nodejs http request tool
Node.js is a very popular development environment, and its powerful JavaScript engine can provide efficient web applications. In web development, HTTP requests and responses are often required, which requires the use of some HTTP request tools. This article will mainly introduce the commonly used HTTP request tools in Node.js.
1. Node.js built-in HTTP module
Node.js comes with its own HTTP module, which can easily create an HTTP service. In the HTTP module, many related request and response APIs are provided, involving reading of HTTP request headers and request bodies, output of response headers and response bodies, etc., which are very convenient to use. The following is a code that uses the HTTP module to create a server:
const http = require('http'); const server = http.createServer((req, res) => { res.statusCode = 200; res.setHeader('Content-Type', 'text/plain'); res.end('Hello World!'); }); server.listen(3000, () => { console.log('Server running at http://localhost:3000/'); });
2. Use the third-party module request
Although Node.js has a built-in HTTP module, its API may be too low-level. Use It's not very convenient to get up. Therefore, we can also choose to use third-party modules, such as the request module. First use npm to install:
npm install request
The request module provides a more convenient API that can quickly complete HTTP requests and obtain response data. The following is an example of using the request module to send a GET request:
const request = require('request'); request('http://www.baidu.com', function (error, response, body) { console.error('error:', error); // Print the error if one occurred console.log('statusCode:', response && response.statusCode); // Print the response status code if a response was received console.log('body:', body); // Print the HTML for the Google homepage. });
3. Using the third-party module axios
In addition to the request module, there is also a very powerful HTTP request tool - axios. It is a Promise-based HTTP client that can be used in the browser and Node.js. axios has the following features:
- Can intercept requests and responses.
- Automatically convert JSON data.
- Support cancellation request.
- You can set default request headers and request parameters.
- More reliable and lightweight.
Use npm to install:
npm install axios
The following is an example of using axios to send a GET request:
const axios = require('axios') axios.get('https://api.github.com/users/johnny4120') .then(function (response) { console.log(response.data) }) .catch(function (error) { console.log(error) })
4. Request parameter processing
When making a request, some parameters are often brought, and different modules handle them differently. When using the request module to make a request, you can use the querystring module to convert the object into a request parameter string, or you can use json parameters directly. For example:
const querystring = require('querystring'); const request = require('request'); const options = { url: 'https://www.google.com/search', qs: { q: 'node.js' } }; request(options, function(error, response, body) { console.log(body); }); // 或者 request.post({ url: 'http://www.example.com', json: {key: 'value'} }, function(error, response, body) { console.log(body); });
When using the axios module, you can use the params parameter to convert the object into a query string, or you can use the data parameter:
const axios = require('axios'); axios.get('https://api.github.com/search/repositories', { params: { q: 'node', sort: 'stars', order: 'desc' } }) .then(function (response) { console.log(response.data); }) .catch(function (error) { console.log(error); }); // 或者 axios.post('http://www.example.com', {foo: 'bar'}) .then(function (response) { console.log(response.data); }) .catch(function (error) { console.log(error); });
In summary, there are many methods in Node.js There are several HTTP request tools to choose from, each of which has its applicable scenarios. Choosing the most appropriate tool based on project needs will make development more efficient and convenient.
The above is the detailed content of nodejs http request tool. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



React combines JSX and HTML to improve user experience. 1) JSX embeds HTML to make development more intuitive. 2) The virtual DOM mechanism optimizes performance and reduces DOM operations. 3) Component-based management UI to improve maintainability. 4) State management and event processing enhance interactivity.
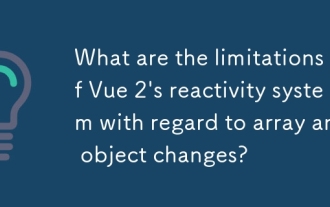
Vue 2's reactivity system struggles with direct array index setting, length modification, and object property addition/deletion. Developers can use Vue's mutation methods and Vue.set() to ensure reactivity.

React components can be defined by functions or classes, encapsulating UI logic and accepting input data through props. 1) Define components: Use functions or classes to return React elements. 2) Rendering component: React calls render method or executes function component. 3) Multiplexing components: pass data through props to build a complex UI. The lifecycle approach of components allows logic to be executed at different stages, improving development efficiency and code maintainability.
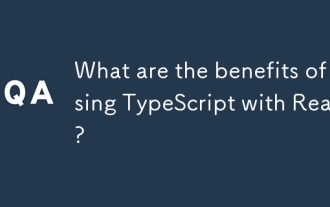
TypeScript enhances React development by providing type safety, improving code quality, and offering better IDE support, thus reducing errors and improving maintainability.

React is the preferred tool for building interactive front-end experiences. 1) React simplifies UI development through componentization and virtual DOM. 2) Components are divided into function components and class components. Function components are simpler and class components provide more life cycle methods. 3) The working principle of React relies on virtual DOM and reconciliation algorithm to improve performance. 4) State management uses useState or this.state, and life cycle methods such as componentDidMount are used for specific logic. 5) Basic usage includes creating components and managing state, and advanced usage involves custom hooks and performance optimization. 6) Common errors include improper status updates and performance issues, debugging skills include using ReactDevTools and Excellent
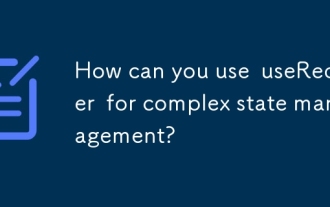
The article explains using useReducer for complex state management in React, detailing its benefits over useState and how to integrate it with useEffect for side effects.
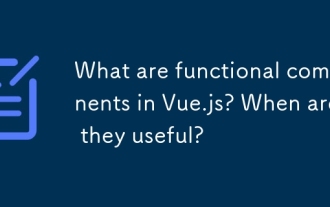
Functional components in Vue.js are stateless, lightweight, and lack lifecycle hooks, ideal for rendering pure data and optimizing performance. They differ from stateful components by not having state or reactivity, using render functions directly, a
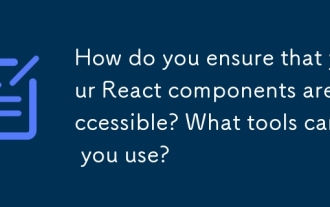
The article discusses strategies and tools for ensuring React components are accessible, focusing on semantic HTML, ARIA attributes, keyboard navigation, and color contrast. It recommends using tools like eslint-plugin-jsx-a11y and axe-core for testi
