How to implement binary tree in Python
Python implements a binary tree
Python can implement a binary tree using object-oriented programming by defining a binary tree node class. Each node contains a data element, left and right child node pointers and some operation methods, such as inserting nodes, finding nodes, deleting nodes, etc.
The following is a simple binary tree implementation example:
class Node: def __init__(self, data): self.data = data self.left = None self.right = None def insert(self, data): if self.data: if data < self.data: if self.left is None: self.left = Node(data) else: self.left.insert(data) elif data > self.data: if self.right is None: self.right = Node(data) else: self.right.insert(data) else: self.data = data def find(self, data): if data < self.data: if self.left is None: return str(data) + " Not Found" return self.left.find(data) elif data > self.data: if self.right is None: return str(data) + " Not Found" return self.right.find(data) else: return str(self.data) + " is found" def inorder_traversal(self, root): res = [] if root: res = self.inorder_traversal(root.left) res.append(root.data) res = res + self.inorder_traversal(root.right) return res
In the above code, the Node class defines a node, including the data element data, and the left and right child node pointers left and right. The insert method is used to insert nodes into a binary tree, the find method is used to find whether a specific node exists in the binary tree, and the inorder_traversal method is used to perform in-order traversal of the binary tree.
The following is how to use this Node class to create a binary tree:
root = Node(50) root.insert(30) root.insert(20) root.insert(40) root.insert(70) root.insert(60) root.insert(80) # 查找节点 print(root.find(70)) # Output: 70 is found print(root.find(90)) # Output: 90 Not Found # 中序遍历 print(root.inorder_traversal(root)) # Output: [20, 30, 40, 50, 60, 70, 80]
In the above code, a root node root is first created, then the insert method is used to insert a node into the tree, and finally using The find method finds nodes and uses the inorder_traversal method to perform in-order traversal of the binary tree.
In addition to insertion, search and traversal methods, binary trees also have other operation methods, such as deleting nodes, determining whether it is a binary search tree, calculating the depth of the tree, etc. The following is a slightly more complete binary tree sample code:
class Node: def __init__(self, data): self.data = data self.left = None self.right = None def insert(self, data): if self.data: if data < self.data: if self.left is None: self.left = Node(data) else: self.left.insert(data) elif data > self.data: if self.right is None: self.right = Node(data) else: self.right.insert(data) else: self.data = data def find(self, data): if data < self.data: if self.left is None: return None return self.left.find(data) elif data > self.data: if self.right is None: return None return self.right.find(data) else: return self def delete(self, data): if self is None: return self if data < self.data: self.left = self.left.delete(data) elif data > self.data: self.right = self.right.delete(data) else: if self.left is None: temp = self.right self = None return temp elif self.right is None: temp = self.left self = None return temp temp = self.right.minimum() self.data = temp.data self.right = self.right.delete(temp.data) return self def minimum(self): if self.left is None: return self return self.left.minimum() def is_bst(self): if self.left: if self.left.data > self.data or not self.left.is_bst(): return False if self.right: if self.right.data < self.data or not self.right.is_bst(): return False return True def height(self, node): if node is None: return 0 left_height = self.height(node.left) right_height = self.height(node.right) return max(left_height, right_height) + 1 def inorder_traversal(self, root): res = [] if root: res = self.inorder_traversal(root.left) res.append(root.data) res = res + self.inorder_traversal(root.right) return res
In this example, we have added the delete method to delete the specified node; the minimum method to find the smallest node in the tree; the is_bst method to determine the current Whether the tree is a binary search tree; the height method is used to calculate the depth of the tree.
We can use the following code to test the new method:
# 创建二叉树 root = Node(50) root.insert(30) root.insert(20) root.insert(40) root.insert(70) root.insert(60) root.insert(80) # 删除节点 print("Deleting node 20:") root.delete(20) print(root.inorder_traversal(root)) # 判断是否为二叉搜索树 print("Is it a BST?:", root.is_bst()) # 计算树的深度 print("Tree height:", root.height(root))
In this way we have completed a relatively complete binary tree implementation, and also demonstrated how to use object-oriented programming in Python ideas to implement a data structure.
