How to traverse the same array in php
In PHP, traversing an array is a very common operation. We usually want to use this method to find elements in an array, operate on an array, or output all elements in an array. In some cases, we need to find those elements with the same value to perform some other operations. This article will explore in detail how to traverse the same array in PHP.
First look at the two methods of traversing arrays in PHP:
- foreach loop
The foreach loop is the most common method of traversing arrays in PHP , its basic syntax structure is as follows:
foreach (array_expression as $key => $value) { // code to be executed; }
-
array_expression
: the array to be traversed -
$key
: the key of the current element -
$value
: The value of the current element
The foreach loop will traverse each element in the array in turn and assign its key and value to $key
and $value
, and then execute the corresponding code.
As a simple example, we have an array:
$arr = array(2, 4, 6, 8, 10);
Use a foreach loop to traverse this array and output each element:
foreach ($arr as $value) { echo $value . "<br>"; }
The output is as follows:
2 4 6 8 10
- for loop
The for loop is also a common way to traverse an array in PHP, similar to the usual for loop. The basic syntax structure is as follows:
for ($i = 0; $i < count($array); $i++) { // code to be executed; }
-
$array
: the array to be traversed -
$i
: the index of the current element
The for loop will use the count()
function to count the number of elements in the array and store it in the variable $i
, and then iterate through each element in the array. elements.
As a simple example, we have the same array as above:
$arr = array(2, 4, 6, 8, 10);
Use a for loop to traverse this array and output each element:
for ($i = 0; $i < count($arr); $i++) { echo $arr[$i] . "<br>"; }
The output is as follows:
2 4 6 8 10
The above are the basic methods of traversing arrays in PHP. Below we will introduce how to traverse the same array.
- Use the array_count_values() function
The array_count_values() function can find elements with the same value in an array. Its basic syntax structure is as follows:
array array_count_values ( array $array )
-
$array
: the array to be found
This function will return a new array containing The number of occurrences of each element in the original array. To give a simple example, we have an array:
$arr = array(1, 2, 3, 4, 3, 2, 1, 5);
Use the array_count_values() function to find the same elements:
$newArr = array_count_values($arr); print_r($newArr);
The output is as follows:
Array ( [1] => 2 [2] => 2 [3] => 2 [4] => 1 [5] => 1 )
You can see from the output Out, the new array contains the number of occurrences of each element in the original array, of which elements 1, 2, and 3 appear twice, and elements 4 and 5 only appear once.
- Using a foreach loop
Another way to iterate through the same elements in an array is to use a foreach loop. The specific idea is to use a nested foreach loop. Each time it traverses an array element, it traverses the elements behind it and compares whether their values are the same. To give a simple example, we have an array:
$arr = array(1, 2, 3, 4, 3, 2, 1, 5);
Use a foreach loop to traverse this array and find the same elements:
$sameArr = array(); foreach ($arr as $key1 => $value1) { foreach ($arr as $key2 => $value2) { if ($key1 < $key2 && $value1 == $value2) { $sameArr[] = $value1; } } } print_r($sameArr);
The output is as follows:
Array ( [0] => 2 [1] => 3 [2] => 1 )
From As can be seen from the output, the same elements 2, 3, and 1 in the original array were found. In fact, this method is more troublesome and more time-consuming when processing large arrays. It is recommended to use the array_count_values() function.
The above are two methods of traversing the same array in PHP. I hope it will be helpful to you.
The above is the detailed content of How to traverse the same array in php. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
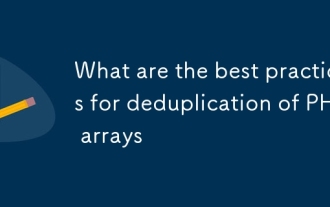
This article explores efficient PHP array deduplication. It compares built-in functions like array_unique() with custom hashmap approaches, highlighting performance trade-offs based on array size and data type. The optimal method depends on profili
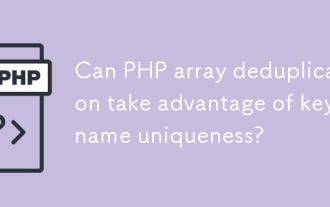
This article explores PHP array deduplication using key uniqueness. While not a direct duplicate removal method, leveraging key uniqueness allows for creating a new array with unique values by mapping values to keys, overwriting duplicates. This ap
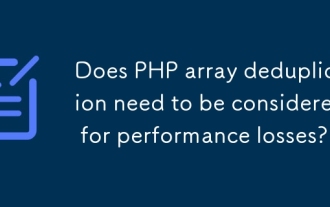
This article analyzes PHP array deduplication, highlighting performance bottlenecks of naive approaches (O(n²)). It explores efficient alternatives using array_unique() with custom functions, SplObjectStorage, and HashSet implementations, achieving
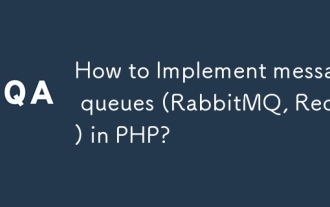
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
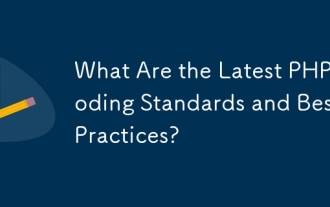
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
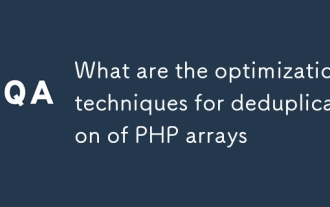
This article explores optimizing PHP array deduplication for large datasets. It examines techniques like array_unique(), array_flip(), SplObjectStorage, and pre-sorting, comparing their efficiency. For massive datasets, it suggests chunking, datab
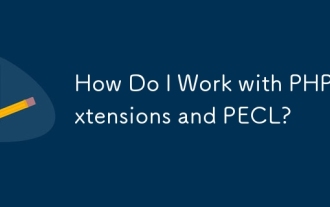
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
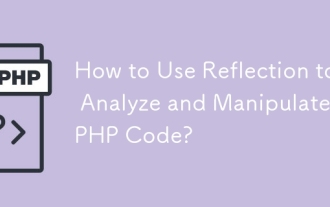
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
