golang division decimals
Golang is an open source programming language. Its original design is to improve the readability and maintainability of programs while maintaining efficiency. It supports object-oriented programming and functional programming paradigms, and provides powerful library and tool support. Among them, mathematical operations are one of the basic functions in programming languages, and division operations are also a very important operation in Golang.
However, you may encounter decimal precision issues when performing division operations in Golang. For example, if we wish to calculate the value of 9/4, we would usually expect to get the result 2.25. But in Golang, when we use the /
operator to calculate 9/4, we get 2, which is an integer, not the expected decimal.
The root of this problem lies in the difference between integer division and floating point division in Golang. In Golang, integer division only retains the integer part and discards the decimal part; while floating-point division will retain the decimal part. When we calculate one integer divided by another integer, Golang will perform integer division by default. If we want the result of a calculation to contain a decimal part, we need to convert the divisor or dividend to a floating point number.
One way to solve this problem is to convert the divisor or dividend to a floating point number. In Golang, we can convert an integer to a floating point number and then divide the floating point number by another integer to get a result with higher decimal precision. The sample code is as follows:
a := 9 b := 4 result := float64(a) / float64(b) fmt.Println(result)
After running the above code, we will get the result of 2.25, which is the same as expected.
Another way to solve this problem is to use the math package provided by Golang. Many mathematical functions are provided in the math package, including floating point division functions. If we need to perform exact floating point division operations, we can use the related functions in the math package. The sample code is as follows:
a := 9.0 b := 4.0 result := a / b fmt.Println(result)
In the above code, we use floating-point divisors and dividends to obtain more accurate results by using floating-point division operations.
In addition to converting integers to floating point numbers or using the math package for floating point operations, the strconv package in Golang also provides a way to solve the problem of decimal precision. This package provides a series of functions for converting strings to numbers, including functions for converting strings to floating point numbers. We can convert both the dividend and the divisor to floating-point types, and use Atoi()
to convert the floating-point calculation results into string format for output. The sample code is as follows:
a := "9" b := "4" aFloat, _ := strconv.ParseFloat(a, 64) bFloat, _ := strconv.ParseFloat(b, 64) result := aFloat / bFloat fmt.Println(strconv.FormatFloat(result, 'f', 2, 64))
In the above code, we use the strconv.ParseFloat()
function to convert the string into a floating point number, and use strconv.FormatFloat()
The function converts the floating-point calculation result into a string and outputs it. Among them, the first parameter indicates the precision of the number, and the second parameter indicates which rounding method is used.
Generally speaking, Golang’s division operation requires developers to pay attention when dealing with decimal precision issues. It is necessary to choose appropriate solutions according to different scenarios to ensure the correctness and accuracy of the program. At the same time, when performing data calculation operations, reasonable handling of decimal precision issues is also an important means to optimize program performance.
The above is the detailed content of golang division decimals. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
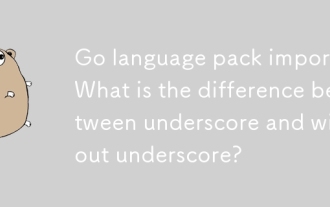
This article explains Go's package import mechanisms: named imports (e.g., import "fmt") and blank imports (e.g., import _ "fmt"). Named imports make package contents accessible, while blank imports only execute t
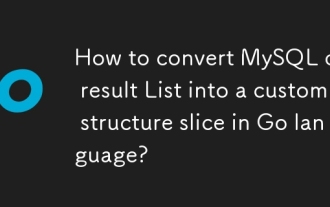
This article details efficient conversion of MySQL query results into Go struct slices. It emphasizes using database/sql's Scan method for optimal performance, avoiding manual parsing. Best practices for struct field mapping using db tags and robus

This article explains Beego's NewFlash() function for inter-page data transfer in web applications. It focuses on using NewFlash() to display temporary messages (success, error, warning) between controllers, leveraging the session mechanism. Limita
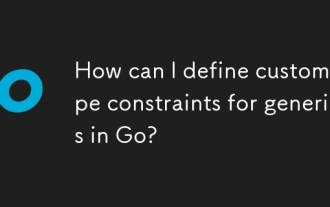
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
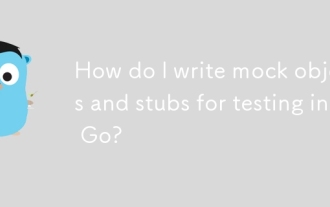
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
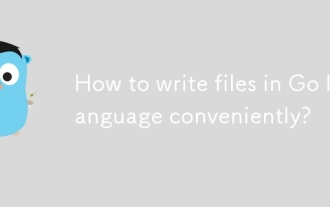
This article details efficient file writing in Go, comparing os.WriteFile (suitable for small files) with os.OpenFile and buffered writes (optimal for large files). It emphasizes robust error handling, using defer, and checking for specific errors.
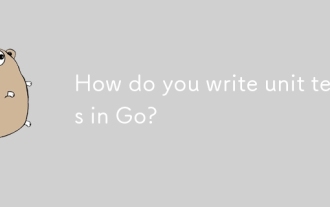
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
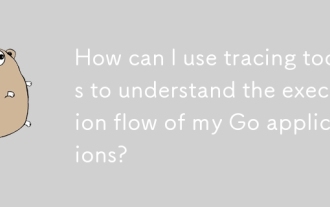
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
