


One line of code converts a Python program into a graphical interface application
Project Gooey supports converting (almost) any Python 2 or 3 console program into a GUI application with one line of code.
1. Quick start
Before you start, you must ensure that Python and pip have been successfully installed in On your computer, if not, you can visit this article: Super Detailed Python Installation Guide to install it.
If you use Python for data analysis, you can install Anaconda directly: Anaconda, a good helper for Python data analysis and mining, has built-in Python and pip.
In addition, it is recommended that you use VSCode Editor, it has many advantages: the best partner for Python programming—VSCode detailed guide.
Please choose any of the following methods to enter the command to install dependencies:
- Windows environment Open Cmd (Start-Run-CMD).
- MacOS environment Open Terminal (command space and enter Terminal).
- If you are using VSCode editor or Pycharm, you can directly use the Terminal at the bottom of the interface.
(Method 1) Install Gooey The simplest way is to install Gooey through PIP:
pipGooey
(Method 2). Alternatively, you can install Gooey
git clone https://github.com/chriskiehl/Gooey.git
by cloning the project to a local directory. After unzipping, enter the folder and run setup.py:
python setup.py install
2. How to use
Gooey By attaching a simple decorator to the main function, and then using GooeyParser you can The parameters that need to be used are visualized as text boxes, selection boxes or even file selection boxes.
For example, when downloading articles from scihub literature, we need to enter two parameters: 1. Keywords, 2. Number of downloaded articles, which can be changed using Gooey:
gooeyGooey, GooeyParser : parser = GooeyParser(description=) parser.add_argument(, help=, widget=) parser.add_argument(, help=) parser.add_argument(, help=) args = parser.parse_args() search(args.keywords, int(args.limit), args.path)
GooeyParser is the same as ArgumentParser. You can add input parameters using add_argument. The difference is that GooeyParser provides visual options:
parser.add_argument(, help=, widget=)
In this line of code, the widget parameter is provided to the args.path variable. A directory selector (widget="DirChooser") is provided. The help parameter is used to remind the user of the role of the selector. The effect is as follows:
When you do not provide the widget parameter , the program uses the text input box by default.
parser.add_argument(, help=) parser.add_argument(, help=)
Gooey will automatically arrange your parameters, so you don't need to worry about the display of individual text boxes or selection boxes. in the code:
args = parser.parse_args() search(args.keywords, int(args.limit), args.path)
args = parser.parse_args() can convert all the text input by the user into the variable value of the corresponding object, through args.var Extract the corresponding variable value directly.
The complete code and effect of this simple visualization program are as follows:
import asyncio from scihub import SciHub from gooey import Gooey, GooeyParser : """ 搜索相关论文并下载 Args: keywords (str): 关键词 limit (int): 篇数 path (str): 下载路径 """ sh = SciHub() result = sh.search(keywords, limit=limit) print(result) loop = asyncio.get_event_loop() # 获取所有需要下载的scihub直链 tasks = [sh.async_get_direct_url(paper["url"]) for paper in result.get("papers", [])] all_direct_urls = loop.run_until_complete(asyncio.gather(*tasks)) print(all_direct_urls) # 下载所有论文 loop.run_until_complete(sh.async_download(loop, all_direct_urls, path=path)) loop.close() @Gooey : parser = GooeyParser(description="中文环境可用的scihub下载器 - @Python实用宝典") parser.add_argument('path', help="下载路径", widget="DirChooser") parser.add_argument('keywords', help="关键词") parser.add_argument('limit', help="下载篇数") args = parser.parse_args() search(args.keywords, int(args.limit), args.path) main()
If you want this code to run perfectly, please combine it with the scihub of the python super literature batch search and download tool that you have to know. py.
You can also use your own program for graphical interface, it doesn't matter.
The effect is as follows:
##3. Supported widget components
All supported widget components are as follows: 1. Check box widget="CheckBox"##2. Drop-down box
##3. Mutually exclusive selection box widget="RadioGroup"
4. Selection boxes for various target types
5.日期/时间选择器 widget="DateChooser/TimeChooser"
6.密码输入框 wiget="PasswordField"
7.多选列表框 widget="Listbox"
8.颜色选择器 widget="ColourChooser"
9.可过滤的下拉框 widget="FilterableDropdown"
10.滑片 widget="Slider"
四、打包
在一切都测试完毕后使用正常后,你可以通过 pyinstaller 将这个可视化程序打包成exe可执行文件。
1.编写 PyInstaller buildspec
PyInstaller使用 buildspec 来确定如何捆绑项目。你可以在Python实用宝典后台回复 buildspec下载 build.spec.txt.
