


How to write Python applications using Flask Blueprint and SQLAlchemy
Installation preparation
python3 -V && pip3 -V pip3 install pipenv
pipenv
pipenv shell
The environment configuration of PyCharm will not be explained too much here. Here is the follow-up Code is explained.
Start practicing
The principle of Flask is to bind the blueprint and the App to implement the Web routing function when the App is initialized. The implementation of routing is the first step in all projects.
Entry file
Before starting the project, define an entry file to allow requests to instantiate the App. What the entry file needs to do is initialize the configuration file, introduce the controller, initialize the database and other operations.
def create_app(): app = Flask(__name__) # 导入config中的配置文件 app.config.from_object('app.config.setting') app.config.from_object('app.config.secure') return app
Call it in the startup file. The judgment needs to be added to the startup file. The reason will be explained later.
from app.app import create_app app = create_app() if __name__ == '__main__': app.run(debug =True,port=81,host= '0.0.0.0')
Routing
Flask is a routing function implemented using blueprints. A method of registering blueprints is added to the entry file to implement introduction and registration.
from flask import Blueprint login = Blueprint('login', __name__) @login.route('/login') def loginIndex(): return "login Hello"
Introduce the blueprint module when the app instance is initialized
from flask import Flask def create_app(): app = Flask(__name__) # 导入config中的配置文件 app.config.from_object('app.config.setting') app.config.from_object('app.config.secure') # 注册并导入蓝图 register_blue(app) return app def register_blue(app): from app.api.login import login app.register_blueprint(login)
Optimize the router
You can add a loader to each router, and load Flask in sequence during initialization The blueprint has achieved the purpose of optimizing the router.
class BaseRoutes: def __init__(self, name): self.name = name self.loader = [] def route(self, rule, **options): def decorator(f): self.loader.append((f, rule, options)) return f return decorator def register(self, bp, url_prefix=''): # 依次注册蓝图 for f, rule, options in self.loader: endpoint = options.pop("endpoint", f.__name__) bp.add_url_rule(url_prefix + rule, endpoint, f, **options)
The optimized loader code used in the api file is as follows:
from app.libs.BaseRoutes import BaseRoutes api = BaseRoutes('login') @api.route('/login/loginHandle', methods=['GET', 'POST']) def loginHandle(): return "login Hello"
SQLAlchemy
After the routing of the web is completed, we begin to further add, delete, modify and check the database For practice and exploration, flask uses SQLAlchemy to operate the database. Here we take the Mysql database as an example.
Using SQLAlchemy requires installing two components of the database driver package. Installing the two components of Flask-SQLAlchemy and PyMySQL will make our development simpler and more convenient.
Definition of database class
For all Flask applications and plug-ins, they need to be registered in the App and use objects to operate. First define the base class for database operations and let other Modules are registered in Base.
Write public methods in all DBs into Base to reduce the process of reinventing the wheel.
instantiate SQLAlchemy in Base
from flask_sqlalchemy import SQLAlchemy db = SQLAlchemy() class Base(db.Model): # 忽略基类的主键 __abstract__ = True
Ordinary data classes inherit the Base class, take the User class as an example, introduce the db package before use
from app.models.base import Base class User(Base): __tablename__ = 'user' id = Column(Integer, primary_key=True, autoincrement=True) name = Column(String(50), nullable=False, default="") email = Column(String(120), nullable=False, default="") _password = Column('password',String(64)) @property def password(self): return self._password @password.setter def password(self, raw): self._password = generate_password_hash(raw)
Entry file loading DB
When loading data, you need to load the database initialization configuration, which is specified using SQLALCHEMY_DATABASE_URI
and has a specified format.
SQLALCHEMY_DATABASE_URI = 'mysql+pymysql://root:123456@127.0.0.1:3306/admin?charset=utf8'
SQLALCHEMY_DATABASE_URI =
Database driver name://username:password@ip address:port number/database name
def create_app(): app = Flask(__name__) app.config.from_object('app.config.secure') # 初始化数据库 db.init_app(app) with app.app_context(): db.create_all() return app
Describe and explain the role of the with keyword , in Python, the stack data structure is mainly used to run App instances. The with keyword can distinguish context very well. When resources are disconnected, they will be automatically released and recycled, which can optimize the program.
Mysql driver can use cymysql or pymysql. There are more tutorials on using pymysql on the Internet. When using the driver, if you are undecided, go to github and use a plug-in with a large number of stat, and choose the popular one. , so there will be more solutions.
