nodejs implements web side
nodejs is a JavaScript runtime environment that runs on the server side. It allows developers to write server-side code in JavaScript to interact with the Web front-end.
Over the past few years, nodejs has become increasingly popular because it provides an efficient, scalable, and easy-to-deploy development environment. Numerous companies and organizations have adopted nodejs, such as LinkedIn, Walmart, and PayPal.
In this article, we will introduce how to use nodejs to implement web-side development, including the deployment of web applications, routing and middleware, and the use of template engines to render server-side data.
- Install nodejs
First, we need to install nodejs. We can find download links for different operating systems on the nodejs official website.
After the installation is completed, we can enter node -v in the terminal to verify whether the installation is successful. If successful, the nodejs version number will be displayed.
- Create a web application
Next, we can create a simple web application using nodejs. Open a terminal, go to an empty folder, and enter the following command:
npm init -y
This command will create a new package.json file that contains the metadata of our project.
Next, we need to install the express module, which is one of the most popular web application frameworks in nodejs. We can use the following command to install:
npm install express
After the installation is complete, we can create an index.js file, which will contain our nodejs code. In index.js, we will import the express module and create a new instance:
const express = require('express'); const app = express();
This means that our application can now use the methods and properties provided by express. Next, we need to tell the application what port to listen on to receive http requests.
const port = 3000; app.listen(port, () => { console.log(`Server started on port ${port}`); });
Here we tell the application to listen on port 3000. And use console.log() to print out a message so that we know the server has started.
- Add Routes
Now that we have the basic framework set up for our application, we need to start adding routes to navigate to different pages. In express, routes define how an application responds to client requests.
We can create a simple dynamic route using the following code:
app.get('/', (req, res) => { res.send('Hello, World!'); })
This route defines a GET request that will return the "Hello, World!" message when the user requests the root path. .
We can also use static routing to define static pages and files of the application.
app.use(express.static('public'));
Here, we tell the server to look for static files in the "public" folder. This includes HTML, CSS, JavaScript, images and other static resources.
- Middleware
Middleware is code that is executed before or after routing. It can be used to process and modify request and response objects, thereby providing more functionality to the application.
For example, we can add a logging middleware for all routes using the following code:
app.use((req, res, next) => { console.log(`${req.method} ${req.url}`); next(); });
Here, we define a middleware function called "logger" that logs HTTP method and URL, and pass the request and response objects to the next middleware.
- Template Engine
In most web applications, we need to dynamically generate HTML pages on the server side. To achieve this functionality, we can use a template engine.
The template engine allows us to render HTML from the server instead of rendering from the client. This usually means faster loading times and better search engine optimization (SEO).
In nodejs, there are many different template engines to choose from, such as Pug, Handlebars and EJS.
The following is an example of rendering variables using the EJS template engine:
app.set('view engine', 'ejs'); app.get('/', (req, res) => { const data = { title: 'Hello, World!', message: 'This is the home page.' }; res.render('index', data); });
Here, we first set the template engine to EJS. Then, we define a data object whose properties include title and message. Finally, we render the EJS template named "index" using the res.render() method and pass the data object to the template.
In our EJS template, we can access these variables using the following code:
<h1><%= title %></h1> <p><%= message %></p>
Here, we use the syntax of <%= %> to output JavaScript variables. When the template is rendered, these variables will be replaced with actual data.
- Deploying the Application
After we have completed the development of the web application, we can deploy it on the web server so that it can be accessed by anyone.
One popular method is to use a cloud computing service such as Amazon Web Services (AWS) or Microsoft Azure. These services provide virtual computer instances, containers, and application platforms that can be used to deploy and host web applications.
We can also use platforms such as Heroku as deployment targets. These platforms provide simple command line tools and deployment processes that can be used to deploy code to remote servers.
Summary
In this article, we introduced how to use nodejs to implement web-side development. We learned how to create a basic web application, add routing and middleware, and use a template engine to render server-side data. Additionally, we discussed how to deploy applications on a Web server so that they can be widely used.
The above is the detailed content of nodejs implements web side. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


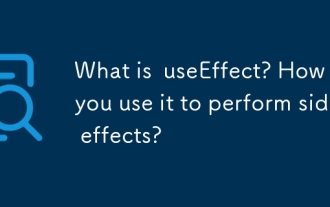
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.

Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.
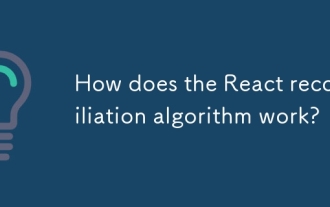
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
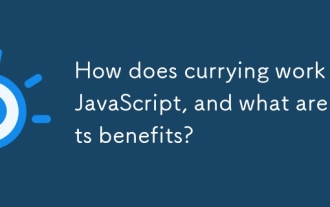
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read

Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
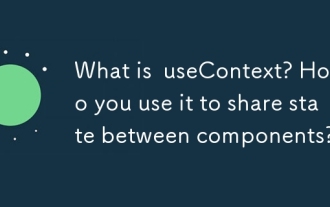
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
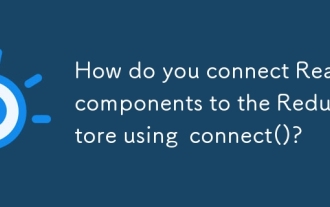
Article discusses connecting React components to Redux store using connect(), explaining mapStateToProps, mapDispatchToProps, and performance impacts.
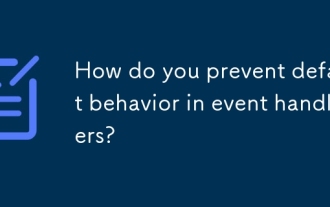
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