下载后你只需要改两行代码:
如下所示:
在路径前面带r,可以不用输入两个斜杆 '' 哦。
2.执行打包命令
为了能够使用 PyInstaller, 我们需要使用pip安装这个模块:
pip install pyinstaller
然后进入 build.spec.text 所在文件夹,执行以下命令打包程序:
pyinstaller build.spec.txt
打包完成后会在当前文件夹下生成一个dist文件夹,里面就包含了你打包生成的可执行文件,打包成功。
The above is the detailed content of One line of code converts a Python program into a graphical interface application. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


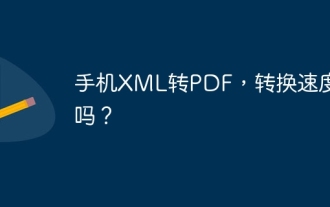
The speed of mobile XML to PDF depends on the following factors: the complexity of XML structure. Mobile hardware configuration conversion method (library, algorithm) code quality optimization methods (select efficient libraries, optimize algorithms, cache data, and utilize multi-threading). Overall, there is no absolute answer and it needs to be optimized according to the specific situation.
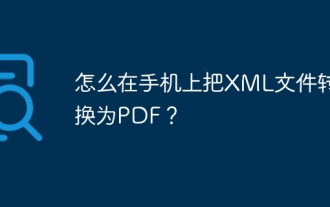
It is impossible to complete XML to PDF conversion directly on your phone with a single application. It is necessary to use cloud services, which can be achieved through two steps: 1. Convert XML to PDF in the cloud, 2. Access or download the converted PDF file on the mobile phone.
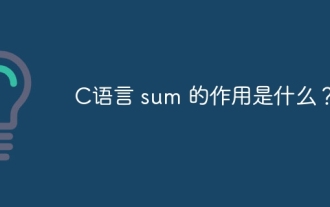
There is no built-in sum function in C language, so it needs to be written by yourself. Sum can be achieved by traversing the array and accumulating elements: Loop version: Sum is calculated using for loop and array length. Pointer version: Use pointers to point to array elements, and efficient summing is achieved through self-increment pointers. Dynamically allocate array version: Dynamically allocate arrays and manage memory yourself, ensuring that allocated memory is freed to prevent memory leaks.
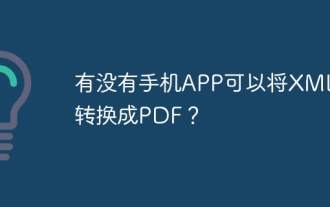
There is no APP that can convert all XML files into PDFs because the XML structure is flexible and diverse. The core of XML to PDF is to convert the data structure into a page layout, which requires parsing XML and generating PDF. Common methods include parsing XML using Python libraries such as ElementTree and generating PDFs using ReportLab library. For complex XML, it may be necessary to use XSLT transformation structures. When optimizing performance, consider using multithreaded or multiprocesses and select the appropriate library.
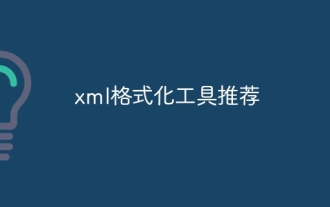
XML formatting tools can type code according to rules to improve readability and understanding. When selecting a tool, pay attention to customization capabilities, handling of special circumstances, performance and ease of use. Commonly used tool types include online tools, IDE plug-ins, and command-line tools.
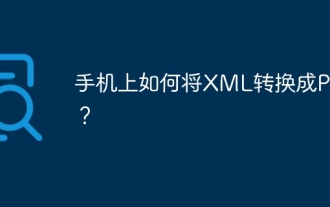
It is not easy to convert XML to PDF directly on your phone, but it can be achieved with the help of cloud services. It is recommended to use a lightweight mobile app to upload XML files and receive generated PDFs, and convert them with cloud APIs. Cloud APIs use serverless computing services, and choosing the right platform is crucial. Complexity, error handling, security, and optimization strategies need to be considered when handling XML parsing and PDF generation. The entire process requires the front-end app and the back-end API to work together, and it requires some understanding of a variety of technologies.
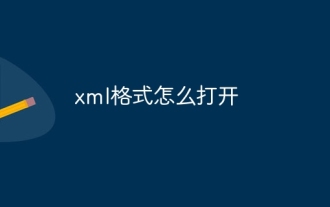
Use most text editors to open XML files; if you need a more intuitive tree display, you can use an XML editor, such as Oxygen XML Editor or XMLSpy; if you process XML data in a program, you need to use a programming language (such as Python) and XML libraries (such as xml.etree.ElementTree) to parse.
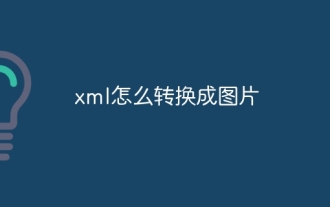
XML can be converted to images by using an XSLT converter or image library. XSLT Converter: Use an XSLT processor and stylesheet to convert XML to images. Image Library: Use libraries such as PIL or ImageMagick to create images from XML data, such as drawing shapes and text.
