php convert array into object array object
In PHP, we often use arrays and objects. Arrays can be used to conveniently store a set of related data, and objects can be used to store some attributes and behaviors. Sometimes we need to convert an array into an object, or an object into an associative array.
This article will introduce how to use PHP functions to convert an array into an object array, and how to convert an object into an associative array.
Convert an array into an object array
In PHP, we can use cast to convert an array into an object. Here is a simple example:
$arr = ['name' => 'Jack', 'age' => 18]; $obj = (object) $arr;
In this example, we define an associative array $arr
, and then use (object)
to convert it into An object $obj
. Now we can access the data in the array through the properties $name
and $age
of $obj
:
echo $obj->name; // Jack echo $obj->age; // 18
But if there are Multiple subarrays, we need to recursively process each subarray to convert them all into objects. Here is a recursive function to implement:
function arrayToObject($array) { if (!is_array($array)) { return $array; } $object = new stdClass(); if (is_array($array) && count($array) > 0) { foreach ($array as $name => $value) { $name = strtolower(trim($name)); if (!empty($name)) { $object->$name = arrayToObject($value); } } return $object; } else { return FALSE; } }
This function implements the function of converting an array into an object. It first checks whether the incoming parameter is an array, and if not, it returns directly; if it is, it creates a new object. Then for each element in the array, convert it into a lowercase property name, and call the arrayToObject
function recursively. Finally returns the converted object.
The following is an example of usage:
$data = [ 'name' => 'Jack', 'age' => 18, 'address' => [ 'state' => 'CA', 'city' => 'San Francisco' ] ]; $obj = arrayToObject($data); echo $obj->name; // Jack echo $obj->address->state; // CA
In this example, we define a multidimensional array $data
, which contains a subarray address
. Then use the arrayToObject
function to convert it into an object, and finally access the data through the properties of $obj
.
Convert an object into an associative array
The method of converting an object into an associative array is relatively simple. We can use the object's get_object_vars
method to convert it into an array. The following is an example:
class Person { public $name; public $age; public $address; public function __construct($name, $age, $address) { $this->name = $name; $this->age = $age; $this->address = $address; } } $person = new Person('Jack', 18, 'San Francisco'); $arr = get_object_vars($person); print_r($arr);
In this example, we define a Person
class, which contains three attributes: name
, age
and address
. Then a $person
object is created and converted into an array $arr
using get_object_vars
. Finally use print_r
to output the array.
The output results are as follows:
Array ( [name] => Jack [age] => 18 [address] => San Francisco )
Conclusion
In PHP, we can convert an array into an object through forced type conversion, or we can use get_object_vars
Method converts an object into an associative array. For multidimensional arrays and objects, we need recursive processing to achieve conversion. Understanding these techniques can help us better handle arrays and objects in PHP.
The above is the detailed content of php convert array into object array object. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
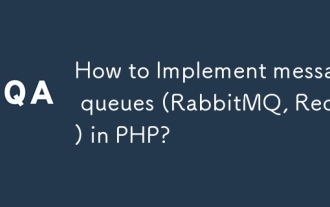
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
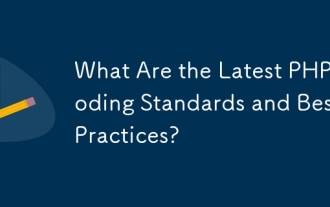
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
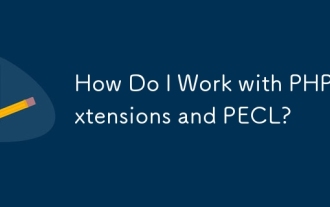
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
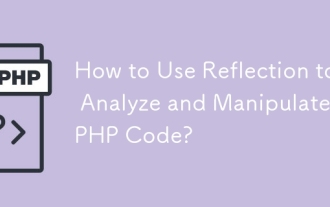
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
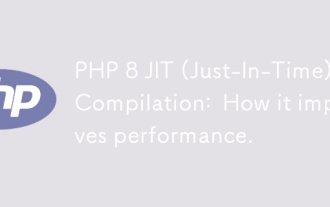
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
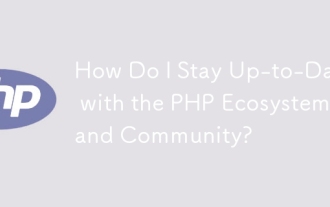
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
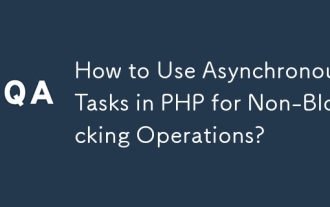
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
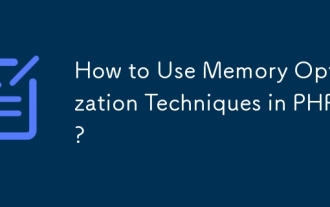
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v