Methods defined in the module
Model can define models, constants, atomic methods that directly operate the database, and you can also use the form of db.session
to read data.
from sqlalchemy import Column, Integer, String, SmallInteger from app.models.base import Base, db class tp_manager(Base): STATUS_NORMAL = 1 STATUS_STOP = 0 # ... @classmethod def get_manager_by_name(cls, username): r = cls.query.filter(cls.username == username, cls.status == cls.STATUS_NORMAL).one() return r @classmethod def get_db(cls): r = db.session.query(cls).filter(cls.status == cls.STATUS_NORMAL).all() return r
Use in routing
When used in routing, you need to introduce the corresponding model package in models. The following is a simple demo. User permissions can use the falsk-login component. to store user information.
from flask import request, session, jsonify from app.libs.BaseRoutes import BaseRoutes from app.validators.form.login import LoginForm from app.models.admin.tp_manager import tp_manager api = BaseRoutes('login') @api.route('/login/loginHandle', methods=['POST']) def loginHandle(): form = LoginForm(request.form) if form.validate(): # 验证通过的逻辑 r = tp_manager.get_manager_by_name(form.username.data) if r: msg = '操作成功' session['manager_id'] = r.id else: msg = '账号和密码错误' return jsonify({"code": 200, "data": [], "msg": msg}) else: # 错误暂时忽略... return form.errors
By the way, before flask uses session, it needs to configure SECRET_KEY
in the configuration file, otherwise an error will be reported, and the key value can be customized.
SECRET_KEY = '需要加密的key值'
SQLAlchemy implements mysql encoding and column type
After SQLAlchemy implemented basic operations on Mysql, I found that the default value set did not take effect, and the character set encoding was also set to the default latin1
, optimizing the column type of Mysql, implementing Mysql connection pool, and accessing NoSql databases such as mongo and redis have become issues to be studied in the next step.
Default value and index setting
The explanation in the python source code package is very clear and comes with examples. In Column
it is set like this:
Column(Integer, index=True, nullable=False, server_default="0",comment="昵称")
server_default
Orm sets the value of initializing Mysql, unique specifies whether it is the only index, default
is the default value when SQLAlchemy performs CURD operations, server_defaul
and The value of default
must be of string type.
index
is to set the index, nullable
is to set whether it is empty, and comment
is to set the comment information.
Compatible with Mysql column type
But there is a question before us. If you want to use the tinyint type, how to set the character set?
from sqlalchemy.dialects.mysql import VARCHAR, TEXT, BIGINT, INTEGER, SMALLINT, TINYINT, DECIMAL, FLOAT, \ DOUBLE, DATETIME, TIMESTAMP, DECIMAL
Take the most commonly used int and varchar as an example. Before using, you must import the corresponding package:
from sqlalchemy import Column, Index, Integer from sqlalchemy.dialects.mysql import VARCHAR, TEXT, BIGINT, INTEGER, SMALLINT, TINYINT, DECIMAL, FLOAT, \ DOUBLE, DATETIME, TIMESTAMP, DECIMAL from app.models.base import Base, db class wm_user_user(Base): STATUS_NORMAL = 1 __tablename__ = 'wm_user_user' user_status = Column(TINYINT(display_width=1,unsigned=True), nullable=False, server_default="1", comment="1为正常,0为审核中") user_nickname = Column(VARCHAR(length=50), index=True, nullable=False, comment="昵称") # 添加配置设置编码 __table_args__ = { 'mysql_charset': 'utf8mb4', 'mysql_collate': 'utf8mb4_general_ci' }
There are three parameters in the TINYINT type:
display_width sets the column Type width, after setting it will be displayed tinyint(1)
, the second unsigned is the value range of positive values, the third zerofill is filled, the value is a numeric type, the following is TINYINT Source code usage instructions.
"""Construct a TINYINT. :param display_width: Optional, maximum display width for this number. :param unsigned: a boolean, optional. :param zerofill: Optional. If true, values will be stored as strings left-padded with zeros. Note that this does not effect the values returned by the underlying database API, which continue to be numeric. """
Let me briefly introduce the string type with varchar as the code. The string type must explicitly declare the string length, which is implemented by length. If the value of length is not added, an error will occur during initialization.
