How to loop out php array
In PHP programming, array is a very useful data type. In some cases, it is necessary to loop or traverse the data stored in the array. In this article, we will explore how to loop over elements in a PHP array.
1. Basic for loop
The most basic way is to use a for loop to access the elements in the array by specifying the subscript of the loop variable. The code example is as follows:
$myArray = array('apple', 'banana', 'cherry', 'date'); for ($i = 0; $i < count($myArray); ++$i) { echo $myArray[$i] . ', '; }
Output result:
apple, banana, cherry, date,
In the above code, we first create an array $myArray
containing four string elements. Then we use the for
loop, and the loop variable $i
starts from 0 until the length of the array, which is 3. In the loop body, we access the array elements through the subscript $i
and output them.
2. Foreach loop
Using the foreach
loop method can traverse the array more conveniently without caring about the length of the array, and has higher flexibility and concise syntax. sex. The following is a sample code:
$myArray = array('apple', 'banana', 'cherry', 'date'); foreach ($myArray as $item) { echo $item . ', '; }
Output result:
apple, banana, cherry, date,
In the above code, we use the foreach
loop, and the variable $item
will be automatically assigned is the value of the array element and then outputs it.
You can get the key name of the current element by passing the variable $key
to the foreach
loop. The sample code is as follows:
$myArray = array('apple', 'banana', 'cherry', 'date'); foreach ($myArray as $key => $item) { echo "索引为 $key 的元素是 $item , "; }
Output result:
索引为 0 的元素是 apple, 索引为 1 的元素是 banana, 索引为 2 的元素是 cherry, 索引为 3 的元素是 date ,
3. While loop
In addition to the above two methods, you can also use while
loop output PHP array. The following is a sample code:
$myArray = array('apple', 'banana', 'cherry', 'date'); $i = 0; while ($i < count($myArray)) { echo $myArray[$i] . ', '; ++$i; }
Output result:
apple, banana, cherry, date,
In the above code, we use the while
loop, and the initial value of the variable $i
is 0 , it will increase by 1 each time the loop is executed. In the loop body, we obtain the array elements for output through the subscript $i
.
4. Do-while loop
Similar to the while
loop, the do-while
loop can also be used to output PHP array elements. An example is as follows:
$myArray = array('apple', 'banana', 'cherry', 'date'); $i = 0; do { echo $myArray[$i] . ', '; ++$i; } while ($i < count($myArray));
Output result:
apple, banana, cherry, date,
It should be noted that the do-while
loop will execute the loop body at least once, even if the initial conditions do not meet the loop conditions .
Summary:
In this article, we introduced how to use different loop methods such as for, foreach, while, and do-while to loop out PHP array elements. The method you choose to loop through array elements depends on the application scenario and personal programming habits. No matter which method is used, you need to correctly use the array subscript or the variable of the foreach loop in the loop body to output the traversed elements.
The above is the detailed content of How to loop out php array. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
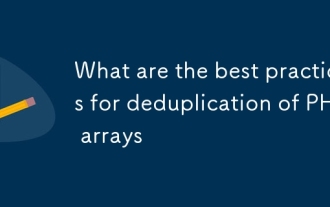
This article explores efficient PHP array deduplication. It compares built-in functions like array_unique() with custom hashmap approaches, highlighting performance trade-offs based on array size and data type. The optimal method depends on profili
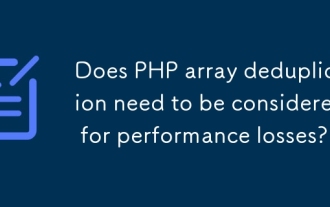
This article analyzes PHP array deduplication, highlighting performance bottlenecks of naive approaches (O(n²)). It explores efficient alternatives using array_unique() with custom functions, SplObjectStorage, and HashSet implementations, achieving
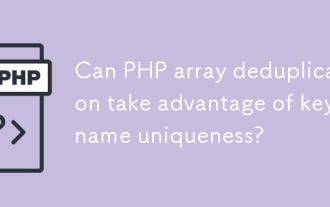
This article explores PHP array deduplication using key uniqueness. While not a direct duplicate removal method, leveraging key uniqueness allows for creating a new array with unique values by mapping values to keys, overwriting duplicates. This ap
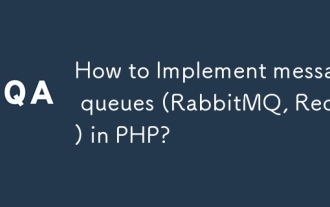
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
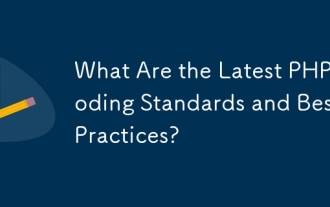
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
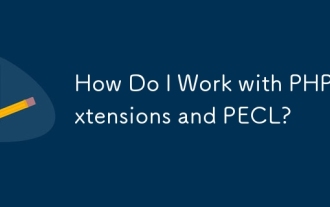
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
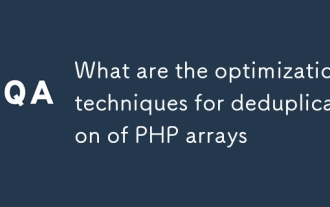
This article explores optimizing PHP array deduplication for large datasets. It examines techniques like array_unique(), array_flip(), SplObjectStorage, and pre-sorting, comparing their efficiency. For massive datasets, it suggests chunking, datab
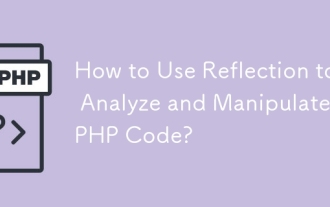
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
