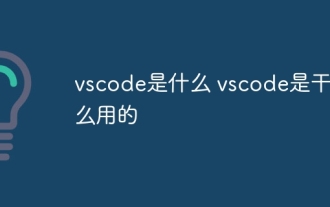
VS Code is the full name Visual Studio Code, which is a free and open source cross-platform code editor and development environment developed by Microsoft. It supports a wide range of programming languages and provides syntax highlighting, code automatic completion, code snippets and smart prompts to improve development efficiency. Through a rich extension ecosystem, users can add extensions to specific needs and languages, such as debuggers, code formatting tools, and Git integrations. VS Code also includes an intuitive debugger that helps quickly find and resolve bugs in your code.
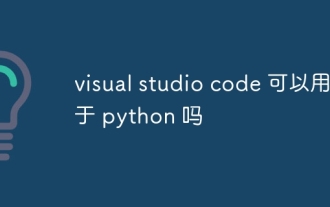
VS Code can be used to write Python and provides many features that make it an ideal tool for developing Python applications. It allows users to: install Python extensions to get functions such as code completion, syntax highlighting, and debugging. Use the debugger to track code step by step, find and fix errors. Integrate Git for version control. Use code formatting tools to maintain code consistency. Use the Linting tool to spot potential problems ahead of time.
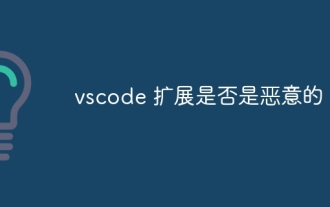
VS Code extensions pose malicious risks, such as hiding malicious code, exploiting vulnerabilities, and masturbating as legitimate extensions. Methods to identify malicious extensions include: checking publishers, reading comments, checking code, and installing with caution. Security measures also include: security awareness, good habits, regular updates and antivirus software.