Finally, the complete binary tree class implementation code is attached:
class Node: def __init__(self, data): self.data = data self.left = None self.right = None def insert(self, data): if self.data: if data < self.data: if self.left is None: self.left = Node(data) else: self.left.insert(data) elif data > self.data: if self.right is None: self.right = Node(data) else: self.right.insert(data) else: self.data = data def find(self, data): if data < self.data: if self.left is None: return None return self.left.find(data) elif data > self.data: if self.right is None: return None return self.right.find(data) else: return self def delete(self, data): if self is None: return self if data < self.data: self.left = self.left.delete(data) elif data > self.data: self.right = self.right.delete(data) else: if self.left is None: temp = self.right self = None return temp elif self.right is None: temp = self.left self = None return temp temp = self.right.minimum() self.data = temp.data self.right = self.right.delete(temp.data) return self def minimum(self): if self.left is None: return self return self.left.minimum() def is_bst(self): if self.left: if self.left.data > self.data or not self.left.is_bst(): return False if self.right: if self.right.data < self.data or not self.right.is_bst(): return False return True def height(self, node): if node is None: return 0 left_height = self.height(node.left) right_height = self.height(node.right) return max(left_height, right_height) + 1 def inorder_traversal(self, root): res = [] if root: res = self.inorder_traversal(root.left) res.append(root.data) res = res + self.inorder_traversal(root.right) return res if __name__ == '__main__': # 创建二叉树 root = Node(50) root.insert(30) root.insert(20) root.insert(40) root.insert(70) root.insert(60) root.insert(80) # 删除节点 print("Deleting node 20:") root.delete(20) print(root.inorder_traversal(root)) # 判断是否为二叉搜索树 print("Is it a BST?:", root.is_bst()) # 计算树的深度 print("Tree height:", root.height(root))
After running the code, you can get the following output:
Deleting node 20 :
[30, 40, 50, 60, 70, 80]
Is it a BST?: True
Tree height: 3
This example includes insertion and search , delete, traverse, determine whether it is a binary search tree and calculate the depth of the tree, etc.
The above is the detailed content of How to implement binary tree in Python. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


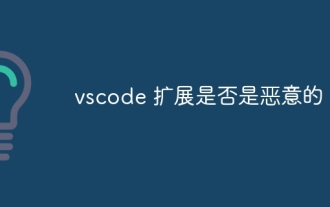
VS Code extensions pose malicious risks, such as hiding malicious code, exploiting vulnerabilities, and masturbating as legitimate extensions. Methods to identify malicious extensions include: checking publishers, reading comments, checking code, and installing with caution. Security measures also include: security awareness, good habits, regular updates and antivirus software.
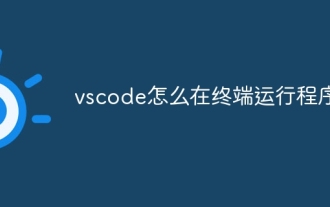
In VS Code, you can run the program in the terminal through the following steps: Prepare the code and open the integrated terminal to ensure that the code directory is consistent with the terminal working directory. Select the run command according to the programming language (such as Python's python your_file_name.py) to check whether it runs successfully and resolve errors. Use the debugger to improve debugging efficiency.
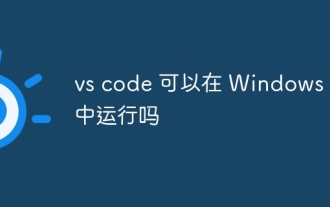
VS Code can run on Windows 8, but the experience may not be great. First make sure the system has been updated to the latest patch, then download the VS Code installation package that matches the system architecture and install it as prompted. After installation, be aware that some extensions may be incompatible with Windows 8 and need to look for alternative extensions or use newer Windows systems in a virtual machine. Install the necessary extensions to check whether they work properly. Although VS Code is feasible on Windows 8, it is recommended to upgrade to a newer Windows system for a better development experience and security.
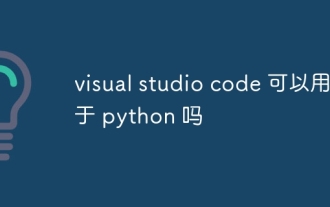
VS Code can be used to write Python and provides many features that make it an ideal tool for developing Python applications. It allows users to: install Python extensions to get functions such as code completion, syntax highlighting, and debugging. Use the debugger to track code step by step, find and fix errors. Integrate Git for version control. Use code formatting tools to maintain code consistency. Use the Linting tool to spot potential problems ahead of time.
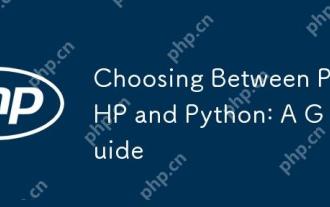
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
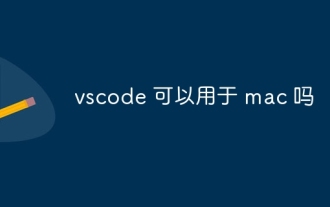
VS Code is available on Mac. It has powerful extensions, Git integration, terminal and debugger, and also offers a wealth of setup options. However, for particularly large projects or highly professional development, VS Code may have performance or functional limitations.
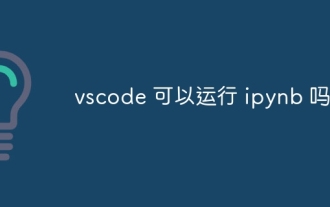
The key to running Jupyter Notebook in VS Code is to ensure that the Python environment is properly configured, understand that the code execution order is consistent with the cell order, and be aware of large files or external libraries that may affect performance. The code completion and debugging functions provided by VS Code can greatly improve coding efficiency and reduce errors.
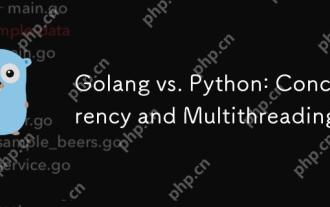
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