自定义数据库名和字符集编码
__tablename__
设置的是自定义数据表名,底下的设置的是数据表字符集编码,要使用utf8mb4编码和utf8mb4_general_ci
编码,这里就不做过多的解释了。
__tablename__ = 'wm_user_user' # ... __table_args__ = { 'mysql_charset': 'utf8mb4', 'mysql_collate': 'utf8mb4_general_ci' }
The above is the detailed content of How to write Python applications using Flask Blueprint and SQLAlchemy. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
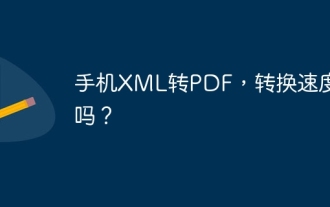
The speed of mobile XML to PDF depends on the following factors: the complexity of XML structure. Mobile hardware configuration conversion method (library, algorithm) code quality optimization methods (select efficient libraries, optimize algorithms, cache data, and utilize multi-threading). Overall, there is no absolute answer and it needs to be optimized according to the specific situation.
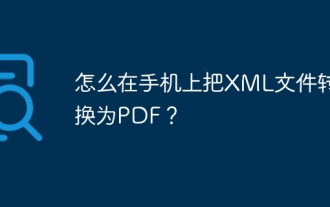
It is impossible to complete XML to PDF conversion directly on your phone with a single application. It is necessary to use cloud services, which can be achieved through two steps: 1. Convert XML to PDF in the cloud, 2. Access or download the converted PDF file on the mobile phone.
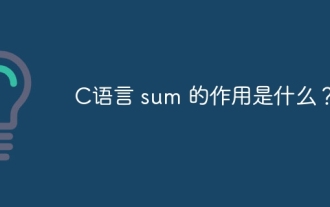
There is no built-in sum function in C language, so it needs to be written by yourself. Sum can be achieved by traversing the array and accumulating elements: Loop version: Sum is calculated using for loop and array length. Pointer version: Use pointers to point to array elements, and efficient summing is achieved through self-increment pointers. Dynamically allocate array version: Dynamically allocate arrays and manage memory yourself, ensuring that allocated memory is freed to prevent memory leaks.
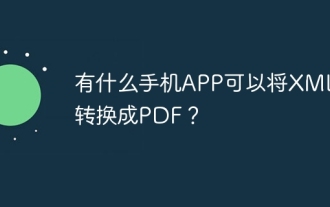
An application that converts XML directly to PDF cannot be found because they are two fundamentally different formats. XML is used to store data, while PDF is used to display documents. To complete the transformation, you can use programming languages and libraries such as Python and ReportLab to parse XML data and generate PDF documents.
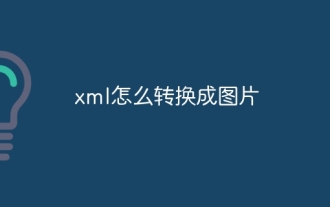
XML can be converted to images by using an XSLT converter or image library. XSLT Converter: Use an XSLT processor and stylesheet to convert XML to images. Image Library: Use libraries such as PIL or ImageMagick to create images from XML data, such as drawing shapes and text.
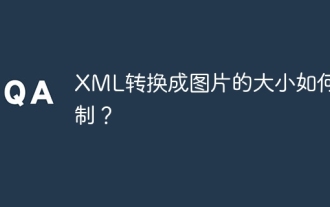
To generate images through XML, you need to use graph libraries (such as Pillow and JFreeChart) as bridges to generate images based on metadata (size, color) in XML. The key to controlling the size of the image is to adjust the values of the <width> and <height> tags in XML. However, in practical applications, the complexity of XML structure, the fineness of graph drawing, the speed of image generation and memory consumption, and the selection of image formats all have an impact on the generated image size. Therefore, it is necessary to have a deep understanding of XML structure, proficient in the graphics library, and consider factors such as optimization algorithms and image format selection.
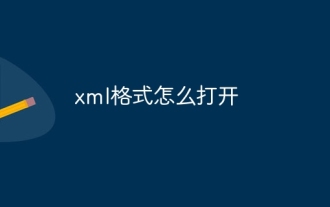
Use most text editors to open XML files; if you need a more intuitive tree display, you can use an XML editor, such as Oxygen XML Editor or XMLSpy; if you process XML data in a program, you need to use a programming language (such as Python) and XML libraries (such as xml.etree.ElementTree) to parse.
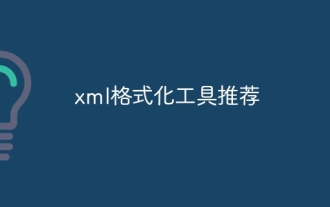
XML formatting tools can type code according to rules to improve readability and understanding. When selecting a tool, pay attention to customization capabilities, handling of special circumstances, performance and ease of use. Commonly used tool types include online tools, IDE plug-ins, and command-line tools.
