golang receives request
With the rapid development of the Internet and the popularity of mobile devices, more and more applications require efficient operations and processing of massive data. As a new development language, Go language has received widespread attention and application. This article will introduce how to use Go language to receive and process HTTP requests.
1. HTTP request
HTTP (Hypertext Transfer Protocol) is a protocol used to transfer files (such as HTML, pictures, videos, etc.) and is the data in the Web. The basis for exchange. In the HTTP protocol, the client sends a request message to the server, and the server returns a response message after receiving the request.
HTTP request consists of three parts:
1. Request line: including request method, request address and HTTP protocol version. Commonly used request methods include GET, POST, PUT, DELETE, etc.
2. Request header: including client information, requested file type, cache control, etc.
3. Request body: The request body is optional and is usually used to submit form data.
2. HTTP processing in Go language
Go language has a built-in lightweight HTTP server that can easily handle HTTP requests. The Go language can implement HTTP servers and clients through the "net/http" package in the standard library.
- HTTP Server
HTTP server is usually a process or thread that communicates with clients and returns responses based on HTTP requests.
In the Go language, you can use the "ListenAndServe" function in the "net/http" package to implement the HTTP server.
The sample code is as follows:
package main import ( "fmt" "net/http" ) func main() { http.HandleFunc("/", indexHandler) http.ListenAndServe(":8080", nil) } func indexHandler(w http.ResponseWriter, r *http.Request) { fmt.Fprint(w, "Hello World") }
This code implements a simple HTTP server, listening to the local 8080 port. When the client initiates an HTTP request, the "indexHandler" function is called and "Hello World" is returned to the client.
- HTTP client
HTTP client is used to send requests to the server and process the server's response. In the Go language, you can use the "Get", "Post", "Put" and "Delete" methods in the "http" package to implement HTTP requests.
The sample code is as follows:
package main import ( "fmt" "io/ioutil" "net/http" ) func main() { resp, err := http.Get("http://example.com/") if err != nil { fmt.Println(err) return } defer resp.Body.Close() body, err := ioutil.ReadAll(resp.Body) if err != nil { fmt.Println(err) return } fmt.Println(string(body)) }
This code implements a simple HTTP client, sends an HTTP request to "http://example.com", and returns the response content.
3. HTTP request example
Let’s take a look at a complete HTTP request example. This instance implements the processing of HTTP requests, including the processing of GET and POST methods.
package main import ( "fmt" "io/ioutil" "net/http" ) func main() { http.HandleFunc("/", indexHandler) http.ListenAndServe(":8080", nil) } func indexHandler(w http.ResponseWriter, r *http.Request) { if r.Method == "GET" { getHandler(w, r) } else if r.Method == "POST" { postHandler(w, r) } else { http.Error(w, "Method Not Allowed", http.StatusMethodNotAllowed) } } func getHandler(w http.ResponseWriter, r *http.Request) { fmt.Fprint(w, "GET Request") } func postHandler(w http.ResponseWriter, r *http.Request) { body, err := ioutil.ReadAll(r.Body) if err != nil { http.Error(w, err.Error(), http.StatusInternalServerError) return } fmt.Fprintf(w, "POST Request: %v", string(body)) }
This code implements a simple HTTP server that listens to the local port 8080. When the client initiates an HTTP request, if it is a GET method, it will call the "getHandler" function and return "GET Request" to the client; if it is a POST method, it will call the "postHandler" function and return "POST Request" and the request body to client.
Summary
This article introduces how to use Go language to implement HTTP request processing. Through the "net/http" package in the standard library of the Go language, HTTP servers and clients can be easily implemented, and HTTP methods such as GET, POST, PUT, and DELETE are supported. If you are looking for efficient HTTP processing, try using Go language.
The above is the detailed content of golang receives request. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
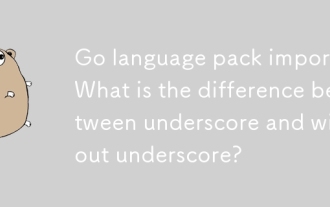
This article explains Go's package import mechanisms: named imports (e.g., import "fmt") and blank imports (e.g., import _ "fmt"). Named imports make package contents accessible, while blank imports only execute t

This article explains Beego's NewFlash() function for inter-page data transfer in web applications. It focuses on using NewFlash() to display temporary messages (success, error, warning) between controllers, leveraging the session mechanism. Limita
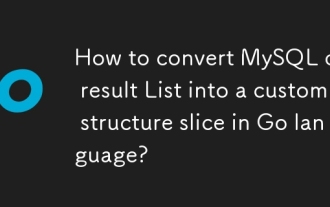
This article details efficient conversion of MySQL query results into Go struct slices. It emphasizes using database/sql's Scan method for optimal performance, avoiding manual parsing. Best practices for struct field mapping using db tags and robus
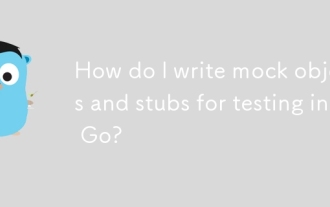
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
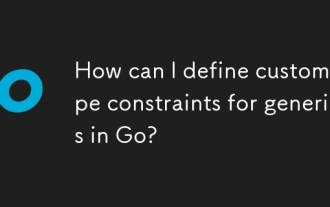
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
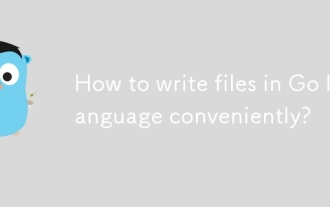
This article details efficient file writing in Go, comparing os.WriteFile (suitable for small files) with os.OpenFile and buffered writes (optimal for large files). It emphasizes robust error handling, using defer, and checking for specific errors.
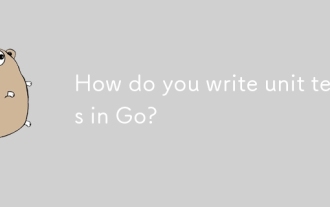
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
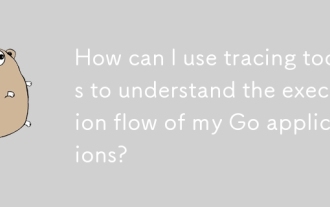
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
